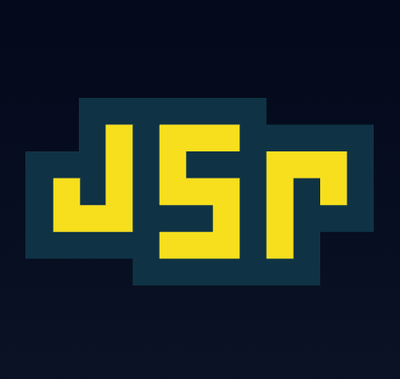
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Jotai is a state management library for React that focuses on atomic state management. It allows you to manage state in a more granular and efficient way by breaking down the state into smaller, independent atoms.
Basic Atom
This feature allows you to create a basic atom and use it in a React component. The `atom` function creates a new atom with an initial state, and the `useAtom` hook allows you to read and update the atom's state.
import { atom, useAtom } from 'jotai';
const countAtom = atom(0);
function Counter() {
const [count, setCount] = useAtom(countAtom);
return (
<div>
<p>{count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
Derived Atom
This feature allows you to create a derived atom that depends on the state of another atom. The `doubledCountAtom` is derived from `countAtom` and always holds double the value of `countAtom`.
import { atom, useAtom } from 'jotai';
const countAtom = atom(0);
const doubledCountAtom = atom((get) => get(countAtom) * 2);
function DoubledCounter() {
const [doubledCount] = useAtom(doubledCountAtom);
return <p>{doubledCount}</p>;
}
Async Atom
This feature allows you to create an atom that handles asynchronous operations. The `fetchUserAtom` fetches user data from an API and the `User` component displays the user's name once the data is loaded.
import { atom, useAtom } from 'jotai';
const fetchUserAtom = atom(async () => {
const response = await fetch('https://jsonplaceholder.typicode.com/users/1');
return response.json();
});
function User() {
const [user] = useAtom(fetchUserAtom);
return user ? <p>{user.name}</p> : <p>Loading...</p>;
}
Recoil is another state management library for React that provides a similar atomic state management approach. It allows you to create atoms and selectors to manage and derive state. Compared to Jotai, Recoil offers more built-in features like selectors and persistence, but Jotai is simpler and more lightweight.
Zustand is a small, fast, and scalable state management library for React. It uses a different approach by creating a global store with hooks to access and update the state. While Jotai focuses on atomic state management, Zustand provides a more traditional global state management solution.
Redux is a widely-used state management library for JavaScript applications. It uses a single global store and actions to manage state changes. Compared to Jotai, Redux is more complex and requires more boilerplate code, but it offers a robust and scalable solution for large applications.
visit jotai.org or npm i jotai
Jotai scales from a simple useState replacement to an enterprise TypeScript application.
An atom represents a piece of state. All you need is to specify an initial value, which can be primitive values like strings and numbers, objects, and arrays. You can create as many primitive atoms as you want.
import { atom } from 'jotai'
const countAtom = atom(0)
const countryAtom = atom('Japan')
const citiesAtom = atom(['Tokyo', 'Kyoto', 'Osaka'])
const mangaAtom = atom({ 'Dragon Ball': 1984, 'One Piece': 1997, Naruto: 1999 })
It can be used like React.useState
:
import { useAtom } from 'jotai'
function Counter() {
const [count, setCount] = useAtom(countAtom)
return (
<h1>
{count}
<button onClick={() => setCount((c) => c + 1)}>one up</button>
...
A new read-only atom can be created from existing atoms by passing a read
function as the first argument. get
allows you to fetch the contextual value
of any atom.
const doubledCountAtom = atom((get) => get(countAtom) * 2)
function DoubleCounter() {
const [doubledCount] = useAtom(doubledCountAtom)
return <h2>{doubledCount}</h2>
}
You can combine multiple atoms to create a derived atom.
const count1 = atom(1)
const count2 = atom(2)
const count3 = atom(3)
const sum = atom((get) => get(count1) + get(count2) + get(count3))
Or if you like fp patterns ...
const atoms = [count1, count2, count3, ...otherAtoms]
const sum = atom((get) => atoms.map(get).reduce((acc, count) => acc + count))
You can make the read function an async function too.
const urlAtom = atom('https://json.host.com')
const fetchUrlAtom = atom(async (get) => {
const response = await fetch(get(urlAtom))
return await response.json()
})
function Status() {
// Re-renders the component after urlAtom is changed and the async function above concludes
const [json] = useAtom(fetchUrlAtom)
...
Specify a write function at the second argument. get
will return the current
value of an atom. set
will update the value of an atom.
const decrementCountAtom = atom(
(get) => get(countAtom),
(get, set, _arg) => set(countAtom, get(countAtom) - 1)
)
function Counter() {
const [count, decrement] = useAtom(decrementCountAtom)
return (
<h1>
{count}
<button onClick={decrement}>Decrease</button>
...
Just do not define a read function.
const multiplyCountAtom = atom(null, (get, set, by) =>
set(countAtom, get(countAtom) * by),
)
function Controls() {
const [, multiply] = useAtom(multiplyCountAtom)
return <button onClick={() => multiply(3)}>triple</button>
}
Just make the write function an async function and call set
when you're ready.
const fetchCountAtom = atom(
(get) => get(countAtom),
async (_get, set, url) => {
const response = await fetch(url)
set(countAtom, (await response.json()).count)
}
)
function Controls() {
const [count, compute] = useAtom(fetchCountAtom)
return (
<button onClick={() => compute('http://count.host.com')}>compute</button>
...
FAQs
👻 Primitive and flexible state management for React
The npm package jotai receives a total of 947,994 weekly downloads. As such, jotai popularity was classified as popular.
We found that jotai demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.