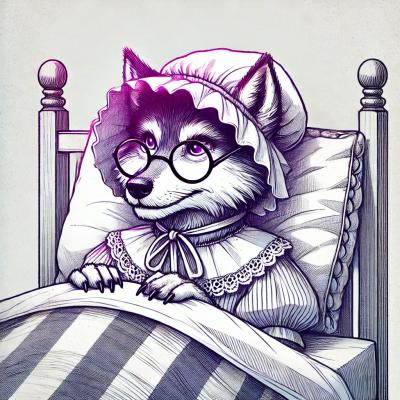
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
jest-docblock
Advanced tools
`jest-docblock` is a package that can extract and parse a specially-formatted comment called a "docblock" at the top of a file.
The jest-docblock package is used to parse and manipulate docblocks - comments at the top of a file that are often used to provide metadata about the file, such as descriptions, authors, and licenses. It is commonly used in the Jest testing framework to handle such metadata in test files.
Parsing docblocks
This feature allows you to parse the contents of a file to extract the metadata contained within a docblock. The parse function returns an object where each key is a tag from the docblock.
const { parse } = require('jest-docblock');
const contents = `/**
* This is a docblock
*
* @flow
*/
const foo = 'bar';`;
const docblock = parse(contents);
console.log(docblock);
Extracting docblock
This feature allows you to extract the raw docblock as a string from the contents of a file. This can be useful if you want to manipulate the docblock as a whole or use it for documentation purposes.
const { extract } = require('jest-docblock');
const contents = `/**
* This is a docblock
*
* @flow
*/
const foo = 'bar';`;
const docblockString = extract(contents);
console.log(docblockString);
Printing docblock
This feature allows you to take an object representing a docblock and print it back into the docblock format. This is useful for modifying or adding new metadata to a file's docblock.
const { print } = require('jest-docblock');
const docblock = { flow: '' };
const newContents = print(docblock);
console.log(newContents);
The comment-parser package is similar to jest-docblock in that it is used to parse comments in code. It supports parsing of block comments in various formats and is not tied to any specific testing framework, making it more flexible for different use cases.
Doctrine is another JSDoc parsing library that can extract type information and other metadata from JSDoc comments. It is more focused on the JSDoc standard and provides a detailed AST of the parsed comments, which can be more powerful but also more complex than jest-docblock.
jsdoc-to-markdown is a package that takes JSDoc comments and converts them into markdown documentation. While it is not a direct alternative to jest-docblock, it serves a related purpose by transforming code comments into user-friendly documentation.
jest-docblock
is a package that can extract and parse a specially-formatted
comment called a "docblock" at the top of a file.
A docblock looks like this:
/**
* Stuff goes here!
*/
Docblocks can contain pragmas, which are words prefixed by @
:
/**
* Pragma incoming!
*
* @flow
*/
Pragmas can also take arguments:
/**
* Check this out:
*
* @myPragma it is so cool
*/
jest-docblock
can:
# with yarn
$ yarn add jest-docblock
# with npm
$ npm install jest-docblock
const code = `
/**
* Everything is awesome!
*
* @everything is:awesome
* @flow
*/
export const everything = Object.create(null);
export default function isAwesome(something) {
return something === everything;
}
`;
const {
extract,
strip,
parse,
parseWithComments,
print,
} = require('jest-docblock');
const docblock = extract(code);
console.log(docblock); // "/**\n * Everything is awesome!\n * \n * @everything is:awesome\n * @flow\n */"
const stripped = strip(code);
console.log(stripped); // "export const everything = Object.create(null);\n export default function isAwesome(something) {\n return something === everything;\n }"
const pragmas = parse(docblock);
console.log(pragmas); // { everything: "is:awesome", flow: "" }
const parsed = parseWithComments(docblock);
console.log(parsed); // { comments: "Everything is awesome!", pragmas: { everything: "is:awesome", flow: "" } }
console.log(print({pragmas, comments: 'hi!'})); // /**\n * hi!\n *\n * @everything is:awesome\n * @flow\n */;
extract(contents: string): string
Extracts a docblock from some file contents. Returns the docblock contained in
contents
. If contents
did not contain a docblock, it will return the empty
string (""
).
strip(contents: string): string
Strips the top docblock from a file and return the result. If a file does not have a docblock at the top, then return the file unchanged.
parse(docblock: string): {[key: string]: string}
Parses the pragmas in a docblock string into an object whose keys are the pragma tags and whose values are the arguments to those pragmas.
parseWithComments(docblock: string): { comments: string, pragmas: {[key: string]: string} }
Similar to parse
except this method also returns the comments from the
docblock. Useful when used with print()
.
print({ comments?: string, pragmas?: {[key: string]: string} }): string
Prints an object of key-value pairs back into a docblock. If comments
are
provided, they will be positioned on the top of the docblock.
jest 22.0.0
[jest-resolve]
Use module.builtinModules
as BUILTIN_MODULES
when it exists[jest-worker]
Remove debug
and inspect
flags from the arguments sent to the child (#5068)[jest-config]
Use all --testPathPattern
and <regexForTestFiles>
args in testPathPattern
(#5066)[jest-cli]
Do not support --watch
inside non-version-controlled environments (#5060)[jest-config]
Escape Windows path separator in testPathPattern CLI arguments (#5054)[jest-jasmine]
Register sourcemaps as node environment to improve performance with jsdom (#5045)[pretty-format]
Do not call toJSON recursively (#5044)[pretty-format]
Fix errors when identity-obj-proxy mocks CSS Modules (#4935)[babel-jest]
Fix support for namespaced babel version 7 (#4918)[expect]
fix .toThrow for promises (#4884)[jest-docblock]
pragmas should preserve urls (#4837)[jest-cli]
Check if npm_lifecycle_script
calls Jest directly (#4629)[jest-cli]
Fix --showConfig to show all configs (#4494)[jest-cli]
Throw if maxWorkers
doesn't have a value (#4591)[jest-cli]
Use fs.realpathSync.native
if available (#5031)[jest-config]
Fix --passWithNoTests
(#4639)[jest-config]
Support rootDir
tag in testEnvironment (#4579)[jest-editor-support]
Fix --showConfig
to support jest 20 and jest 21 (#4575)[jest-editor-support]
Fix editor support test for node 4 (#4640)[jest-mock]
Support mocking constructor in mockImplementationOnce
(#4599)[jest-runtime]
Fix manual user mocks not working with custom resolver (#4489)[jest-util]
Fix runOnlyPendingTimers
for setTimeout
inside setImmediate
(#4608)[jest-message-util]
Always remove node internals from stacktraces (#4695)[jest-resolve]
changes method of determining builtin modules to include missing builtins (#4740)[pretty-format]
Prevent error in pretty-format for window in jsdom test env (#4750)[jest-resolve]
Preserve module identity for symlinks (#4761)[jest-config]
Include error message for preset
json (#4766)[pretty-format]
Throw PrettyFormatPluginError
if a plugin halts with an exception (#4787)[expect]
Keep the stack trace unchanged when PrettyFormatPluginError
is thrown by pretty-format (#4787)[jest-environment-jsdom]
Fix asynchronous test will fail due to timeout issue. (#4669)[jest-cli]
Fix --onlyChanged
path case sensitivity on Windows platform (#4730)[jest-runtime]
Use realpath to match transformers (#5000)[expect]
[BREAKING] Replace identity equality with Object.is in toBe matcher (#4917)[jest-message-util]
Add codeframe to test assertion failures (#5087)[jest-config]
Add Global Setup/Teardown options (#4716)[jest-config]
Add testEnvironmentOptions
to apply to jsdom options or node context. (#5003)[jest-jasmine2]
Update Timeout error message to jest.timeout
and display current timeout value (#4990)[jest-runner]
Enable experimental detection of leaked contexts (#4895)[jest-cli]
Add combined coverage threshold for directories. (#4885)[jest-mock]
Add timestamps
to mock state. (#4866)[eslint-plugin-jest]
Add prefer-to-have-length
lint rule. (#4771)[jest-environment-jsdom]
[BREAKING] Upgrade to JSDOM@11 (#4770)[jest-environment-*]
[BREAKING] Add Async Test Environment APIs, dispose is now teardown (#4506)[jest-cli]
Add an option to clear the cache (#4430)[babel-plugin-jest-hoist]
Improve error message, that the second argument of jest.mock
must be an inline function (#4593)[jest-snapshot]
[BREAKING] Concatenate name of test and snapshot (#4460)[jest-cli]
[BREAKING] Fail if no tests are found (#3672)[jest-diff]
Highlight only last of odd length leading spaces (#4558)[jest-docblock]
Add docblock.print()
(#4517)[jest-docblock]
Add strip
(#4571)[jest-docblock]
Preserve leading whitespace in docblock comments (#4576)[jest-docblock]
remove leading newlines from parswWithComments().comments
(#4610)[jest-editor-support]
Add Snapshots metadata (#4570)[jest-editor-support]
Adds an 'any' to the typedef for updateFileWithJestStatus
(#4636)[jest-editor-support]
Better monorepo support (#4572)[jest-environment-jsdom]
Add simple rAF polyfill in jsdom environment to work with React 16 (#4568)[jest-environment-node]
Implement node Timer api (#4622)[jest-jasmine2]
Add testPath to reporter callbacks (#4594)[jest-mock]
Added support for naming mocked functions with .mockName(value)
and .mockGetName()
(#4586)[jest-runtime]
Add module.loaded
, and make module.require
not enumerable (#4623)[jest-runtime]
Add module.parent
(#4614)[jest-runtime]
Support sourcemaps in transformers (#3458)[jest-snapshot]
[BREAKING] Add a serializer for jest.fn
to allow a snapshot of a jest mock (#4668)[jest-worker]
Initial version of parallel worker abstraction, say hello! (#4497)[jest-jasmine2]
Add testLocationInResults
flag to add location information per spec to test results (#4782)[jest-environment-jsdom]
Update JSOM to 11.4, which includes built-in support for requestAnimationFrame
(#4919)[jest-cli]
Hide watch usage output when running on non-interactive environments (#4958)[jest-snapshot]
Promises support for toThrowErrorMatchingSnapshot
(#4946)[jest-cli]
Explain which snapshots are obsolete (#5005)[docs]
Add guide of using with puppeteer (#5093)[jest-util]
jest-util
should not depend on jest-mock
(#4992)[*]
[BREAKING] Drop support for Node.js version 4 (#4769)[docs]
Wrap code comments at 80 characters (#4781)[eslint-plugin-jest]
Removed from the Jest core repo, and moved to https://github.com/jest-community/eslint-plugin-jest (#4867)[babel-jest]
Explicitly bump istanbul to newer versions (#4616)[expect]
Upgrade mocha and rollup for browser testing (#4642)[docs]
Add info about coveragePathIgnorePatterns
(#4602)[docs]
Add Vuejs series of testing with Jest (#4648)[docs]
Mention about optional done
argument in test function (#4556)[jest-cli]
Bump node-notifier version (#4609)[jest-diff]
Simplify highlight for leading and trailing spaces (#4553)[jest-get-type]
Add support for date (#4621)[jest-matcher-utils]
Call chalk.inverse
for trailing spaces (#4578)[jest-runtime]
Add .advanceTimersByTime
; keep .runTimersToTime()
as an alias.[docs]
Include missing dependency in TestEnvironment sample code[docs]
Add clarification for hook execution order[docs]
Update expect.anything()
sample code (#5007)FAQs
`jest-docblock` is a package that can extract and parse a specially-formatted comment called a "docblock" at the top of a file.
The npm package jest-docblock receives a total of 11,294,070 weekly downloads. As such, jest-docblock popularity was classified as popular.
We found that jest-docblock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.