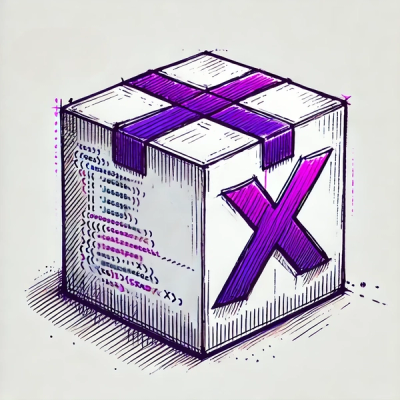
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
jordalgo-task
Advanced tools
A javascript data type for async requests. Very similar to the data.task and fun-task with some modifications. Published as jordalgo-task
npm install jordalgo-task
import Task from 'Task';
const task = new Task((sendFail, sendSuccess) => {
const id = setTimeout(() => {
sendSuccess(1);
}, 1000);
return () => { clearTimeout(id); };
});
const cancel = task.run(
fail => {
// never called;
},
success => {
// success === 1
}
);
run
is invoked.function getUser(id) {
return new Task((sendFail, sendSuccess) => {
// AJAX request to get a user with id
sendSuccess({ user });
});
}
function getFollowers(username) {
return new Task((sendFail, sendSuccess) => {
// AJAX request using username
success([followers]);
});
}
getUser()
.map(user => user.name)
.chain(getFollowers)
.run(
fail => {},
success => {
// success === [followers] (if all went well)
}
});
A promise-like feature that allows you to hang on to values already processed within a Task. Computations don't get re-run.
var count = 0;
const task = new Task((sendFail, sendSuccess) => {
const id = setTimeout(() => {
sendSuccess(++count);
}, 1000);
return () => { clearTimeout(id); };
});
const taskOnce = task.memoize();
taskOnce.run(
fail => {},
success => {
// success === 1
// count === 1
}
});
taskOnce.run(
fail => {},
success => {
// success === 1
// count === 1
}
});
Parallelize multiple Tasks. Returns an array of successs. If one of the Tasks sends a fail then the cancelation functions for all other Tasks (not yet completed) will be called.
var count = 0;
function createTask(to) {
var order = ++count;
return new Task(sendFail, sendSuccess => {
var id = setTimeout(() => {
sendSuccess(order);
}, to);
return () => {
clearTimeout(id);
};
});
}
Task.all([
createTask(100),
createTask(500),
createTask(0)
]).run(
fail => {},
success => {
// count === 3
// success === [3, 1, 2]
}
});
Task is compatible with Fantasy Land and Static Land implementing:
run
on an Task.A lot of code was inspired and stolen directly from data.task (Quildreen Motta) and fun-task (Roman Pominov).
FAQs
A javascript monad for dealing with async tasks
We found that jordalgo-task demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.