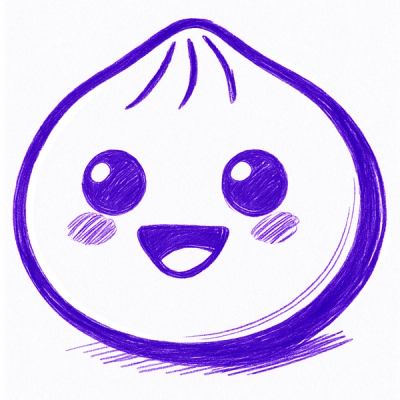
Security News
Bun 1.2.19 Adds Isolated Installs for Better Monorepo Support
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
The jmespath npm package is a query language for JSON, allowing you to extract and manipulate data from complex JSON structures with ease. It provides a powerful and expressive syntax for querying and transforming JSON data.
Searching JSON data
This feature allows you to search through JSON data using JMESPath expressions. In this example, it searches for names of locations in the state of WA.
"search(jsonData, 'locations[?state == \"WA\"].name')"
Projection
Projection is used to extract a list of values from a JSON structure. This code sample demonstrates how to get a list of all location names.
"search(jsonData, 'locations[*].name')"
Functions
JMESPath supports a variety of functions to manipulate and transform data. This example uses the `length` function to count the number of locations.
"search(jsonData, 'length(locations)')"
Lodash is a utility library that provides functions for common programming tasks, including data manipulation. While it doesn't use a query language like JMESPath, it offers a wide range of methods for manipulating objects and arrays, making it a versatile alternative.
JsonPath is another query language for JSON, similar to JMESPath. It allows you to extract parts of a JSON document using a query syntax. While both aim to simplify data extraction from JSON, their syntax and capabilities differ, with JMESPath generally offering a more expressive query language.
jmespath.js is a javascript implementation of JMESPath, which is a query language for JSON. It will take a JSON document and transform it into another JSON document through a JMESPath expression.
Using jmespath.js is really easy. There's a single function
you use, jmespath.search
:
> var jmespath = require('jmespath');
> jmespath.search({foo: {bar: {baz: [0, 1, 2, 3, 4]}}}, "foo.bar.baz[2]")
2
In the example we gave the search
function input data of
{foo: {bar: {baz: [0, 1, 2, 3, 4]}}}
as well as the JMESPath
expression foo.bar.baz[2]
, and the search
function evaluated
the expression against the input data to produce the result 2
.
The JMESPath language can do a lot more than select an element from a list. Here are a few more examples:
> jmespath.search({foo: {bar: {baz: [0, 1, 2, 3, 4]}}}, "foo.bar")
{ baz: [ 0, 1, 2, 3, 4 ] }
> jmespath.search({"foo": [{"first": "a", "last": "b"},
{"first": "c", "last": "d"}]},
"foo[*].first")
[ 'a', 'c' ]
> jmespath.search({"foo": [{"age": 20}, {"age": 25},
{"age": 30}, {"age": 35},
{"age": 40}]},
"foo[?age > `30`]")
[ { age: 35 }, { age: 40 } ]
The example above only show a small amount of what a JMESPath expression can do. If you want to take a tour of the language, the best place to go is the JMESPath Tutorial.
One of the best things about JMESPath is that it is implemented in many different programming languages including python, ruby, php, lua, etc. To see a complete list of libraries, check out the JMESPath libraries page.
And finally, the full JMESPath specification can be found on the JMESPath site.
FAQs
JMESPath implementation in javascript
The npm package jmespath receives a total of 6,585,687 weekly downloads. As such, jmespath popularity was classified as popular.
We found that jmespath demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Bun 1.2.19 introduces isolated installs for smoother monorepo workflows, along with performance boosts, new tooling, and key compatibility fixes.
Security News
Popular npm packages like eslint-config-prettier were compromised after a phishing attack stole a maintainer’s token, spreading malicious updates.
Security News
/Research
A phishing attack targeted developers using a typosquatted npm domain (npnjs.com) to steal credentials via fake login pages - watch out for similar scams.