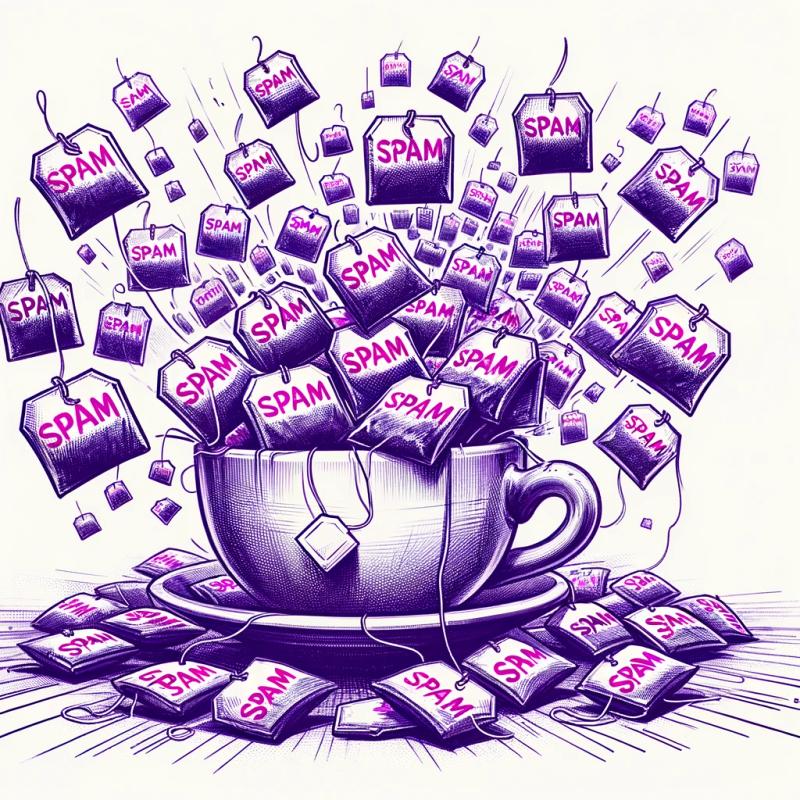
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
jest-environment-jsdom
Advanced tools
Package description
The jest-environment-jsdom package is a custom environment for Jest that allows you to simulate a browser-like environment using jsdom. This is useful for testing web applications without running them in an actual browser. It provides a simulated DOM API that you can interact with in your tests.
Simulating browser environment for testing
This feature allows you to simulate browser-like interactions such as clicking a button and then asserting the expected outcome, all within a Node.js environment.
test('simulates a click event', () => {
document.body.innerHTML = '<button id="button">Click me</button>';
const button = document.getElementById('button');
button.addEventListener('click', () => {
button.innerHTML = 'Clicked';
});
button.click();
expect(button.innerHTML).toBe('Clicked');
});
Testing DOM manipulation
You can test DOM manipulation by creating, modifying, and removing elements, and then asserting that these changes have taken place as expected.
test('adds a new div to the body', () => {
const div = document.createElement('div');
div.id = 'new-div';
document.body.appendChild(div);
expect(document.getElementById('new-div')).not.toBeNull();
});
Mocking global variables
The package allows you to mock global variables such as localStorage, which is useful for testing code that interacts with browser APIs that are not available in Node.js.
test('mocks a global variable', () => {
global.localStorage = {
getItem: jest.fn().mockReturnValue('mockValue')
};
expect(global.localStorage.getItem('key')).toBe('mockValue');
});
This package is similar to jest-environment-jsdom but is intended for testing Node.js applications. It does not simulate a browser environment and is therefore not suitable for testing browser-specific code.
Karma is a test runner that works with any framework and allows you to run tests in real browsers. Unlike jest-environment-jsdom, which simulates a browser environment, Karma actually runs tests in real browsers, which can be more accurate but also slower and more complex to set up.
This package integrates jsdom with Mocha, another testing framework. It provides similar functionality to jest-environment-jsdom but is designed to work with Mocha instead of Jest.
Enzyme is a testing utility for React that makes it easier to assert, manipulate, and traverse your React Components' output. It can be used in conjunction with jest-environment-jsdom to test React components in a simulated browser environment.
FAQs
Unknown package
The npm package jest-environment-jsdom receives a total of 14,840,814 weekly downloads. As such, jest-environment-jsdom popularity was classified as popular.
We found that jest-environment-jsdom demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.