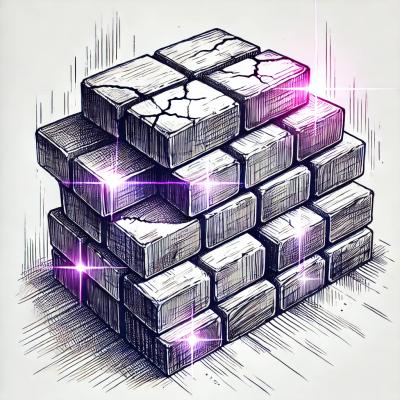
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Japa is a batteries included minimal testing framework for Node.Js. Japa does not have any cli to run your tests, infact running the test file as a node script will execute the tests for you (quite similar to tape)
Japa is a batteries included minimal testing framework for Node.Js. Japa does not have any cli to run your tests, infact running the test file as a node script will execute the tests for you (quite similar to tape).
Japa needs Node.js >= 6.0.0.
npm i --save japa
Below is the list of specific reasons to choose Japa over any other testing framework.
Japa core is so minimal and only focuses on building your tests, not running them.
Japa harness the goodness of ES2105 and later. You can define your tests as async methods or return a Promise from your tests.
It comes with fully-fledged API, which can be used to build your own test runners.
The default test reporter output is as pretty as Mocha spec reporter. Check below screenshot.
Majority of test runners outputs assertions diff quite similar to GIT. Which is great when diffing code, but not when reading failing test reports. Japa has a simple and clean diff viewer.
Japa minimal core does not limit the functionalities you get with it. It covers all the use cases of writing tests and offers explicit API's for them.
You can write your tests as async functions, return a Promise or make use of traditional done style callbacks
test('Async test', async (assert) => {
const result = await someTimeConsumingWork()
})
test('Returns promise', (assert) => {
return new Promise(() => {
})
})
test('Traditional callbacks', (assert, done) => {
fs.readFile(path, (error, contents) => {
if(error) {
return done(error)
}
assert.equal(contents, 'All good')
done()
})
})
At times your test passes, since the failing code is not reachable by your test files. It is always a good idea to plan assertions to these kind of scanerios.
const test = require('japa')
test('Planning assertions', (assert) => {
assert.plan(2)
const users = await User.all()
assert.isArray(users)
assert.deepEqual(users[0], { username: '...' })
})
You can define timeout for each test when defining them.
const test = require('japa')
test('This is the slow one', (assert) => {
}).timeout(5000)
We all deal with flaky tests, which needs to be retried couple of times before they can pass.
The below test will run for 4 times (3 retries + 1 original run cycle) before failing.
const test = require('japa')
test('I am so flaky', (assert) => {
}).retry(3)
Got a bug that needs some love? Skip it's tests until it get's fixed.
const test = require('japa')
test.skip('I will be skipped', (assert) => {
})
When bugs spotted, your users can create a PR with failing test instead of creating issues. Tests started with failing
will not break the test suite.
const test = require('japa')
test.failing('Some regression here', (assert) => {
assert.fail(2, 4, 'Expected 2 to be equal to 2 but got 4')
})
It is a good practice to keep your tests flat and not be depedented on any global state. But at times you may want to execute cleanup/setup methods before and after tests.
It is a good idea to group tests and perform clean tasks inside life cycle hooks.
Will run before all the tests in the group.
const test = require('japa')
test.group('Some Module', (group) => {
group.before(async () => {
})
})
Will run before each test in the group.
const test = require('japa')
test.group('Some Module', (group) => {
group.beforeEach(async () => {
})
})
Will run after all the tests in the group.
const test = require('japa')
test.group('Some Module', (group) => {
group.after(async () => {
})
})
Will run after each test in the group.
const test = require('japa')
test.group('Some Module', (group) => {
group.afterEach(async () => {
})
})
Below is the list of settings/options which can turn on/off globally.
Test suite doesn't stop on test failures and continue to run. The bail
method can be used to stop executing the test after first failure.
const test = require('japa')
test.bail(true)
test('title', () => {
})
Instead of defining timeout for each test, you can define them once for all tests.
const test = require('japa')
test.timeout(5000)
test('title', () => {
})
Japa makes use of the list
reporter bundled with the core. You can attach your own reporter by making use of the use
method.
const test = require('japa')
test.use(function (emitter) {
emitter.on('test:end', console.log)
})
test('title', () => {
})
Japa makes use of ChaiJs Assertion Library. Make sure to read their docs.
Here is the list of all the testing methods
Write a new test
test('title', (assert) => {})
Create a test to be skipped
test.skip('title', (assert) => {})
Create a test which is expected to be failed
test.failing('title', (assert) => {})
How long to wait for test to finish.
test('title', (assert) => {
}).timeout(5000)
How many times to retry a test before marking it as fail. The retry
only happens when test keeps on failing
test('title', (assert) => {
}).retry(2)
Create a new test group/suite. Nested groups are not allowed.
test.group('Module name', (group) => {
group.after(async () => >{
await Db.clear()
})
})
Below is the list of events, you can listen for when writing for your reporters.
{
title: 'GROUP TITLE',
status: 'pending'
}
{
title: 'GROUP TITLE',
status: 'failed | passed',
errors: [Array of errors for all failing tests]
}
{
title: 'TEST TITLE',
status: 'todo | skipped',
regression: 'true | false'
}
{
title: 'TEST TITLE',
status: 'todo | skipped | failed | passed',
timeout: 'true | false',
error: 'Error if failed',
regression: 'true | false',
regressionMessage: 'Error message of regression test (if regression)'
}
FAQs
Lean test runner for Node.js
We found that japa demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.