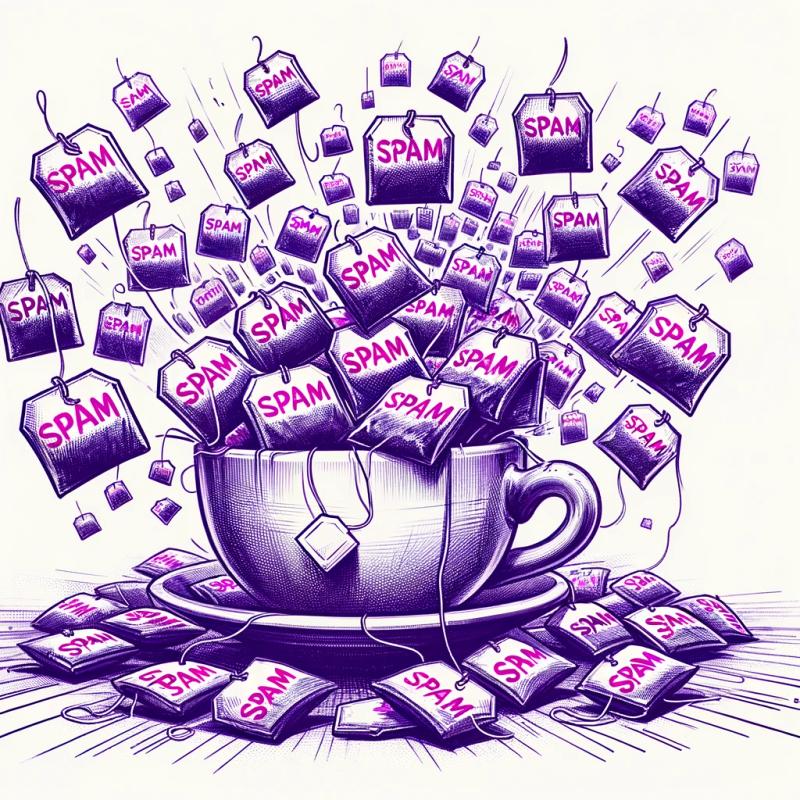
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
joi-fhir
Advanced tools
Readme
Node.js utility for validating FHIR resources.
joi-fhir
on NPM (Only for deployment)npm install --save joi-fhir
const validateFhir = require('joi-fhir');
const Encounter = {
resourceType: 'Encounter',
id: 'ENC001',
...
}
// Validate any generic FHIR resource
validateFhir(Encounter)
.then((validated) => console.log('Validated encounter', validated))
.catch((error) => console.log('Error validating encounter', error));
// Ensure FHIR resource matches a certain type
validateFhir(Encounter, { resourceType: 'Encounter' })
.then((validated) => console.log('Validated encounter', validated))
.catch((error) => console.log('Error validating encounter', error));
This module exports a single Function
that accepts a FHIR resource and an options
object. The function validates the FHIR resource matches the spec and returns a promise with either the validated/formatted FHIR resource or an error detailing the malformed data.
validateFhir(resource);
Parameter | Type | Description |
---|---|---|
resource | Object | A FHIR resource |
options
Parameter | Type | Description | Default |
---|---|---|---|
resourceType | String | If provided, ensure the resource is of this type. Otherwise, allow any FHIR resource | none |
encounterA
Data about an encounter.
{
"resourceType": "Encounter",
"status": "in-progress",
"subject": {
"reference": "Patient/P01"
},
"reason": [
{
"text": "Laceration to leg"
}
]
}
const validateFhir = require('@agilemd/joi-fhir');
return validateFhir(encounter)
.then((validated) => {
...
})
.catch((err) => {
// Handle error
})
validated/formatted Encounter
{
"id": "31a49ff9-2d10-481c-8720-a1a3e61fa981",
"resourceType": "Encounter",
"status": "in-progress",
"subject": {
"reference": "Patient/P01"
},
"reason": [
{
"text": "Laceration to leg"
}
]
}
Clone the source repository, cd
into the joi-fhir
directory, and install dependencies:
git clone git@github.com:agilemd/joi-fhir.git
cd joi-fhir
npm install
To run the unit tests:
npm test
Changes must not reduce coverage of statements, branches, and functions. To determine unit test coverage:
npm run coverage
The debug module is used for runtime logging. Omit the DEBUG
environment variable to squelch all logging. Set DEBUG
to the desired level (e.g. DEBUG=@agilemd/joi-fhir:SUBMODULE
) to restrict logging to a desired service. Or, use DEBUG=*
to get all debug output from everywhere, including dependencies.
DEBUG=@agilemd/joi-fhir* npm test
To enable a git hook that runs npm test
prior to pushing, cd
into the project repo and run:
touch .git/hooks/pre-push
chmod +x .git/hooks/pre-push
echo "npm test" > .git/hooks/pre-push
This project follows semantic versioning. After committing the latest code to GitHub master, update the version:
npm version [major/minor/patch]
Then push the tag to GitHub and publish this package to npm:
git push origin --tags
npm publish
This project is a work in progress. Any defined FHIR resource will pass validation; however, only certain resources are fully validated. The table below describes which resources have complete validation and which are in progress
Support legend
Icon | Description |
---|---|
:white_check_mark: | Completely implemented |
:o: | Partially implemented |
:no_entry_sign: | Not yet implemented |
Resource Type | Support |
---|---|
Account | :no_entry_sign: |
ActivityDefinition | :no_entry_sign: |
AllergyIntolerance | :no_entry_sign: |
AdverseEvent | :no_entry_sign: |
Appointment | :no_entry_sign: |
AppointmentResponse | :no_entry_sign: |
AuditEvent | :no_entry_sign: |
Basic | :no_entry_sign: |
Binary | :no_entry_sign: |
BodySite | :no_entry_sign: |
Bundle | :no_entry_sign: |
CapabilityStatement | :no_entry_sign: |
CarePlan | :no_entry_sign: |
CareTeam | :no_entry_sign: |
ChargeItem | :no_entry_sign: |
Claim | :no_entry_sign: |
ClaimResponse | :no_entry_sign: |
ClinicalImpression | :no_entry_sign: |
CodeSystem | :no_entry_sign: |
Communication | :no_entry_sign: |
CommunicationRequest | :no_entry_sign: |
CompartmentDefinition | :no_entry_sign: |
Composition | :no_entry_sign: |
ConceptMap | :no_entry_sign: |
Condition | :no_entry_sign: |
Consent | :no_entry_sign: |
Contract | :no_entry_sign: |
Coverage | :no_entry_sign: |
DataElement | :no_entry_sign: |
DetectedIssue | :no_entry_sign: |
Device | :no_entry_sign: |
DeviceComponent | :no_entry_sign: |
DeviceMetric | :no_entry_sign: |
DeviceRequest | :no_entry_sign: |
DeviceUseStatement | :no_entry_sign: |
DiagnosticReport | :no_entry_sign: |
DocumentManifest | :no_entry_sign: |
DocumentReference | :no_entry_sign: |
EligibilityRequest | :no_entry_sign: |
EligibilityResponse | :no_entry_sign: |
Encounter | :no_entry_sign: |
Endpoint | :no_entry_sign: |
EnrollmentRequest | :no_entry_sign: |
EnrollmentResponse | :no_entry_sign: |
EpisodeOfCare | :no_entry_sign: |
ExpansionProfile | :no_entry_sign: |
ExplanationOfBenefit | :no_entry_sign: |
FamilyMemberHistory | :no_entry_sign: |
Flag | :no_entry_sign: |
Goal | :no_entry_sign: |
GraphDefinition | :no_entry_sign: |
Group | :no_entry_sign: |
GuidanceResponse | :no_entry_sign: |
HealthcareService | :no_entry_sign: |
ImagingManifest | :no_entry_sign: |
ImagingStudy | :no_entry_sign: |
Immunization | :no_entry_sign: |
ImmunizationRecommendation | :no_entry_sign: |
ImplementationGuide | :no_entry_sign: |
Library | :no_entry_sign: |
Linkage | :no_entry_sign: |
List | :no_entry_sign: |
Location | :no_entry_sign: |
Measure | :no_entry_sign: |
MeasureReport | :no_entry_sign: |
Media | :no_entry_sign: |
Medication | :no_entry_sign: |
MedicationAdministration | :no_entry_sign: |
MedicationDispense | :no_entry_sign: |
MedicationStatement | :no_entry_sign: |
MessageDefinition | :no_entry_sign: |
MessageHeader | :no_entry_sign: |
NamingSystem | :no_entry_sign: |
NutritionOrder | :no_entry_sign: |
Observation | :no_entry_sign: |
OperationDefinition | :no_entry_sign: |
OperationOutcome | :no_entry_sign: |
Organization | :no_entry_sign: |
Parameters | :no_entry_sign: |
Patient | :no_entry_sign: |
PaymentNotice | :no_entry_sign: |
PaymentReconciliation | :no_entry_sign: |
Person | :no_entry_sign: |
PlanDefinition | :no_entry_sign: |
Practitioner | :no_entry_sign: |
PractitionerRole | :no_entry_sign: |
Procedure | :no_entry_sign: |
ProcedureRequest | :no_entry_sign: |
ProcessRequest | :no_entry_sign: |
ProcessResponse | :no_entry_sign: |
Provenance | :no_entry_sign: |
Questionnaire | :no_entry_sign: |
QuestionnaireResponse | :no_entry_sign: |
ReferralRequest | :no_entry_sign: |
RelatedPerson | :no_entry_sign: |
RequestGroup | :no_entry_sign: |
ResearchStudy | :no_entry_sign: |
ResearchSubject | :no_entry_sign: |
RiskAssessment | :no_entry_sign: |
Schedule | :no_entry_sign: |
SearchParameter | :no_entry_sign: |
Sequence | :no_entry_sign: |
ServiceDefinition | :no_entry_sign: |
Slot | :no_entry_sign: |
Specimen | :no_entry_sign: |
StructureDefinition | :no_entry_sign: |
StructureMap | :no_entry_sign: |
Subscription | :no_entry_sign: |
Substance | :no_entry_sign: |
SupplyDelivery | :no_entry_sign: |
SupplyRequest | :no_entry_sign: |
Task | :no_entry_sign: |
TestScript | :no_entry_sign: |
TestReport | :no_entry_sign: |
ValueSet | :no_entry_sign: |
VisionPrescription | :no_entry_sign: |
FAQs
Validate FHIR data with Joi
The npm package joi-fhir receives a total of 2 weekly downloads. As such, joi-fhir popularity was classified as not popular.
We found that joi-fhir demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.