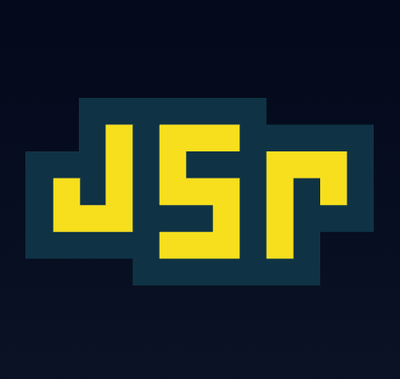
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
JSCS — JavaScript Code Style.
jscs
is a code style checker. jscs
can check cases, which are not implemeted in jshint,
but it does not duplicate jshint
functionality, so you should use jscs
and jshint
together.
jscs
can be installed using npm
:
npm install jscs
To run jscs
, you can use the following command from the project root:
./node_modules/.bin/jscs path[ path[...]]
jscs
is configured using .jscs.json
file, located in the project root.
Example configuration:
{
/*
Option: requireCurlyBraces
Requires curly braces after statements.
Valid example:
if (x) {
x++;
}
Invalid example:
if (x) x++;
*/
"requireCurlyBraces": ["if", "else", "for", "while", "do"],
/*
Option: requireSpaceAfterKeywords
Requires space after keyword.
Valid example:
return true;
Invalid example:
if(x) {
x++;
}
*/
"requireSpaceAfterKeywords": ["if", "else", "for", "while", "do", "switch", "return"],
/*
Option: disallowSpaceAfterKeywords
Disallows space after keyword.
Valid example:
if(x > y) {
y++;
}
*/
"disallowSpaceAfterKeywords": ["if", "else", "for", "while", "do", "switch"],
/*
Option: disallowMultipleVarDecl
Disallows multiple var declaration.
Valid example:
var x = 1;
var y = 2;
Invalid example:
var x = 1,
y = 2;
*/
"disallowMultipleVarDecl": true,
/*
Option: requireMultipleVarDecl
Requires multiple var declaration.
Valid example:
var x = 1,
y = 2;
Invalid example:
var x = 1;
var y = 2;
*/
"requireMultipleVarDecl": true,
/*
Option: disallowSpacesInsideObjectBrackets
Disallows space after opening object curly brace and before closing.
Valid example:
var x = {a: 1};
Invalid example:
var x = { a: 1 };
*/
"disallowSpacesInsideObjectBrackets": true,
/*
Option: disallowSpacesInsideArrayBrackets
Disallows space after opening array square brace and before closing.
Valid example:
var x = [a: 1];
Invalid example:
var x = [ a: 1 ];
*/
"disallowSpacesInsideArrayBrackets": true,
/*
Option: requireSpacesInsideObjectBrackets
Possible values: "all" for strict mode, "allButNested" ignores closing brackets in a row.
Requires space after opening object curly brace and before closing.
Valid example for mode "all":
var x = { a: { b: 1 } };
Valid example for mode "allButNested":
var x = { a: { b: 1 }};
Invalid example:
var x = {a: 1};
*/
"requireSpacesInsideObjectBrackets": "all",
/*
Option: disallowQuotedKeysInObjects
Disallows quoted keys in object if possible.
Valid example:
var x = {a: 1};
Invalid example:
var x = {'a': 1};
*/
"disallowQuotedKeysInObjects": true,
/*
Option: disallowSpaceAfterObjectKeys
Disallows space after object keys.
Valid example:
var x = {a: 1};
Invalid example:
var x = {a : 1};
*/
"disallowSpaceAfterObjectKeys": true,
/*
Option: requireSpaceAfterObjectKeys
Requires space after object keys.
Valid example:
var x = {a : 1};
Invalid example:
var x = {a: 1};
*/
"requireSpaceAfterObjectKeys": true,
/*
Option: requireAlignedObjectValues
Possible values:
"all" for strict mode,
"skipWithFunction" ignores objects if one of the property values is a function expression,
"skipWithLineBreak" ignores objects if there are line breaks between properties
Requires proper alignment in object literals.
Valid example:
var x = {
a : 1,
bcd : 2,
ef : 'str'
};
Invalid example:
var x = {
a : 1,
bcd : 2,
ef : 'str'
};
*/
"requireAlignedObjectValues": "all",
/*
Option: disallowLeftStickedOperators
Disallows sticking operators to the left.
Valid example:
x = y ? 1 : 2;
Invalid example:
x = y? 1 : 2;
*/
"disallowLeftStickedOperators": ["?", "+", "-", "/", "*", "=", "==", "===", "!=", "!==", ">", ">=", "<", "<="],
/*
Option: requireRightStickedOperators
Requires sticking operators to the right.
Valid example:
x = !y;
Invalid example:
x = ! y;
*/
"requireRightStickedOperators": ["!"],
/*
Option: disallowRightStickedOperators
Disallows sticking operators to the right.
Valid example:
x = y + 1;
Invalid example:
x = y +1;
*/
"disallowRightStickedOperators": ["?", "+", "/", "*", ":", "=", "==", "===", "!=", "!==", ">", ">=", "<", "<="],
/*
Option: requireLeftStickedOperators
Requires sticking operators to the left.
Valid example:
x = [1, 2];
Invalid example:
x = [1 , 2];
*/
"requireLeftStickedOperators": [","],
/*
Option: disallowImplicitTypeConversion
Disallows implicit type conversion.
Valid example:
x = Boolean(y);
x = Number(y);
x = String(y);
x = s.indexOf('.') !== -1;
Invalid example:
x = !!y;
x = +y;
x = '' + y;
x = ~s.indexOf('.');
*/
"disallowImplicitTypeConversion": ["numeric", "boolean", "binary", "string"],
/*
Option: disallowKeywords
Disallows usage of specified keywords.
Invalid example:
with (x) {
prop++;
}
*/
"disallowKeywords": ["with"],
/*
Option: disallowMultipleLineBreaks
Disallows multiple blank lines in a row.
Invalid example:
var x = 1;
x++;
*/
"disallowMultipleLineBreaks": true,
/*
Option: disallowKeywordsOnNewLine
Disallows placing keywords on a new line.
Valid example:
if (x < 0) {
x++;
} else {
x--;
}
Invalid example:
if (x < 0) {
x++;
}
else {
x--;
}
*/
"disallowKeywordsOnNewLine": ["else"],
/*
Option: requireKeywordsOnNewLine
Requires placing keywords on a new line.
Valid example:
if (x < 0) {
x++;
}
else {
x--;
}
Invalid example:
if (x < 0) {
x++;
} else {
x--;
}
*/
"requireKeywordsOnNewLine": ["else"],
/*
Option: requireLineFeedAtFileEnd
Requires placing line feed at file end.
*/
"requireLineFeedAtFileEnd": true,
/*
Option: validateJSDoc
Enables jsdoc validation.
Option: validateJSDoc.checkParamNames
Ensures param names in jsdoc and in function declaration are equal.
Option: validateJSDoc.requireParamTypes
Ensures params in jsdoc contains type.
Option: validateJSDoc.checkRedundantParams
Reports redundant params in jsdoc.
*/
"validateJSDoc": {
"checkParamNames": true,
"checkRedundantParams": true,
"requireParamTypes": true
},
/*
Option: excludeFiles
Disables style checking for specified paths.
*/
"excludeFiles": ["node_modules/**"]
}
Version 1.0.1:
disallowQuotedKeysInObjects
.FAQs
JavaScript Code Style
The npm package jscs receives a total of 43,399 weekly downloads. As such, jscs popularity was classified as popular.
We found that jscs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.