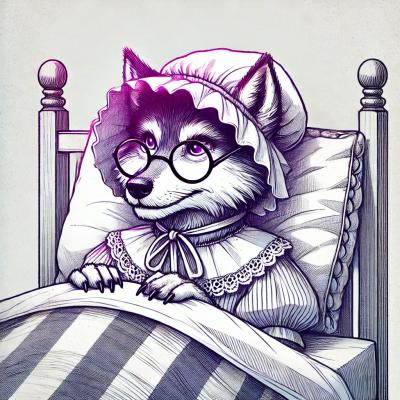
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
A simple JSON file store for node.js.
WARNING: Don't use it if you want to persist a large amount of objects. Use a real DB instead.
npm install jfs --save
var Store = require("jfs");
var db = new Store("data");
var d = {
foo: "bar"
};
// save with custom ID
db.save("anId", d, function(err){
// now the data is stored in the file data/anId.json
});
// save with generated ID
db.save(d, function(err, id){
// id is a unique ID
});
// save synchronously
var id = db.saveSync("anId", d);
db.get("anId", function(err, obj){
// obj = { foo: "bar" }
})
// pretty print file content
var prettyDB = new Store("data",{pretty:true});
var id = prettyDB.saveSync({foo:{bar:"baz"}});
// now the file content is formated in this way:
{
"foo": {
"bar": "baz"
}
}
// instead of this:
{"foo":{"bar":"baz"}}
// get synchronously
var obj = db.getSync("anId");
// get all available objects
db.all(function(err, objs){
// objs is a map: ID => OBJECT
});
// get all synchronously
var objs = db.allSync()
// delete by ID
db.delete("myId", function(err){
// the file data/myId.json was removed
});
// delete synchronously
db.delete("myId");
If you want to store all objects in a single file,
set the type
option to single
:
var db = new Store("data",{type:'single'});
or point to a JSON file:
var db = new Store("./path/to/data.json");
If you don't want to persist your data, you can set type
to memory
:
var db = new Store("data",{type:'memory'});
npm test
This project is licensed under the MIT License.
FAQs
A simple JSON file store
The npm package jfs receives a total of 4,795 weekly downloads. As such, jfs popularity was classified as popular.
We found that jfs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.