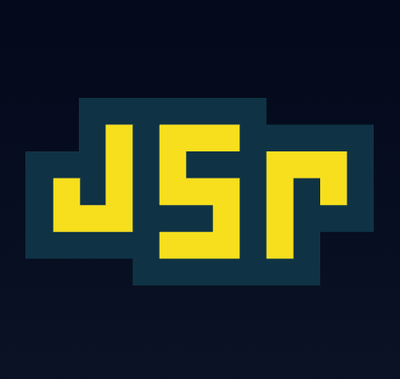
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
javascript-time-ago
Advanced tools
The `javascript-time-ago` npm package is used to format dates and times in a human-readable way, such as '5 minutes ago' or '2 days ago'. It supports multiple languages and locales, making it a versatile tool for internationalization.
Basic Usage
This feature allows you to format a date into a human-readable string indicating the time elapsed since that date. The example shows how to set up the package for English and format a date to '1 minute ago'.
const TimeAgo = require('javascript-time-ago');
const en = require('javascript-time-ago/locale/en');
TimeAgo.addLocale(en);
const timeAgo = new TimeAgo('en-US');
console.log(timeAgo.format(new Date(Date.now() - 60 * 1000))); // '1 minute ago'
Custom Styles
This feature allows you to define custom styles for formatting the elapsed time. The example demonstrates how to create a custom style and use it to format a date.
const TimeAgo = require('javascript-time-ago');
const en = require('javascript-time-ago/locale/en');
TimeAgo.addLocale(en);
const timeAgo = new TimeAgo('en-US');
const customStyle = {
steps: [
{ formatAs: 'second' },
{ formatAs: 'minute' },
{ formatAs: 'hour' },
{ formatAs: 'day' },
{ formatAs: 'week' },
{ formatAs: 'month' },
{ formatAs: 'year' }
]
};
console.log(timeAgo.format(new Date(Date.now() - 60 * 1000), customStyle)); // '1 minute ago'
Localization
This feature supports multiple languages and locales, allowing you to format dates in different languages. The example shows how to set up the package for both English and Spanish.
const TimeAgo = require('javascript-time-ago');
const en = require('javascript-time-ago/locale/en');
const es = require('javascript-time-ago/locale/es');
TimeAgo.addLocale(en);
TimeAgo.addLocale(es);
const timeAgoEn = new TimeAgo('en-US');
const timeAgoEs = new TimeAgo('es-ES');
console.log(timeAgoEn.format(new Date(Date.now() - 60 * 1000))); // '1 minute ago'
console.log(timeAgoEs.format(new Date(Date.now() - 60 * 1000))); // 'hace 1 minuto'
Moment.js is a widely-used library for parsing, validating, manipulating, and formatting dates. It offers extensive functionality for date and time manipulation, but it is larger in size compared to `javascript-time-ago` and has been deprecated in favor of more modern solutions.
date-fns provides a comprehensive set of functions for working with dates in JavaScript. It is modular, allowing you to import only the functions you need, which can result in smaller bundle sizes. It also supports internationalization, similar to `javascript-time-ago`.
timeago.js is a small library used to format dates in a 'time ago' style. It is lightweight and easy to use, but it may not offer as many customization options or support for as many locales as `javascript-time-ago`.
[![NPM Version][npm-badge]][npm] [![Build Status][travis-badge]][travis] [![Test Coverage][coveralls-badge]][coveralls]
International higly customizable relative date/time formatter (both for past and future dates).
Formats a date to something like:
npm install intl-messageformat@^1.3.0 --save
npm install javascript-time-ago --save
This package assumes that the [Intl
][Intl] global object exists in the runtime. Intl
is present in all modern browsers except Safari (which can be solved with the Intl polyfill).
Node.js 0.12 has the Intl
APIs built-in, but only includes the English locale data by default. If your app needs to support more locales than English, you'll need to get Node to load the extra locale data, or (a much simpler approach) just install the Intl polyfill.
If you decide you need the Intl polyfill then here are some basic installation and configuration instructions
import javascript_time_ago from 'javascript-time-ago'
// Load locale specific relative date/time messages
//
javascript_time_ago.locale(require('javascript-time-ago/locales/en'))
javascript_time_ago.locale(require('javascript-time-ago/locales/ru'))
// Load number pluralization functions for the locales.
// (the ones that decide if a number is gonna be
// "zero", "one", "two", "few", "many" or "other")
// http://cldr.unicode.org/index/cldr-spec/plural-rules
// https://github.com/eemeli/make-plural.js
// http://www.unicode.org/cldr/charts/latest/supplemental/language_plural_rules.html
//
require('javascript-time-ago/intl-messageformat-global')
require('intl-messageformat/dist/locale-data/en')
require('intl-messageformat/dist/locale-data/ru')
// Initialization complete.
// Ready to format relative dates and times.
const time_ago_english = new javascript_time_ago('en-US')
time_ago_english.format(new Date())
// "just now"
time_ago_english.format(new Date(Date.now() - 60 * 1000))
// "a minute ago"
time_ago_english.format(new Date(Date.now() - 2 * 60 * 60 * 1000))
// "2 hours ago"
time_ago_english.format(new Date(Date.now() - 24 * 60 * 60 * 1000))
// "a day ago"
const time_ago_russian = new javascript_time_ago('ru-RU')
time_ago_russian.format(new Date())
// "только что"
time_ago_russian.format(new Date(Date.now() - 60 * 1000)))
// "минуту назад"
time_ago_russian.format(new Date(Date.now() - 2 * 60 * 60 * 1000)))
// "2 часа назад"
time_ago_russian.format(new Date(Date.now() - 24 * 60 * 60 * 1000))
// "вчера"
Mimics Twitter style time ago ("1m", "2h", "Mar 3", "Apr 4, 2012")
…
const time_ago = new javascript_time_ago('en-US')
// A `style` is simply an `options` object
// passed to the `.format()` function as a second parameter.
const twitter = time_ago.style.twitter()
time_ago.format(new Date(), twitter)
// ""
time_ago.format(new Date(Date.now() - 60 * 1000), twitter)
// "1m"
time_ago.format(new Date(Date.now() - 2 * 60 * 60 * 1000), twitter)
// "2h"
time_ago.style.fuzzy()
The time scale is (actually same as the default style but with "ago" omitted):
To install the Intl polyfill (supporting 200+ languages):
npm install intl --save
Then configure the Intl polyfill:
This library currently comes with English and Russian localization built-in, but any other locale can be added easily at runtime (Pull Requests adding new locales are accepted too).
The built-in localization resides in the locales
folder.
The format of the localization is:
{
…
"day":
{
"previous": "a day ago",
"now": "today",
"next": "in a day",
"past":
{
"one": "{0} day ago",
"other": "{0} days ago"
},
"future":
{
"one": "in {0} day",
"other": "in {0} days"
}
},
…
}
The past
and future
keys can be one of: zero
, one
, two
, few
, many
and other
. For more info of which is which read the official Unicode CLDR documentation. [Unicode CLDR][CLDR] (Common Locale Data Repository) is an industry standard and is basically a collection of formatting rules for all locales (date, time, currency, measurement units, numbers, etc).
One can also use raw Unicode CLDR locale rules which will be automatically converted to the format described above.
Example for en-US-POSIX locale
{
"main": {
"en-US-POSIX": {
"dates": {
"fields": {
…
"day": {
"displayName": "day", // ignored
"relative-type--1": "yesterday", // ignored
"relative-type-0": "today", // ignored
"relative-type-1": "tomorrow", // ignored
"relativeTime-type-future": {
"relativeTimePattern-count-one" : "in {0} day",
"relativeTimePattern-count-other" : "in {0} days"
},
"relativeTime-type-past": {
"relativeTimePattern-count-one" : "{0} day ago",
"relativeTimePattern-count-other" : "{0} days ago"
}
},
…
}
}
}
}
}
To add support for a specific language one can download the CLDR dates package:
npm install cldr-dates-modern --save
And then add the neccessary locales from it:
import javascript_time_ago from 'javascript-time-ago'
import russian from 'cldr-dates-modern/main/ru/dateFields.json'
javascript_time_ago.locale('ru', russian.main.ru.dates.fields)
const time_ago = new javascript_time_ago('ru')
time_ago.format(new Date(Date.now() - 60 * 1000))
// "1 минуту назад"
No locales are loaded by default. This is done to allow tools like Webpack take advantage of code splitting to reduce the resulting javascript bundle size.
But server side doesn't need code splitting, so to load all available locales in Node.js you can use this shortcut:
// A faster way to load all the localization data for Node.js
// (`intl-messageformat` will load everything automatically when run in Node.js)
require('javascript-time-ago/load-all-locales')
Localization data described in the above section can be further customized, for example, supporting "long" and "short" formats. Refer to locales/en.js
for an example.
Built-in localization data is presented in different variants. Example:
import english from 'javascript-time-ago/locales/en'
english.tiny // '1s', '2m', '3h', '4d', …
english.short // '1 sec. ago', '2 min. ago', …
english.long // '1 second ago', '2 minutes ago', …
One can pass options
as a second parameter to the .format(date, options)
function. It's called a style
(see "twitter" style for example). The options
object can specify:
flavour
– preferred labels style (e.g. tiny
, short
, long
)units
– a list of time interval measurement units which can be used in the formatted output (e.g. ['second', 'minute', 'hour']
)gradation
– custom time interval measurement units gradationoverride
– is a function of { elapsed, time, date, now }
. If the override
function returns a value, then the .format()
call will return that value. Otherwise it has no effect.A gradation
is a list of time interval measurement steps. A simple example:
[
{
unit: 'second',
},
{
unit: 'minute',
factor: 60,
threshold: 59.5
},
{
unit: 'hour',
factor: 60 * 60,
threshold: 59.5 * 60
},
…
]
factor
is a divider for the supplied time interval (in seconds)threshold
is a minimum time interval value (in seconds) required for this gradation stepthreshold
customization is possible, see the link below)granularity
can also be specified (for example, 5
for minute
to allow only 5-minute intervals)For more gradation examples see source/classify elapsed.js
Available gradations:
import { gradation } from 'javascript-time-ago'
gradation.canonical() // '1 second ago', '2 minutes ago', …
gradation.convenient() // 'just now', '5 minutes ago', …
There is also a React component.
After cloning this repo, ensure dependencies are installed by running:
npm install
This module is written in ES6 and uses Babel for ES5 transpilation. Widely consumable JavaScript can be produced by running:
npm run build
Once npm run build
has run, you may import
or require()
directly from
node.
After developing, the full test suite can be evaluated by running:
npm test
While actively developing, one can use (personally I don't use it)
npm run watch
in a terminal. This will watch the file system and run tests automatically whenever you save a js file.
When you're ready to test your new functionality on a real project, you can run
npm pack
It will build
, test
and then create a .tgz
archive which you can then install in your project folder
npm install [module name with version].tar.gz
MIT [npm]: https://www.npmjs.org/package/javascript-time-ago [npm-badge]: https://img.shields.io/npm/v/javascript-time-ago.svg?style=flat-square [travis]: https://travis-ci.org/halt-hammerzeit/javascript-time-ago [travis-badge]: https://img.shields.io/travis/halt-hammerzeit/javascript-time-ago/master.svg?style=flat-square [CLDR]: http://cldr.unicode.org/ [Intl]: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Intl [coveralls]: https://coveralls.io/r/halt-hammerzeit/javascript-time-ago?branch=master [coveralls-badge]: https://img.shields.io/coveralls/halt-hammerzeit/javascript-time-ago/master.svg?style=flat-square
FAQs
Localized relative date/time formatting
The npm package javascript-time-ago receives a total of 289,690 weekly downloads. As such, javascript-time-ago popularity was classified as popular.
We found that javascript-time-ago demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.