jest-fail-on-console
Utility to make jest tests fail when console.error()
, console.warn()
, etc. are used

What problem is this solving?
Jest doesn't fail the tests when there is a console.error
. In large codebase, we can end up with the test output overloaded by a lot of errors, warnings, etc..
To prevent this, we want to fail each test that is logging to the console. We also want to conserve a clear output of the original error.
This is what this utility is doing.
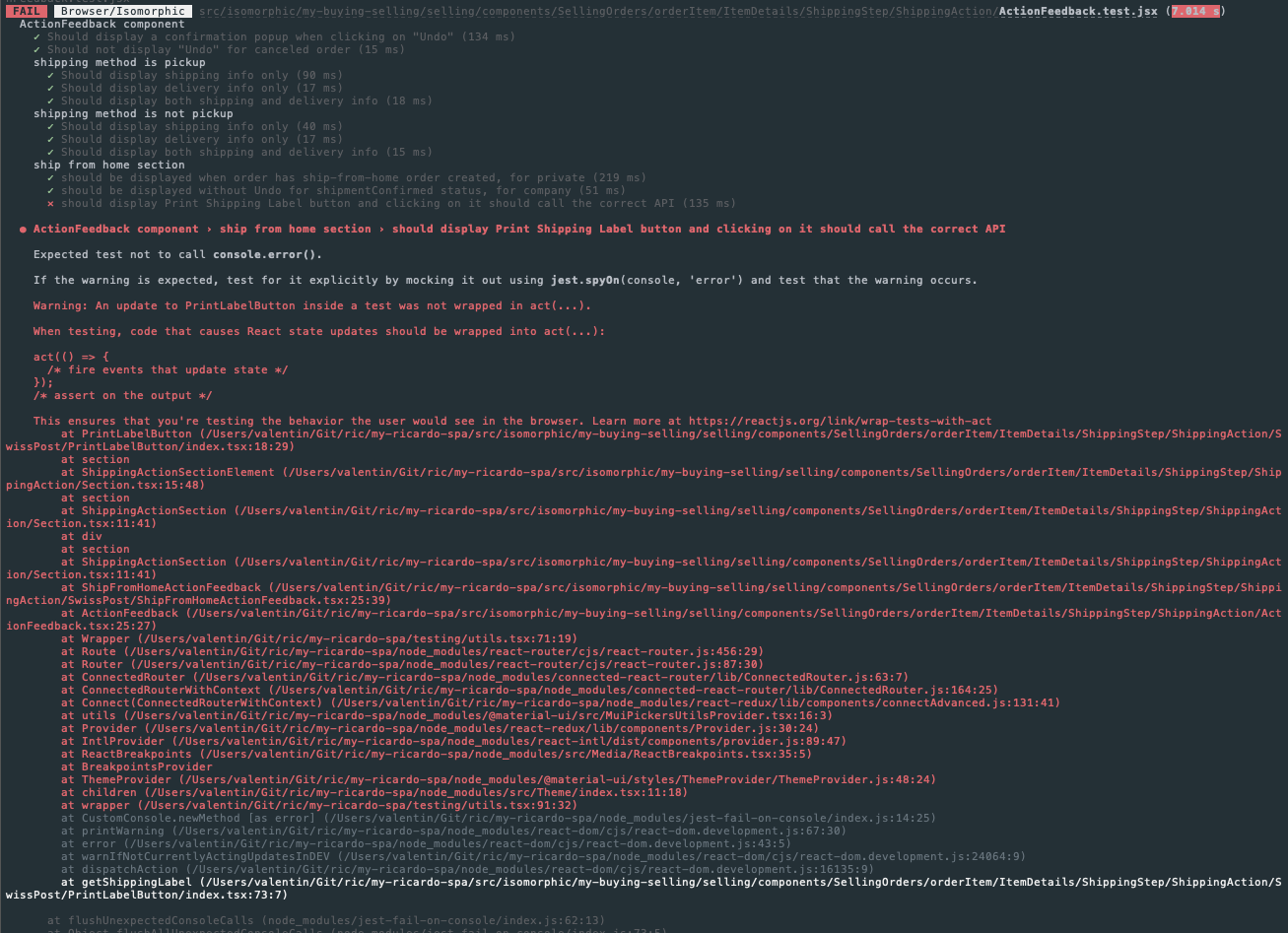
Downloads
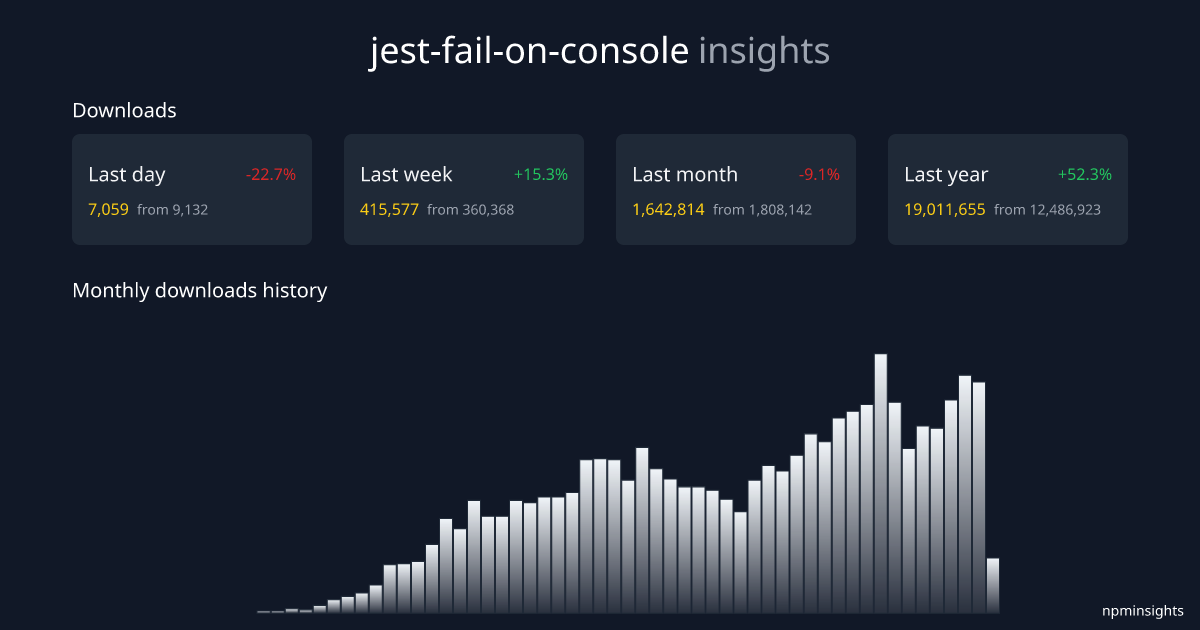
Install
yarn add -D jest-fail-on-console
or
npm install -D jest-fail-on-console
How to use
In a file used in the setupFilesAfterEnv
option of Jest, add this code:
import failOnConsole from 'jest-fail-on-console'
failOnConsole()
failOnConsole({
shouldFailOnWarn: false,
})
But I have some expected console errors/warning
If a console.error()
is expected, then you should assert for it:
test('should log an error', () => {
jest.spyOn(console, 'error').mockImplementation()
expect(console.error).toHaveBeenCalledWith('your error message')
})
Options
You can pass an object with options to the function:
errorMessage
Use this if you want to override the default error message of this library.
type errorMessage = (
methodName: 'assert' | 'debug' | 'error' | 'info' | 'log' | 'warn',
bold: (string: string) => string
) => string
shouldFailOnAssert
Use this to make a test fail when a console.assert()
is logged.
- Type:
boolean
- Default:
false
shouldFailOnDebug
Use this to make a test fail when a console.debug()
is logged.
- Type:
boolean
- Default:
false
shouldFailOnError
Use this to make a test fail when a console.error()
is logged.
- Type:
boolean
- Default:
true
shouldFailOnInfo
Use this to make a test fail when a console.info()
is logged.
- Type:
boolean
- Default:
false
shouldFailOnLog
Use this to make a test fail when a console.log()
is logged.
- Type:
boolean
- Default:
false
shouldFailOnWarn
Use this to make a test fail when a console.warn()
is logged.
- Type:
boolean
- Default:
true
allowMessage
type allowMessage = (
message: string,
methodName: 'assert' | 'debug' | 'error' | 'info' | 'log' | 'warn',
context: { group: string; groups: string[] }
) => boolean
This function is called for every console method supported by this utility.
If true
is returned, the message will show in the console and the test won't fail.
Example:
failOnConsole({
allowMessage: (errorMessage) => {
if (/An expected error/.test(errorMessage)) {
return true
}
return false
},
})
silenceMessage
type silenceMessage = (
message: string,
methodName: 'assert' | 'debug' | 'error' | 'info' | 'log' | 'warn',
context: { group: string; groups: string[] }
) => boolean
This function is called for every console method supported by this utility.
If true
is returned, the message will not show in the console and the test won't fail.
Example:
failOnConsole({
silenceMessage: (errorMessage) => {
if (/Not implemented: navigation/.test(errorMessage)) {
return true
}
return false
},
})
skipTest
Use this if you want to ignore checks introduced by this library for specific tests determined by
the return of the callback function. Return false
if you do not want to skip console checks for
the specific test and return true
if you would like to skip it.
const ignoreList = [/.*components\/SomeComponent.test.tsx/]
const ignoreNameList = ['some component some test name']
failOnConsole({
skipTest: ({ testPath, testName }) => {
for (const pathExp of ignoreList) {
const result = pathExp.test(testPath)
if (result) return true
}
if (ignoreNameList.includes(testName)) {
return true
}
return false
},
})
shouldPrintMessage
Use this to print the message immediately when called not awaiting the test to finish. This is useful to show the message if there are
other or earlier test failures which will result in the fail on console error to be hidden by jest.
- Type:
boolean
- Default:
false
License
MIT
Maintainers
This project is maintained by Valentin Hervieu.
This project was originally part of @ricardo-ch organisation because I (Valentin) was working at Ricardo.
After leaving this company, they gracefully accepted to transfer the project to me. ❤️
Credits
Most of the logic is taken from React's setupTests file.