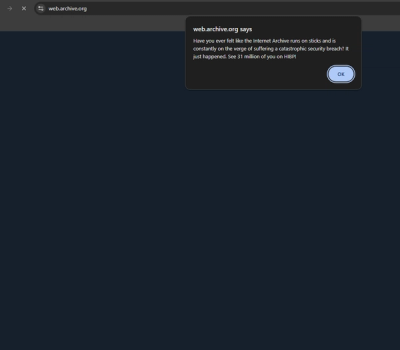
Security News
Internet Archive Hacked, 31 Million Record Compromised
The Internet Archive's "Wayback Machine" has been hacked and defaced, with 31 millions records compromised.
json-schema-to-typescript
Advanced tools
The json-schema-to-typescript npm package is a tool that converts JSON Schema definitions into TypeScript interfaces. This is particularly useful for ensuring type safety in TypeScript projects by generating types directly from JSON Schema, which can help in maintaining consistency between your data models and TypeScript types.
Convert JSON Schema to TypeScript Interface
This feature allows you to convert a JSON Schema into a TypeScript interface. The `compile` function takes a JSON Schema and a name for the resulting TypeScript interface, and returns a promise that resolves to the TypeScript code as a string.
const { compile } = require('json-schema-to-typescript');
const schema = {
title: 'Example Schema',
type: 'object',
properties: {
name: { type: 'string' },
age: { type: 'number' }
},
required: ['name', 'age']
};
compile(schema, 'ExampleSchema')
.then(ts => console.log(ts));
Generate TypeScript Definitions from Multiple Schemas
This feature allows you to generate TypeScript definitions from a JSON Schema file. The `compileFromFile` function reads a JSON Schema from a file and returns a promise that resolves to the TypeScript code as a string.
const { compileFromFile } = require('json-schema-to-typescript');
compileFromFile('path/to/schema.json')
.then(ts => console.log(ts));
Customizing Output
This feature allows you to customize the output of the TypeScript definitions. The `compile` function accepts an options object where you can specify various settings like adding a banner comment to the generated TypeScript code.
const { compile } = require('json-schema-to-typescript');
const schema = {
title: 'Example Schema',
type: 'object',
properties: {
name: { type: 'string' },
age: { type: 'number' }
},
required: ['name', 'age']
};
const options = {
bannerComment: '/* This is a generated file */'
};
compile(schema, 'ExampleSchema', options)
.then(ts => console.log(ts));
The typescript-json-schema package generates JSON Schema from your TypeScript types. It works in the opposite direction compared to json-schema-to-typescript, making it useful for projects where you start with TypeScript types and need to generate JSON Schema for validation or documentation purposes.
The quicktype package can generate TypeScript types from JSON Schema, JSON data, and other sources. It supports multiple languages and is highly configurable, making it a versatile tool for generating types from various data formats.
The json-schema-to-flow-type package converts JSON Schema to Flow types. While it serves a similar purpose to json-schema-to-typescript, it is specifically designed for projects using Flow instead of TypeScript.
Compile json schema to typescript typings
Input:
{
"title": "Example Schema",
"type": "object",
"properties": {
"firstName": {
"type": "string"
},
"lastName": {
"type": "string"
},
"age": {
"description": "Age in years",
"type": "integer",
"minimum": 0
},
"hairColor": {
"enum": ["black", "brown", "blue"],
"type": "string"
}
},
"additionalProperties": false,
"required": ["firstName", "lastName"]
}
Output:
export interface ExampleSchema {
firstName: string;
lastName: string;
/**
* Age in years
*/
age?: number;
hairColor?: "black" | "brown" | "blue";
}
# Using Yarn:
yarn add json-schema-to-typescript
# Or, using NPM:
npm install json-schema-to-typescript --save
import { compile, compileFromFile } from 'json-schema-to-typescript'
// compile from file
compileFromFile('foo.json')
.then(ts => fs.writeFileSync('foo.d.ts', ts))
// or, compile a JS object
let mySchema = {
properties: [...]
}
compile(mySchema, 'MySchema')
.then(ts => ...)
See server demo and browser demo for full examples.
compileFromFile
and compile
accept options as their last argument (all keys are optional):
key | type | default | description |
---|---|---|---|
additionalProperties | boolean | true | Default value for additionalProperties , when it is not explicitly set |
bannerComment | string | "/* eslint-disable */\n/**\n* This file was automatically generated by json-schema-to-typescript.\n* DO NOT MODIFY IT BY HAND. Instead, modify the source JSONSchema file,\n* and run json-schema-to-typescript to regenerate this file.\n*/" | Disclaimer comment prepended to the top of each generated file |
cwd | string | process.cwd() | Root directory for resolving $ref s |
declareExternallyReferenced | boolean | true | Declare external schemas referenced via $ref ? |
enableConstEnums | boolean | true | Prepend enums with const ? |
format | boolean | true | Format code? Set this to false to improve performance. |
ignoreMinAndMaxItems | boolean | false | Ignore maxItems and minItems for array types, preventing tuples being generated. |
maxItems | number | 20 | Maximum number of unioned tuples to emit when representing bounded-size array types, before falling back to emitting unbounded arrays. Increase this to improve precision of emitted types, decrease it to improve performance, or set it to -1 to ignore maxItems . |
strictIndexSignatures | boolean | false | Append all index signatures with | undefined so that they are strictly typed. |
style | object | { bracketSpacing: false, printWidth: 120, semi: true, singleQuote: false, tabWidth: 2, trailingComma: 'none', useTabs: false } | A Prettier configuration |
unknownAny | boolean | true | Use unknown instead of any where possible |
unreachableDefinitions | boolean | false | Generates code for $defs that aren't referenced by the schema. |
$refOptions | object | {} | $RefParser Options, used when resolving $ref s |
A CLI utility is provided with this package.
cat foo.json | json2ts > foo.d.ts
# or
json2ts foo.json > foo.d.ts
# or
json2ts foo.json foo.d.ts
# or
json2ts --input foo.json --output foo.d.ts
# or
json2ts -i foo.json -o foo.d.ts
# or (quote globs so that your shell doesn't expand them)
json2ts -i 'schemas/**/*.json'
# or
json2ts -i schemas/ -o types/
You can pass any of the options described above (including style options) as CLI flags. Boolean values can be set to false using the no-
prefix.
# generate code for definitions that aren't referenced
json2ts -i foo.json -o foo.d.ts --unreachableDefinitions
# use single quotes and disable trailing semicolons
json2ts -i foo.json -o foo.d.ts --style.singleQuote --no-style.semi
npm test
title
=> interface
deprecated
allOf
("intersection")anyOf
("union")oneOf
(treated like anyOf
)maxItems
(eg)minItems
(eg)additionalProperties
of typepatternProperties
(partial support)extends
required
properties on objects (eg)validateRequired
(eg)tsType
tsType
: Overrides the type that's generated from the schema. Useful for forcing a type to any
or when using non-standard JSON schema extensions (eg).tsEnumNames
: Overrides the names used for the elements in an enum. Can also be used to create string enums (eg).dependencies
(single, multiple)divisibleBy
(eg)format
(eg)multipleOf
(eg)maximum
(eg)minimum
(eg)maxProperties
(eg)minProperties
(eg)not
/disallow
oneOf
("xor", use anyOf
instead)pattern
(string, regex)uniqueItems
(eg)Prettier is known to run slowly on really big files. To skip formatting and improve performance, set the format
option to false
.
FAQs
compile json schema to typescript typings
The npm package json-schema-to-typescript receives a total of 492,599 weekly downloads. As such, json-schema-to-typescript popularity was classified as popular.
We found that json-schema-to-typescript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The Internet Archive's "Wayback Machine" has been hacked and defaced, with 31 millions records compromised.
Security News
TC39 is meeting in Tokyo this week and they have approved nearly a dozen proposals to advance to the next stages.
Security News
Our threat research team breaks down two malicious npm packages designed to exploit developer trust, steal your data, and destroy data on your machine.