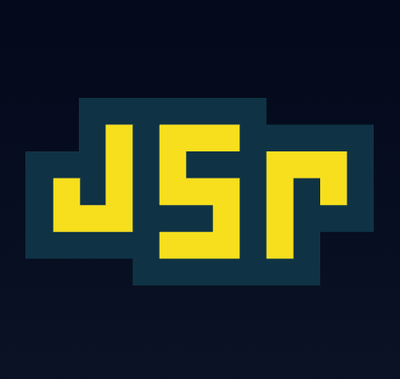
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
jest-junit
Advanced tools
The jest-junit npm package is a Jest reporter that creates compatible JUnit XML reports. It's primarily used in CI/CD pipelines to integrate test results with tools that consume JUnit XML format, such as Jenkins, CircleCI, and others. This allows for better visualization of test outcomes and more efficient debugging when tests fail.
Generating JUnit XML reports
This command runs Jest tests and generates a JUnit XML report. The '--reporters' option specifies that both the default reporter and jest-junit should be used, allowing for output in the console as well as the creation of an XML report.
jest --reporters=default --reporters=jest-junit
Customizing output location and file name
This example demonstrates how to customize the output directory and file name of the JUnit XML report using environment variables. The report will be saved to './artifacts/my_test_results.xml'.
JEST_JUNIT_OUTPUT_DIR='./artifacts' JEST_JUNIT_OUTPUT_NAME='my_test_results.xml' jest --reporters=default --reporters=jest-junit
Configuring through jest.config.js
This code snippet shows how to configure jest-junit through Jest's configuration file, jest.config.js. It specifies custom values for the suite name, output directory, and file name of the report.
module.exports = { reporters: ['default', ['jest-junit', { suiteName: 'My Suite', outputDirectory: './reports', outputName: 'results.xml' }]] };
Similar to jest-junit, mocha-junit-reporter is designed for Mocha tests. It generates JUnit XML reports for Mocha test suites. While jest-junit is tailored for Jest, mocha-junit-reporter offers similar functionality for projects using Mocha as their testing framework.
karma-junit-reporter is a plugin for the Karma test runner that outputs test results in JUnit XML format. It serves a similar purpose for Karma-based projects, providing integration with CI/CD tools that require JUnit XML reports. Compared to jest-junit, it's specific to the Karma ecosystem.
jasmine-reporters is a package that provides JUnit XML reporting for Jasmine, another popular testing framework. It includes a JUnit reporter among other reporter types. This package is analogous to jest-junit for projects that use Jasmine, enabling them to generate JUnit-compatible reports.
A Jest testResultsProcessor that creates compatible junit xml files
yarn add --dev jest-junit
In your jest config add the following entry:
{
"testResultsProcessor": "./node_modules/jest-junit"
}
Then simply run:
jest
jest-junit offers two configurations based on environment variables.
JEST_SUITE_NAME
: Default "jest tests"
JEST_JUNIT_OUTPUT
: Default "./junit.xml"
Example:
JEST_SUITE_NAME="Jest JUnit Unit Tests" JEST_JUNIT_OUTPUT="./artifacts/junit.xml" jest
Example output:
<testsuites name="Jest JUnit Unit Tests">
<testsuite name="My first suite" tests="1" errors="0" failures="0" skipped="0" timestamp="2016-11-19T01:37:20" time="0.105">
<testcase classname="My test case" name="My test case" time="6">
</testcase>
</testsuite>
</testsuites>
FAQs
A jest reporter that generates junit xml files
The npm package jest-junit receives a total of 3,974,907 weekly downloads. As such, jest-junit popularity was classified as popular.
We found that jest-junit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.