What is jsii?
The jsii npm package is a tool that allows developers to write code in TypeScript and then use it in other programming languages such as Python, Java, and C#. It facilitates the creation of multi-language libraries by generating bindings for different languages from a single TypeScript codebase.
What are jsii's main functionalities?
TypeScript to Python
This feature allows you to write a class in TypeScript and then use it in Python by generating the necessary bindings.
const jsii = require('jsii');
// Define a TypeScript class
class HelloWorld {
sayHello() {
return 'Hello, world!';
}
}
// Export the class using jsii
module.exports = { HelloWorld };
// In Python, you can now use the generated bindings
# from your_module import HelloWorld
# hello = HelloWorld()
# print(hello.sayHello())
TypeScript to Java
This feature allows you to write a class in TypeScript and then use it in Java by generating the necessary bindings.
const jsii = require('jsii');
// Define a TypeScript class
class Calculator {
add(a, b) {
return a + b;
}
}
// Export the class using jsii
module.exports = { Calculator };
// In Java, you can now use the generated bindings
// Calculator calculator = new Calculator();
// int result = calculator.add(1, 2);
// System.out.println(result);
TypeScript to C#
This feature allows you to write a class in TypeScript and then use it in C# by generating the necessary bindings.
const jsii = require('jsii');
// Define a TypeScript class
class Greeter {
greet(name) {
return `Hello, ${name}!`;
}
}
// Export the class using jsii
module.exports = { Greeter };
// In C#, you can now use the generated bindings
// Greeter greeter = new Greeter();
// string greeting = greeter.greet("World");
// Console.WriteLine(greeting);
Other packages similar to jsii
swig
SWIG (Simplified Wrapper and Interface Generator) is a tool that connects programs written in C and C++ with various high-level programming languages. Unlike jsii, which focuses on TypeScript, SWIG is used for C/C++ and supports a wide range of languages including Python, Java, and C#.
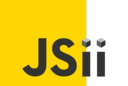

Overview
jsii
allows code in any language to naturally interact with JavaScript classes. It is the technology that enables the
AWS Cloud Development Kit to deliver polyglot libraries from a single codebase!
A class library written in TypeScript can be used in projects authored in TypeScript or Javascript (as
usual), but also in Python, Java, C# (and other languages from the .NET family), ...
:question: Documentation
Head over to our documentation website!
The jsii toolchain is spread out on multiple repositories:
- aws/jsii-compiler is where the
jsii
compiler is maintained (except releases
in the 1.x
line) - aws/jsii-rosetta is where the
jsii-rosetta
sample code transliteration tool
is maintained (except releases in the 1.x
line) - aws/jsii is where the rest of the toolchain is maintained, including:
@jsii/spec
, the package that defines the .jsii
assembly specificationjsii-config
, an interactive tool to help configure your jsii packagejsii-pacmak
, the bindings generator for jsii packagesjsii-reflect
, a higher-level way to process .jsii
assemblies- The jsii runtime libraries for the supported jsii target languages
1.x
release lines of jsii
and jsii-rosetta
:book: Blog Posts
Here's a collection of blog posts (in chronological order) related to jsii
:
:information_source: If you wrote blog posts about jsii
and would like to have them referenced here, do not hesitate
to file a pull request to add the links here!
:gear: Maintenance & Support
The applicable Maintenance & Support policy can be reviewed in SUPPORT.md.
The current status of jsii
compiler releases is:
Release | Status | EOS | Comment |
---|
5.5.x | Current | TBD |  |
5.4.x | Maintenance | 2024-02-28 |  |
5.3.x | Maintenance | 2024-10-07 |  |
1.x | Maintenance | 2024-10-31 | https://github.com/aws/jsii |
:balance_scale: License
jsii is distributed under the Apache License, Version 2.0.
See LICENSE and NOTICE for more information.
:gear: Contributing
See CONTRIBUTING.
Contributors ✨
Thanks goes to these wonderful people (emoji key):
This project follows the all-contributors specification.
Contributions of any kind welcome!