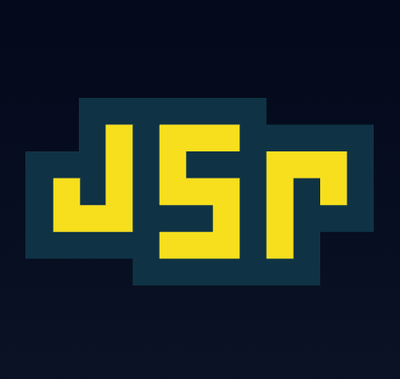
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
jsftp is a lightweight FTP client for Node.js that allows you to interact with FTP servers. It supports a variety of FTP operations such as uploading, downloading, renaming, and deleting files, as well as creating and removing directories.
Connecting to an FTP server
This code demonstrates how to connect to an FTP server using jsftp. You need to provide the host, username, and password for the FTP server.
const JSFtp = require('jsftp');
const ftp = new JSFtp({
host: 'ftp.example.com',
user: 'username',
pass: 'password'
});
ftp.auth('username', 'password', (err, res) => {
if (err) return console.error(err);
console.log('Connected to FTP server');
});
Uploading a file
This code demonstrates how to upload a file from the local filesystem to the FTP server using jsftp.
ftp.put('local/path/to/file.txt', 'remote/path/to/file.txt', err => {
if (err) return console.error(err);
console.log('File uploaded successfully');
});
Downloading a file
This code demonstrates how to download a file from the FTP server to the local filesystem using jsftp.
ftp.get('remote/path/to/file.txt', 'local/path/to/file.txt', err => {
if (err) return console.error(err);
console.log('File downloaded successfully');
});
Listing files in a directory
This code demonstrates how to list files in a directory on the FTP server using jsftp.
ftp.ls('remote/path/to/directory', (err, res) => {
if (err) return console.error(err);
res.forEach(file => {
console.log(file.name);
});
});
Deleting a file
This code demonstrates how to delete a file on the FTP server using jsftp.
ftp.raw('DELE', 'remote/path/to/file.txt', (err, res) => {
if (err) return console.error(err);
console.log('File deleted successfully');
});
The 'ftp' package is another FTP client for Node.js. It provides similar functionalities to jsftp, such as connecting to an FTP server, uploading, downloading, and deleting files. However, 'ftp' is known for its simplicity and ease of use, making it a good choice for basic FTP operations.
The 'promise-ftp' package is a promise-based FTP client for Node.js. It offers similar functionalities to jsftp but uses promises instead of callbacks, which can make the code cleaner and easier to manage, especially for complex workflows.
The 'ssh2-sftp-client' package is an SFTP client for Node.js. While it focuses on SFTP rather than FTP, it provides similar functionalities such as uploading, downloading, and managing files on a server. It is built on top of the 'ssh2' package and is known for its reliability and performance.
jsftp is a client FTP module for NodeJS that focuses on correctness, clarity and conciseness. It doesn't get in the middle of the user intentions.
jsftp gives the user access to all the raw commands of FTP in form of methods in the Ftp
object. It also provides several convenience methods for actions that require complex chains of commands (e.g. uploading and retrieving files). When commands succeed they always pass the response of the server to the callback, in the form of an object that contains two properties: code
, which is the response code of the FTP operation, and text
, which is the complete text of the response.
Raw (or native) commands are accessible in the form Ftp.raw["desired_command"](params, callback)
Thus, a command like QUIT
will be called like
Ftp.raw.quit(function(err, data) {
if (err)
throw err;
console.log("Bye!");
});
and a command like MKD
, which accepts parameters, will look like
Ftp.raw.mkd("/new_dir", function(err, data) {
if (err)
throw err;
console.log(data.text); // Presenting the FTP response text to the user
});
// Initialize some common variables
var user = "johndoe";
var pass = "12345"
var ftp = new Ftp({
host: "myhost.com",
port: 21, // The port defaults to 21, but let's include it anyway.
});
// First, we authenticate the user
ftp.auth(user, pass, function(err, res) {
if (err) throw err;
// Retrieve a file in the remote server. When the file has been retrieved,
// the callback will be called with `data` being the Buffer with the
// contents of the file.
// This is a convenience method that hides the actual complexity of setting
// up passive mode and retrieving files.
ftp.get("/folder/file.ext", function(err, data) {
if (err) throw err;
// Do something with the buffer
doSomething(data);
// We can use raw FTP commands directly as well. In this case we use FTP
// 'QUIT' method, which accepts no parameters and returns the farewell
// message from the server
ftp.raw.quit(function(err, res) {
if (err) throw err;
console.log("FTP session finalized! See you soon!");
});
});
});
// The following code assumes that you have authenticated the user, just like
// I did in the code above.
// Create a directory
ftp.raw.mkd("/example_dir", function(err, res) {
if (err)
throw err;
console.log(data.text);
});
// Delete a directory
ftp.raw.rmd("/example_dir", function(err, res) {
if (err)
throw err;
console.log(data.text);
});
You can find more usage examples in the unit tests for it. This documentation will grow as jsftp evolves, I promise!
Host name for the current FTP server.
Port number for the current FTP server (defaults to 21).
NodeJS socket for the current FTP server.
NodeJS socket for the current passive connection, if any.
features
is an array of feature names for the current FTP server. It is
generated when the user authenticates with the auth
method.
All the standard FTP commands are available under the raw
namespace. These
commands might accept parameters or not, but they always accept a callback
with the signature err, data
, in which err
is the error response coming
from the server (usually a 4xx or 5xx error code) and the data is an object
that contains two properties: code
and text
. code
is an integer indicating
the response code of the response and text
is the response stgring itself.
Authenticates the user with the given username and password. If null or empty
values are passed for those, auth
will use anonymous credentials. callback
will be called with the response text in case of successful login or with an
error as a first parameter, in normal Node fashion.
Lists filePath
contents using a passive connection.
Downloads filePath
from the server.
Uploads a file to filePath
. It accepts a buffer
parameter that will be
written in the remote file.
Renames a file in the server. from
and to
are both filepaths.
Lists information about files or directories and yields an array of file objects with parsed file properties to the callback. You should use this function instead of stat
or list
in case you need to do something with the individual files properties.
Refreshes the interval thats keep the server connection active. There is no need to call this method since it is taken care internally
With NPM:
npm install jsftp
From GitHub:
git clone https://github.com/sergi/jsftp.git
The test script fires up by default the FTP server that comes with OSX. You
will have to put your OSX user credentials in jsftp_test.js
if you want to
run it. If you are not on OSX, feel free to change the FTP host, port and
credentials to point to a remote server.
To run the tests in the command line:
node jsftp_test.js
If tests are failing it might be that your user doesn't have enough rights to
run the FTP service. In that case you should run the tests as sudo
:
sudo node jsftp_test.js
Please note that running scripts as sudo is dangerous and you will grant the script to do anything in your server. You should do it at your own risk.
See LICENSE.
FAQs
A sane FTP client implementation for NodeJS
The npm package jsftp receives a total of 230,077 weekly downloads. As such, jsftp popularity was classified as popular.
We found that jsftp demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.