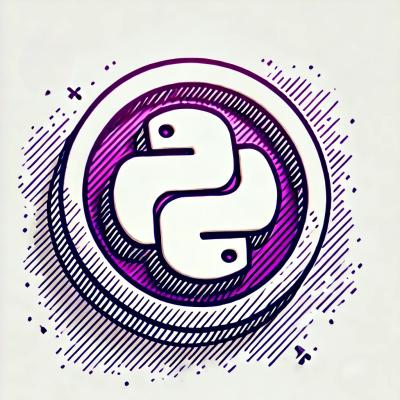
Security News
Introducing the Socket Python SDK
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
'JSON Web Almost Everything' - JWA, JWS, JWE, JWT, JWK, JWKS for Node.js, Browser, Cloudflare Workers, Deno, and other Web-interoperable runtimes
The 'jose' npm package is a JavaScript library that provides a variety of cryptographic operations based on JSON Web Tokens (JWT), JSON Web Encryption (JWE), JSON Web Signature (JWS), and JSON Web Key (JWK). It is designed to be compliant with the JOSE (JSON Object Signing and Encryption) suite of standards, and it supports various algorithms for encryption, decryption, signing, and verification of tokens.
JWT Signing
This code sample demonstrates how to create and sign a JWT with a specified algorithm and secret.
const { SignJWT } = require('jose');
const jwt = await new SignJWT({ 'urn:example:claim': true })
.setProtectedHeader({ alg: 'HS256' })
.setIssuedAt()
.setIssuer('urn:example:issuer')
.setAudience('urn:example:audience')
.setExpirationTime('2h')
.sign(new TextEncoder().encode('your-256-bit-secret'));
console.log(jwt);
JWT Verification
This code sample shows how to verify a JWT using a secret key and retrieve the payload and protected header.
const { jwtVerify } = require('jose');
const { payload, protectedHeader } = await jwtVerify(jwt, new TextEncoder().encode('your-256-bit-secret'));
console.log(payload);
console.log(protectedHeader);
JWE Encryption
This code sample illustrates how to encrypt a JWT using RSA-OAEP-256 for key management and A256GCM for content encryption.
const { EncryptJWT } = require('jose');
const jwe = await new EncryptJWT({ 'urn:example:claim': true })
.setProtectedHeader({ alg: 'RSA-OAEP-256', enc: 'A256GCM' })
.setIssuedAt()
.setIssuer('urn:example:issuer')
.setAudience('urn:example:audience')
.setExpirationTime('2h')
.encrypt(publicKey);
console.log(jwe);
JWE Decryption
This code sample demonstrates how to decrypt a JWE and obtain the payload and protected header using a private key.
const { jwtDecrypt } = require('jose');
const { payload, protectedHeader } = await jwtDecrypt(jwe, privateKey);
console.log(payload);
console.log(protectedHeader);
JWK Key Generation
This code sample shows how to generate a public and private key pair for the RS256 algorithm.
const { generateKeyPair } = require('jose');
const { publicKey, privateKey } = await generateKeyPair('RS256');
console.log(publicKey);
console.log(privateKey);
The 'jsonwebtoken' package is similar to 'jose' in that it provides functionality for creating and verifying JWTs. It is widely used and has a simpler API for basic JWT operations, but it does not support JWE or more advanced JOSE features.
The 'node-jose' package is another alternative that implements the JOSE suite of standards. It offers similar functionalities to 'jose' but has a different API design and may have different performance characteristics.
The 'jws' package is focused on JSON Web Signatures (JWS). It is a simpler library that allows signing and verifying messages but does not handle JWTs, JWEs, or JWKs directly.
"JSON Web Almost Everything" - JWA, JWS, JWE, JWT, JWK, JWKS for Node.js, Browser, Cloudflare Workers, Deno, and other Web-interoperable runtimes.
The following specifications are implemented by jose
The test suite utilizes examples defined in RFC7520 to confirm its JOSE implementation is correct.
example
ESM import
import * as jose from 'jose'
example
CJS require
const jose = require('jose')
example
Deno import
import * as jose from 'https://deno.land/x/jose/index.ts'
Version | Security Fixes 🔑 | Other Bug Fixes 🐞 | New Features ⭐ |
---|---|---|---|
v4.x | ✅ | ✅ | ✅ |
v3.x, v2.x, v1.x | ✅ | ❌ | ❌ |
Yes. All module's public API is subject to Semantic Versioning 2.0.0.
jws
, jwa
or jsonwebtoken
?node-jose
?node-jose
is built to work in any javascript runtime, to be able to do that it packs a lot of
polyfills and javascript implementation code in the form of
node-forge
, this significantly increases the footprint
of the modules with dependencies that either aren't ever used or have native implementation available
in the runtime already, those are often times faster and more reliable.
Uint8Array
is a valid input, so is Buffer
since buffers are instances of Uint8Array.Uint8Array
is returned and you want a Buffer
instead, use Buffer.from(uint8array)
.Yes the bundle size is on the larger side, that is because each module is actually published multiple times so that it can remain truly without dependencies and be universal / isomorphic.
Nevertheless, since each module can be required independently and is fully tree-shakeable, the install size should not be a cause for concern.
FAQs
JWA, JWS, JWE, JWT, JWK, JWKS for Node.js, Browser, Cloudflare Workers, Deno, Bun, and other Web-interoperable runtimes
The npm package jose receives a total of 8,860,081 weekly downloads. As such, jose popularity was classified as popular.
We found that jose demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The initial version of the Socket Python SDK is now on PyPI, enabling developers to more easily interact with the Socket REST API in Python projects.
Security News
Floating dependency ranges in npm can introduce instability and security risks into your project by allowing unverified or incompatible versions to be installed automatically, leading to unpredictable behavior and potential conflicts.
Security News
A new Rust RFC proposes "Trusted Publishing" for Crates.io, introducing short-lived access tokens via OIDC to improve security and reduce risks associated with long-lived API tokens.