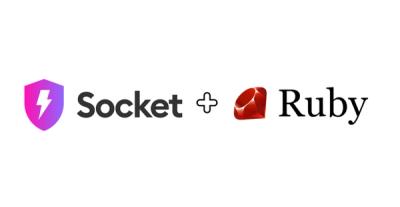
Product
Introducing Ruby Support in Socket
Socket is launching Ruby support for all users. Enhance your Rails projects with AI-powered security scans for vulnerabilities and supply chain threats. Now in Beta!
A simple SHA-256 / SHA-224 hash function for JavaScript supports UTF-8 encoding.
The js-sha256 npm package is a simple SHA-256 & SHA-224 hash function for JavaScript supports UTF-8 encoding. It can be used in various environments like web browsers and Node.js. It provides functionalities for hashing messages with SHA-256 and SHA-224 algorithms.
SHA-256 Hash
Generates a SHA-256 hash of the input string. This is useful for creating unique identifiers for data, secure password storage, and ensuring data integrity.
sha256('message')
SHA-224 Hash
Generates a SHA-224 hash of the input string. Similar to SHA-256, but produces a shorter hash value. It's used for applications where hash length is a concern but still requires a high level of security.
sha224('message')
HMAC-SHA-256
Generates a HMAC (Hash-based Message Authentication Code) using SHA-256. This is used for verifying both the data integrity and the authenticity of a message.
sha256.hmac('key', 'message')
HMAC-SHA-224
Generates a HMAC using SHA-224. Similar to HMAC-SHA-256, it's used for data integrity and authenticity but with a shorter hash value.
sha224.hmac('key', 'message')
Crypto-js is a package that provides cryptographic functionalities including SHA-256. It offers a broader range of cryptographic algorithms compared to js-sha256, making it suitable for applications requiring more than just SHA-256 or SHA-224.
Sha.js is a streaming SHA hashes module for Node.js that supports SHA-1, SHA-224, SHA-256, SHA-384, and SHA-512. It's more versatile than js-sha256 in terms of the variety of SHA algorithms supported.
A simple SHA-256 / SHA-224 hash function for JavaScript supports UTF-8 encoding.
SHA256 Online
SHA224 Online
SHA256 File Online
SHA225 File Online
You can also install js-sha256 by using Bower.
bower install js-sha256
For node.js, you can use this command to install:
npm install js-sha256
You could use like this:
sha256('Message to hash');
sha224('Message to hash');
var hash = sha256.create();
hash.update('Message to hash');
hash.hex();
var hash2 = sha256.update('Message to hash');
hash2.update('Message2 to hash');
hash2.array();
// HMAC
sha256.hmac('key', 'Message to hash');
sha224.hmac('key', 'Message to hash');
var hash = sha256.hmac.create('key');
hash.update('Message to hash');
hash.hex();
var hash2 = sha256.hmac.update('key', 'Message to hash');
hash2.update('Message2 to hash');
hash2.array();
If you use node.js, you should require the module first:
var sha256 = require('js-sha256');
or
var sha256 = require('js-sha256').sha256;
var sha224 = require('js-sha256').sha224;
or
const { sha256, sha224 } = require('js-sha256');
If you use TypeScript, you can import like this:
import { sha256, sha224 } from 'js-sha256';
It supports AMD:
require(['your/path/sha256.js'], function(sha256) {
// ...
});
sha256(''); // e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855
sha256('The quick brown fox jumps over the lazy dog'); // d7a8fbb307d7809469ca9abcb0082e4f8d5651e46d3cdb762d02d0bf37c9e592
sha256('The quick brown fox jumps over the lazy dog.'); // ef537f25c895bfa782526529a9b63d97aa631564d5d789c2b765448c8635fb6c
sha224(''); // d14a028c2a3a2bc9476102bb288234c415a2b01f828ea62ac5b3e42f
sha224('The quick brown fox jumps over the lazy dog'); // 730e109bd7a8a32b1cb9d9a09aa2325d2430587ddbc0c38bad911525
sha224('The quick brown fox jumps over the lazy dog.'); // 619cba8e8e05826e9b8c519c0a5c68f4fb653e8a3d8aa04bb2c8cd4c
// It also supports UTF-8 encoding
sha256('中文'); // 72726d8818f693066ceb69afa364218b692e62ea92b385782363780f47529c21
sha224('中文'); // dfbab71afdf54388af4d55f8bd3de8c9b15e0eb916bf9125f4a959d4
// It also supports byte `Array`, `Uint8Array`, `ArrayBuffer` input
sha256([]); // e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855
sha256(new Uint8Array([211, 212])); // 182889f925ae4e5cc37118ded6ed87f7bdc7cab5ec5e78faef2e50048999473f
// Different output
sha256(''); // e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855
sha256.hex(''); // e3b0c44298fc1c149afbf4c8996fb92427ae41e4649b934ca495991b7852b855
sha256.array(''); // [227, 176, 196, 66, 152, 252, 28, 20, 154, 251, 244, 200, 153, 111, 185, 36, 39, 174, 65, 228, 100, 155, 147, 76, 164, 149, 153, 27, 120, 82, 184, 85]
sha256.digest(''); // [227, 176, 196, 66, 152, 252, 28, 20, 154, 251, 244, 200, 153, 111, 185, 36, 39, 174, 65, 228, 100, 155, 147, 76, 164, 149, 153, 27, 120, 82, 184, 85]
sha256.arrayBuffer(''); // ArrayBuffer
The project is released under the MIT license.
The project's website is located at https://github.com/emn178/js-sha256
Author: Chen, Yi-Cyuan (emn178@gmail.com)
v0.11.0 / 2024-01-24
FAQs
A simple SHA-256 / SHA-224 hash function for JavaScript supports UTF-8 encoding.
The npm package js-sha256 receives a total of 1,232,577 weekly downloads. As such, js-sha256 popularity was classified as popular.
We found that js-sha256 demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket is launching Ruby support for all users. Enhance your Rails projects with AI-powered security scans for vulnerabilities and supply chain threats. Now in Beta!
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.