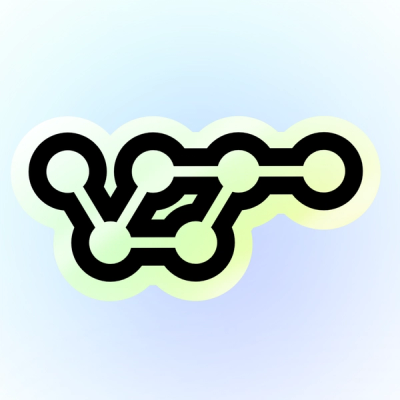
Security News
vlt Debuts New JavaScript Package Manager and Serverless Registry at NodeConf EU
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
jest-cucumber
Advanced tools
jest-cucumber is an npm package that allows you to write BDD (Behavior-Driven Development) tests using Gherkin syntax and run them with Jest. It bridges the gap between business stakeholders and developers by enabling the creation of human-readable test scenarios.
Define Feature Files
You can define your test scenarios in Gherkin syntax, which is a human-readable format. This makes it easier for non-developers to understand the test cases.
Feature: Addition
Scenario: Add two numbers
Given I have entered 50 into the calculator
And I have entered 70 into the calculator
When I press add
Then the result should be 120 on the screen
Step Definitions
You can define step definitions in JavaScript to map the Gherkin steps to actual test code. This allows you to implement the logic for each step in your test scenarios.
const { defineFeature, loadFeature } = require('jest-cucumber');
const feature = loadFeature('./features/addition.feature');
defineFeature(feature, test => {
test('Add two numbers', ({ given, when, then }) => {
let calculator;
given('I have entered 50 into the calculator', () => {
calculator = new Calculator();
calculator.enter(50);
});
given('I have entered 70 into the calculator', () => {
calculator.enter(70);
});
when('I press add', () => {
calculator.add();
});
then('the result should be 120 on the screen', () => {
expect(calculator.result).toBe(120);
});
});
});
Integration with Jest
jest-cucumber integrates seamlessly with Jest, allowing you to run your BDD tests using Jest's test runner. This means you can leverage Jest's powerful features like mocking, snapshot testing, and parallel test execution.
const { defineFeature, loadFeature } = require('jest-cucumber');
const feature = loadFeature('./features/addition.feature');
defineFeature(feature, test => {
test('Add two numbers', ({ given, when, then }) => {
let calculator;
given('I have entered 50 into the calculator', () => {
calculator = new Calculator();
calculator.enter(50);
});
given('I have entered 70 into the calculator', () => {
calculator.enter(70);
});
when('I press add', () => {
calculator.add();
});
then('the result should be 120 on the screen', () => {
expect(calculator.result).toBe(120);
});
});
});
// Run the tests using Jest
// jest
Cucumber is a widely-used BDD framework that supports multiple languages including JavaScript. It allows you to write tests in Gherkin syntax and provides a rich set of features for defining and running BDD tests. Compared to jest-cucumber, Cucumber is more feature-rich but may require more setup and configuration.
CodeceptJS is an end-to-end testing framework that supports BDD-style tests using Gherkin syntax. It integrates with various test runners and provides a high-level API for writing tests. While it offers more flexibility in terms of test runners and integrations, it may not be as tightly integrated with Jest as jest-cucumber.
Nightwatch is an end-to-end testing framework that supports BDD-style tests using Gherkin syntax. It is designed for browser automation and provides a simple API for writing and running tests. Compared to jest-cucumber, Nightwatch is more focused on browser automation and may not offer the same level of integration with Jest.
Execute Gherkin scenarios in Jest
jest-cucumber is an alternative to Cucumber.js that runs on top on Jest. Instead of using describe
and it
blocks, you instead write a Jest test for each scenario, and then define Given
, When
, and Then
step definitions inside of your Jest tests. jest-cucumber then allows you to link these Jest tests to your feature files and ensure that they always stay in sync.
Jest is an excellent test runner with great features like parallel test execution, mocking, snapshots, code coverage, etc. If you're using VS Code, there's also a terrific Jest extension that allows you get realtime feedback as you're writing your tests and easily debug failing tests individually. Cucumber is a popular tool for doing Acceptance Test-Driven Development and creating business-readable executable specifications. This library aims to achieve the best of both worlds, and even run your unit tests and acceptance tests in the same test runner.
npm install jest jest-cucumber --save-dev
Feature: Logging in
Scenario: Entering a correct password
Given I have previously created a password
When I enter my password correctly
Then I should be granted access
"testMatch": [
"**/*.steps.js"
],
// logging-in.steps.js
import { defineFeature, loadFeature } from 'jest-cucumber';
const feature = loadFeature('features/LoggingIn.feature');
// logging-in.steps.js
import { defineFeature, loadFeature } from 'jest-cucumber';
const feature = loadFeature('features/LoggingIn.feature');
defineFeature(feature, test => {
test('Entering a correct password', ({ given, when, then }) => {
});
});
// logging-in.steps.js
import { loadFeature, defineFeature } from 'jest-cucumber';
import { PasswordValidator } from 'src/password-validator';
const feature = loadFeature('specs/features/basic-scenarios.feature');
defineFeature(feature, (test) => {
let passwordValidator = new PasswordValidator();
let accessGranted = false;
beforeEach(() => {
passwordValidator = new PasswordValidator();
});
test('Entering a correct password', ({ given, when, then }) => {
given('I have previously created a password', () => {
passwordValidator.setPassword('1234');
});
when('I enter my password correctly', () => {
accessGranted = passwordValidator.validatePassword('1234');
});
then('I should be granted access', () => {
expect(accessGranted).toBe(true);
});
});
});
If you prefer an experience more like Cucumber with global step matching and the ability to define steps exactly once that can be matched to multiple steps across multiple feature files, then Jest Cucumber does accommodate this preference with autoBindSteps.
However, the default mode in Jest Cucumber can be thought of as Cucumber reimagined for Jest, and is designed for writing Jest tests that are kept in sync with Gherkin feature files. The goal is that your Jest tests (i.e., step definitions) are perfectly readable by themselves without jumping back and forth between step definitions and feature files. Another goal is to avoid global step matching, which many people find problematic and difficult to maintain as a codebase grows. By default, Jest Cucumber expects that your step definitions and feature files match exactly, and will report errors / generate suggested code when they are out of sync. To avoid duplicated step code, you can use the techniques described here.
FAQs
Execute Gherkin scenarios in Jest
The npm package jest-cucumber receives a total of 183,205 weekly downloads. As such, jest-cucumber popularity was classified as popular.
We found that jest-cucumber demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt introduced its new package manager and a serverless registry this week, innovating in a space where npm has stagnated.
Security News
Research
The Socket Research Team uncovered a malicious Python package typosquatting the popular 'fabric' SSH library, silently exfiltrating AWS credentials from unsuspecting developers.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.