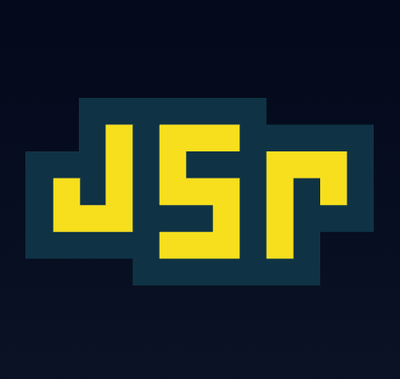
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
json-schema-faker
Advanced tools
The json-schema-faker npm package is a tool that generates fake data based on JSON Schema definitions. It is useful for testing, prototyping, and mocking APIs.
Basic JSON Schema Support
Generates fake data based on a simple JSON Schema. The schema defines an object with 'name' and 'age' properties, and json-schema-faker generates a corresponding fake object.
const jsf = require('json-schema-faker');
const schema = {
type: 'object',
properties: {
name: { type: 'string' },
age: { type: 'integer' }
},
required: ['name', 'age']
};
const fakeData = jsf.generate(schema);
console.log(fakeData);
Custom Formats
Allows the creation of custom formats for generating specific types of fake data. In this example, a custom format 'customFormat' is defined to always return 'customValue'.
const jsf = require('json-schema-faker');
jsf.format('customFormat', () => 'customValue');
const schema = {
type: 'object',
properties: {
customField: { type: 'string', format: 'customFormat' }
}
};
const fakeData = jsf.generate(schema);
console.log(fakeData);
Extending with Faker.js
Integrates with Faker.js to use its extensive library of fake data generators. In this example, the schema uses Faker.js to generate a fake email address.
const jsf = require('json-schema-faker');
const faker = require('faker');
jsf.extend('faker', () => faker);
const schema = {
type: 'object',
properties: {
email: { type: 'string', faker: 'internet.email' }
}
};
const fakeData = jsf.generate(schema);
console.log(fakeData);
Faker.js is a popular library for generating fake data. It provides a wide range of data types and is highly customizable. Unlike json-schema-faker, it does not use JSON Schema definitions but offers a rich API for generating fake data directly.
Chance.js is another library for generating random data. It is lightweight and provides a simple API for generating various types of random data. Like Faker.js, it does not use JSON Schema definitions but offers a straightforward way to generate random data.
Mockaroo is an online tool and API for generating fake data. It supports a wide range of data types and allows users to define schemas using a web interface. It is more user-friendly for non-developers compared to json-schema-faker.
json-schema-faker
supports (currently) the JSON-Schema specification draft-04 only (?)
var jsf = require('json-schema-faker');
var schema = {
type: 'object',
properties: {
user: {
type: 'object',
properties: {
id: {
$ref: '#/definitions/positiveInt'
},
name: {
type: 'string',
faker: 'name.findName'
},
email: {
type: 'string',
format: 'email',
faker: 'internet.email'
}
},
required: ['id', 'name', 'email']
}
},
required: ['user'],
definitions: {
positiveInt: {
type: 'integer',
minimum: 0,
minimumExclusive: true
}
}
};
var sample = jsf(schema);
console.log(sample.user.name);
// output: John Doe
Inline references are fully supported (json-pointers) but external can't be resolved by json-schema-faker
.
In order to achieve that you can use refaker and then use the resolved schemas:
var schema = {
type: 'object',
properties: {
someValue: {
$ref: 'otherSchema'
}
}
};
var refs = [
{
id: 'otherSchema',
type: 'string'
}
];
var sample = jsf(schema, refs);
console.log(sample.someValue);
// output: voluptatem
json-schema-faker
has built-in generators for core-formats, Faker.js and Chance.js are also supported.
You can use faker or chance properties but they are optional:
{
"type": "string",
"faker": "internet.email"
}
The above schema will invoke:
require('faker').internet.email();
Another example is passing arguments to the generator:
{
"type": "string",
"chance": {
"email": {
"domain": "fake.com"
}
}
}
And will invoke:
var Chance = require('chance'),
chance = new Chance();
chance.email({ "domain": "fake.com" });
If you pass an array, they will be used as raw arguments.
Note that both generators has higher precedence than format.
Additionally, you can add custom generators for those:
jsf.formats('semver', function(gen, schema) {
return gen.randexp('^\\d\\.\\d\\.\\d{1,2}$');
});
Now that format can be generated:
{
"type": "string",
"format": "semver"
}
Usage:
Callback:
Note that custom generators has lower precedence than core ones.
Actually, I've found some projects or services:
Many of they are incomplete (?), so I decided to code this library.
Any contribution is well received.
FAQs
JSON-Schema + fake data generators
The npm package json-schema-faker receives a total of 195,616 weekly downloads. As such, json-schema-faker popularity was classified as popular.
We found that json-schema-faker demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.