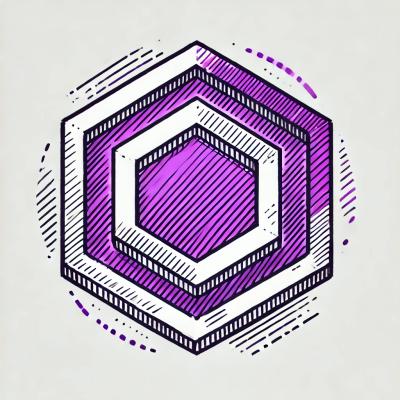
Security News
ESLint is Now Language-Agnostic: Linting JSON, Markdown, and Beyond
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
javascript-time-ago
Advanced tools
The `javascript-time-ago` npm package is used to format dates and times in a human-readable way, such as '5 minutes ago' or '2 days ago'. It supports multiple languages and locales, making it a versatile tool for internationalization.
Basic Usage
This feature allows you to format a date into a human-readable string indicating the time elapsed since that date. The example shows how to set up the package for English and format a date to '1 minute ago'.
const TimeAgo = require('javascript-time-ago');
const en = require('javascript-time-ago/locale/en');
TimeAgo.addLocale(en);
const timeAgo = new TimeAgo('en-US');
console.log(timeAgo.format(new Date(Date.now() - 60 * 1000))); // '1 minute ago'
Custom Styles
This feature allows you to define custom styles for formatting the elapsed time. The example demonstrates how to create a custom style and use it to format a date.
const TimeAgo = require('javascript-time-ago');
const en = require('javascript-time-ago/locale/en');
TimeAgo.addLocale(en);
const timeAgo = new TimeAgo('en-US');
const customStyle = {
steps: [
{ formatAs: 'second' },
{ formatAs: 'minute' },
{ formatAs: 'hour' },
{ formatAs: 'day' },
{ formatAs: 'week' },
{ formatAs: 'month' },
{ formatAs: 'year' }
]
};
console.log(timeAgo.format(new Date(Date.now() - 60 * 1000), customStyle)); // '1 minute ago'
Localization
This feature supports multiple languages and locales, allowing you to format dates in different languages. The example shows how to set up the package for both English and Spanish.
const TimeAgo = require('javascript-time-ago');
const en = require('javascript-time-ago/locale/en');
const es = require('javascript-time-ago/locale/es');
TimeAgo.addLocale(en);
TimeAgo.addLocale(es);
const timeAgoEn = new TimeAgo('en-US');
const timeAgoEs = new TimeAgo('es-ES');
console.log(timeAgoEn.format(new Date(Date.now() - 60 * 1000))); // '1 minute ago'
console.log(timeAgoEs.format(new Date(Date.now() - 60 * 1000))); // 'hace 1 minuto'
Moment.js is a widely-used library for parsing, validating, manipulating, and formatting dates. It offers extensive functionality for date and time manipulation, but it is larger in size compared to `javascript-time-ago` and has been deprecated in favor of more modern solutions.
date-fns provides a comprehensive set of functions for working with dates in JavaScript. It is modular, allowing you to import only the functions you need, which can result in smaller bundle sizes. It also supports internationalization, similar to `javascript-time-ago`.
timeago.js is a small library used to format dates in a 'time ago' style. It is lightweight and easy to use, but it may not offer as many customization options or support for as many locales as `javascript-time-ago`.
International higly customizable relative date/time formatter (both for past and future dates).
Formats a date/timestamp to:
For React users there's also a React component.
This is a readme for version 2.x
. For older versions, see version 1.x
readme. See a migration guide for migrating from version 1.x
to version 2.x
.
npm install javascript-time-ago --save
import TimeAgo from 'javascript-time-ago'
// Load locale-specific relative date/time formatting rules and labels.
import en from 'javascript-time-ago/locale/en'
// Add locale-specific relative date/time formatting rules and labels.
TimeAgo.addLocale(en)
// Create relative date/time formatter.
const timeAgo = new TimeAgo('en-US')
timeAgo.format(new Date())
// "just now"
timeAgo.format(Date.now() - 60 * 1000)
// "a minute ago"
timeAgo.format(Date.now() - 2 * 60 * 60 * 1000)
// "2 hours ago"
timeAgo.format(Date.now() - 24 * 60 * 60 * 1000)
// "a day ago"
This library includes date/time formatting rules and labels for any language.
No languages are loaded default: a developer must manually choose which languages should be loaded. The languages should be imported from javascript-time-ago/locale
and then added via TimeAgo.addLocale(...)
. An example of using Russian language:
import TimeAgo from 'javascript-time-ago'
// Load locale-specific relative date/time formatting rules and labels.
import en from 'javascript-time-ago/locale/en'
import ru from 'javascript-time-ago/locale/ru'
// Add locale-specific relative date/time formatting rules and labels.
// "en" is the default (fallback) locale.
// (that could be changed via `TimeAgo.setDefaultLocale(...)`)
TimeAgo.addLocale(en)
TimeAgo.addLocale(ru)
// cyka blyat
const timeAgo = new TimeAgo('ru-RU')
timeAgo.format(new Date())
// "только что"
timeAgo.format(Date.now() - 60 * 1000)
// "1 минуту назад"
timeAgo.format(Date.now() - 2 * 60 * 60 * 1000)
// "2 часа назад"
timeAgo.format(Date.now() - 24 * 60 * 60 * 1000)
// "1 день назад"
This library allows for any custom logic for formatting time difference labels:
What scale should be used for measuring the time difference: should it be precise down to the second, or should it only calculate it up to a minute, or should it start from being more precise at the start and then gradually decrease its precision as the time goes on.
What labels should be used: should it use the standard built-in labels for the languages ("... minutes ago"
, "... min. ago"
, "...s"
), or should it use custom ones, or should it skip using relative time labels in some cases and instead output something like "Dec 11, 2015"
.
Such configuration comes under the name of "preset".
While a completely custom preset could be supplied, this library comes with several built-in presets that some people might find useful.
Historically it's not the default preset, but it goes under the name of "default".
timeAgo.format(Date.now() - 60 * 1000, 'default')
// "1 minute ago"
The "approximate" preset is (historically) the default one.
timeAgo.format(Date.now() - 60 * 1000)
// "1 minute ago"
The "twitter" preset mimics Twitter style of time ago ("1m", "2h", "Mar 3", "Apr 4, 2012")
timeAgo.format(new Date() - 1, 'twitter')
// "1s"
timeAgo.format(Date.now() - 60 * 1000, 'twitter')
// "1m"
timeAgo.format(Date.now() - 2 * 60 * 60 * 1000, 'twitter')
// "2h"
timeAgo.format(Date.now() - 2 * 24 * 60 * 60 * 1000, 'twitter')
// "Mar 3"
timeAgo.format(Date.now() - 365 * 24 * 60 * 60 * 1000, 'twitter')
// "Mar 5, 2017"
The "twitter" preset uses Intl
for formatting day/month/year
labels. If Intl
is not available (for example, in Internet Explorer), it falls back to the default labels for months/years intervals: "1 mo. ago"
/"1 yr. ago"
.
Not all locales are applicable for this preset: only those having tiny.json
time labels.
Same as the "approximate" preset but without the "ago" part. I guess this preset should be considered a "legacy" one and will be removed in the next major version.
timeAgo.format(Date.now() - 60 * 1000, 'time')
// "1 minute"
Not all locales are applicable for this preset: only those having long-time.json
.
The above sections explained all the basics required for using this library in a project.
This part of the documentation contains some advanced topics for those willing to have a better understanding of how this library works internally.
This library comes with several "presets" built-in. Each of those presets is an object defining its own flavour
(the name's historical) and gradation
. A completely custom "preset" object may be passed as a second parameter to .format(date, preset)
, having the following shape:
flavour
– Preferred time labels style. Is "long"
by default. Can be either a string (e.g. "short"
) or an array of preferred "flavours" in which case each one of them is tried until a supported one is found. For example, ["tiny", "short"]
will search for tiny
time labels first and then fall back to short
ones if tiny
time labels aren't defined for the language. short
, long
and narrow
time labels are always present for each language.
gradation
– Time interval measurement units scale. Is convenient
by default. Another one available is canonical
. A developer may supply a custom gradation
which must be an array of steps each of them having either a unit: string
or a format(value, locale): string
function. See Twitter preset for such an advanced example.
units
– A list of time interval measurement units which can be used in the output. E.g. ["second", "minute", "hour", ...]
. By default all available units are used. This is only used to filter out some of the non-conventional time units like "quarter"
which is present in CLDR data.
(the name's historical; will be renamed to "style" in the next major version)
Relative date/time labels come in various styles: long
, short
, narrow
are the standard CLDR ones (always present), possibly accompanied by other ones like tiny
("1m"
, "2h"
, ...). Refer to locale/en
for an example.
import english from 'javascript-time-ago/locale/en'
english.tiny // '1s', '2m', '3h', '4d', …
english.narrow // '1 sec. ago', '2 min. ago', …
english.short // '1 sec. ago', '2 min. ago', …
english.long // '1 second ago', '2 minutes ago', …
tiny
is supposed to be the shortest one possible. It's not a CLDR-defined one and has been defined only for a small subset of languages (en
, ru
, ko
, and several others).
narrow
is a CLDR-defined one and is intended to be shorter than short
, or at least no longer than it. I personally find narrow
a weird one because for some locales it's the same as short
and for other locales it's a really weird one (e.g. for Russian).
short
is "short".
long
is "regular".
A gradation
is a list of time interval measurement steps.
[
{
unit: 'second',
},
{
unit: 'minute',
factor: 60,
threshold: 59.5
},
{
unit: 'hour',
factor: 60 * 60,
threshold: 59.5 * 60
},
…
]
Each step is described by:
unit
— a localized time measurement unit: second
, minute
, hour
, day
, month
, year
are the standardized CLDR ones.factor
— a divider for the supplied time interval (in seconds).threshold
— a minimum time interval value (in seconds) required for this gradation step. Each step must have a threshold
defined except for the first one. Can a number
or a function(now: number, future: boolean)
returning a number
. Some advanced threshold
customization is possible like threshold_for_[prev-unit]
(see ./source/gradation/convenient.js
).granularity
— for example, 5
for minute
to allow only 5-minute intervals: 0 minutes
, 5 minutes
, 10 minutes
, etc.If a gradation step should output not simply a time interval of a certain time unit but something different instead then it may be described by:
threshold
— same as above.format
— a function(value, locale)
returning a string
. value
argument is the date/time being formatted as passed to TimeAgo.format(value)
: either a number
or a Date
. locale
argument is the selected locale (aka "BCP 47 language tag", e.g. ru-RU
). For example, the built-in Twitter gradation has regular minute
and hour
steps followed by a custom one formatting a date as "day/month/year", e.g. Jan 24, 2018
.For more gradation examples see source/gradation
folder.
Built-in gradations:
import {
canonical, // '1 second ago', '2 minutes ago', …
convenient // 'just now', '5 minutes ago', …
} from 'javascript-time-ago/gradation'
When given future dates .format()
produces the corresponding output, e.g. "in 5 minutes", "in a year", etc.
The default locale is en
and can be changed: TimeAgo.setDefaultLocale('ru')
.
This library is based on Intl.RelativeTimeFormat
.
There is also a React component built upon this library which autorefreshes itself.
(this is an "advanced" section)
Intl
global object is not required for this library, but, for example, if you choose to use the built-in twitter
style then it will fall back to the default style if Intl
is not available.
Intl
is present in all modern web browsers and is absent from some of the old ones: Internet Explorer 10, Safari 9 and iOS Safari 9.x (which can be solved using Intl
polyfill).
Node.js starting from 0.12
has Intl
built-in, but only includes English locale data by default. If your app needs to support more locales than English on server side (e.g. Server-Side Rendering) then you'll need to use Intl
polyfill.
An example of applying Intl
polyfill:
npm install intl@1.2.4 --save
Node.js
import IntlPolyfill from 'intl'
const locales = ['en', 'ru', ...]
if (typeof Intl === 'object') {
if (!Intl.DateTimeFormat || Intl.DateTimeFormat.supportedLocalesOf(locales).length !== locales.length) {
Intl.DateTimeFormat = IntlPolyfill.DateTimeFormat
}
} else {
global.Intl = IntlPolyfill
}
Web browser: only download intl
package if the web browser doesn't support it, and only download the required locale.
async function initIntl() {
if (typeof Intl === 'object') {
return
}
await Promise.all([
import('intl'),
import('intl/locale-data/jsonp/en'),
import('intl/locale-data/jsonp/ru'),
...
])
}
initIntl().then(...)
FAQs
Localized relative date/time formatting
The npm package javascript-time-ago receives a total of 197,934 weekly downloads. As such, javascript-time-ago popularity was classified as popular.
We found that javascript-time-ago demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
ESLint has added JSON and Markdown linting support with new officially-supported plugins, expanding its versatility beyond JavaScript.
Security News
Members Hub is conducting large-scale campaigns to artificially boost Discord server metrics, undermining community trust and platform integrity.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.