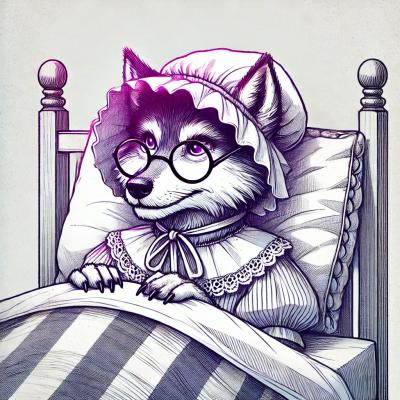
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
json-to-fs-structure
Advanced tools
A simple module that takes a simple JSON file and produces the properties as a file structure in the same directory or given directory.
Clean and simple JavaScript project for turning JSON objects into directory structures.
For development, clone into this repository and to install run:
yarn install
To test run:
yarn test
To use this node module, install it like so:
yarn add json-to-fs-structure
And (as it's currently intended to be used on the server) usage looks like this on a simple node server:
var express = require('express');
var { jsonToFsStructure } = require('json-to-fs-structure');
var app = express();
app.get('/', function(req, res){
const options = {
jsonObject: {
testArrayField5: [
{ somedir: {} },
{ anotherdir: {} },
{
andanotherdir: {
interiorone: {
interiortwo: {
interiorthree: [{ interiorfour: {} }, { interiorfive: {} }]
}
}
}
}
]
}
};
jsonToFsStructure(options);
res.send("Hello world!");
});
app.listen(3000);
Or if you want to see how it works synchronously (server only returns after it is written to the root directory), your code would look more like this:
var express = require('express');
var { jsonToFsStructure } = require('json-to-fs-structure');
var app = express();
app.get('/', function(req, res){
const options = {
jsonObject: {
testArrayField5: [
{ somedir: {} },
{ anotherdir: {} },
{
andanotherdir: {
interiorone: {
interiortwo: {
interiorthree: [{ interiorfour: {} }, { interiorfive: {} }]
}
}
}
}
]
},
filePath: ".",
callback: () => res.send("Hello world!"))
};
jsonToFsStructure(options);
});
app.listen(3000);
Both of those examples would leave you with directory trees that look like this:
Suppose you want to execute a function for each directory whether it be a terminating (leaf) directory or another directory. The function format is as follows:
const procedure = (newPath, accumulator, obj) => {
// something that takes the just created directory (relative newPath)
// and the accumulator (a structure you can provide that continues through each call)
// and the obj, the value of the nested structure if this is a leaf node it's {}
// lastly we return the accumulator to persist it
return accumulator;
}
The corresponding functions for the above executing procedures are: jsonToFsWithLeafFunction
, jsonToFsWithNonLeafFunction
and jsonToFsWithFunction
. So an example usage in a simple express server would look like this:
var express = require('express');
var { jsonToFsWithLeafFunction } = require('json-to-fs-structure');
var app = express();
const procedure = (newPath, accumulator, obj) => {
accumulator.contextvalue += 2;
accumulator.paths.push(newPath);
return accumulator;
};
app.get('/', function(req, res){
let passByValueContext = {"contextvalue": 1, paths: []};
const options = {
jsonObject: {
testArrayField5: [
{ somedir: {} },
{ anotherdir: {} },
{
andanotherdir: {
interiorone: {
interiortwo: {
interiorthree: [{ interiorfour: {} }, { interiorfive: {} }]
}
}
}
}
]
},
procedure,
context: passByValueContext
};
jsonToFsWithLeafFunction(options);
console.log(passByValueContext);
res.send("Hello world!");
});
app.listen(3000);
What we expect to see in the console when this runs is:
{ contextvalue: 9,
paths:
[ './testArrayField5/somedir',
'./testArrayField5/anotherdir',
'./testArrayField5/andanotherdir/interiorone/interiortwo/interiorthree/interiorfour',
'./testArrayField5/andanotherdir/interiorone/interiortwo/interiorthree/interiorfive' ] }
And since our context value is scoped to each request, you will see that for every GET method.
FAQs
A simple module that takes a simple JSON file and produces the properties as a file structure in the same directory or given directory.
We found that json-to-fs-structure demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.