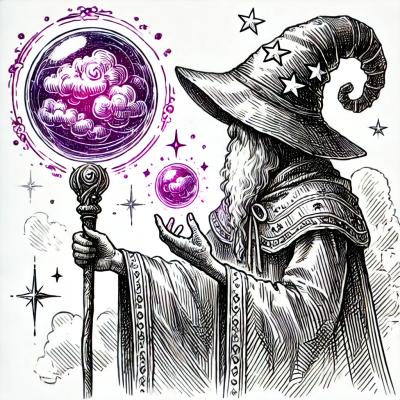
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
jasminewd2
Advanced tools
The jasminewd2 package is a bridge between Jasmine and WebDriverJS, allowing for seamless integration of asynchronous WebDriverJS commands in Jasmine tests. It provides utilities to handle promises and asynchronous operations in a more readable and manageable way.
Asynchronous Testing
This feature allows you to write tests that wait for promises to resolve before making assertions. The code sample demonstrates how to navigate to a URL and then check the page title, ensuring that the test waits for the navigation to complete.
describe('Asynchronous specs', function() {
it('should wait for the promise to resolve', function() {
return browser.get('http://www.example.com').then(function() {
expect(browser.getTitle()).toEqual('Example Domain');
});
});
});
Custom Matchers
This feature allows you to define custom matchers to extend Jasmine's built-in matchers. The code sample shows how to create a custom matcher `toBeWithinRange` that checks if a number falls within a specified range.
describe('Custom matchers', function() {
beforeEach(function() {
jasmine.addMatchers({
toBeWithinRange: function() {
return {
compare: function(actual, expected) {
var result = {};
result.pass = actual >= expected[0] && actual <= expected[1];
if (result.pass) {
result.message = 'Expected ' + actual + ' to be within range ' + expected;
} else {
result.message = 'Expected ' + actual + ' to be within range ' + expected + ' but it was not';
}
return result;
}
};
}
});
});
it('is within range', function() {
expect(100).toBeWithinRange([90, 110]);
});
});
Handling Asynchronous Setup and Teardown
This feature allows you to perform asynchronous operations in setup and teardown functions. The code sample demonstrates how to navigate to a URL before all tests and quit the browser after all tests, ensuring that these operations complete before proceeding.
describe('Asynchronous setup and teardown', function() {
beforeAll(function(done) {
browser.get('http://www.example.com').then(function() {
done();
});
});
afterAll(function(done) {
browser.quit().then(function() {
done();
});
});
it('should have a title', function() {
expect(browser.getTitle()).toEqual('Example Domain');
});
});
Protractor is an end-to-end test framework for Angular and AngularJS applications. It is built on top of WebDriverJS and integrates seamlessly with Jasmine, providing additional features like automatic waiting and Angular-specific locators. Compared to jasminewd2, Protractor offers a more comprehensive solution for testing Angular applications.
WebdriverIO is a popular WebDriver binding for Node.js. It supports various test frameworks, including Jasmine, Mocha, and Cucumber. WebdriverIO provides a rich set of features for writing and managing end-to-end tests, including support for asynchronous operations and custom commands. Compared to jasminewd2, WebdriverIO offers more flexibility in terms of test framework integration and additional utilities.
Nightwatch.js is an end-to-end testing framework built on top of Selenium WebDriver. It provides a simple syntax for writing tests and includes built-in support for running tests in parallel. Nightwatch.js also supports various test frameworks, including Mocha and Cucumber. Compared to jasminewd2, Nightwatch.js offers a more integrated solution with built-in test runner and assertion library.
Adapter for Jasmine-to-WebDriverJS. Used by Protractor.
Important: There are two active branches of jasminewd.
jasminewd
.jasminewd2
.Automatically makes tests asynchronously wait until the WebDriverJS control flow is empty.
If a done
function is passed to the test, waits for both the control flow and until done is called.
Enhances expect
so that it automatically unwraps promises before performing the assertion.
npm install jasminewd2
Assumes selenium-webdriver as a peer dependency.
// In your setup.
var JasmineRunner = require('jasmine');
var jrunner = new JasmineRunner();
require('jasminewd2');
global.driver = new webdriver.Builder().
usingServer('http://localhost:4444/wd/hub').
withCapabilities({browserName: 'chrome'}).
build();
jrunner.projectBaseDir = '';
jrunner.execute(['**/*_spec.js']);
// In your tests
describe('tests with webdriver', function() {
it('will wait until webdriver is done', function() {
// This will be an asynchronous test. It will finish once webdriver has
// loaded the page, found the element, and gotten its text.
driver.get('http://www.example.com');
var myElement = driver.findElement(webdriver.By.id('hello'));
// Here, expect understands that myElement.getText() is a promise,
// and resolves it before asserting.
expect(myElement.getText()).toEqual('hello world');
});
})
0.1.0-beta.0
This beta release is for the selenium-webdriver 3.0.0-beta-3 upgrade.
FAQs
WebDriverJS adapter for Jasmine2.
The npm package jasminewd2 receives a total of 506,908 weekly downloads. As such, jasminewd2 popularity was classified as popular.
We found that jasminewd2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.