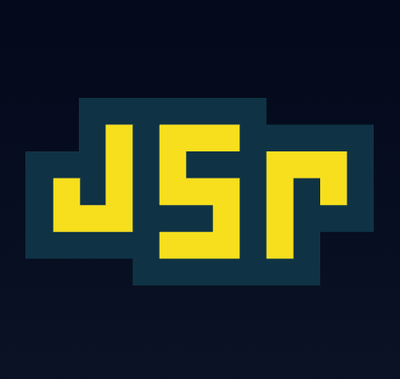
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
JSS (JavaScript Style Sheets) is a library for generating CSS styles with JavaScript. It allows you to define styles in a JavaScript object and apply them to your components, making it easier to manage and maintain styles in a JavaScript-centric development environment.
Creating Styles
This feature allows you to create styles using JavaScript objects. The `createStyleSheet` method generates a stylesheet from the provided styles and attaches it to the document.
const styles = {
button: {
color: 'blue',
background: 'white',
border: '1px solid blue'
}
};
const { classes } = jss.createStyleSheet(styles).attach();
// Usage in a component
const button = document.createElement('button');
button.className = classes.button;
button.textContent = 'Click me';
document.body.appendChild(button);
Dynamic Styles
This feature allows you to create dynamic styles that can change based on props or state. The `update` method is used to update the styles with new values.
const styles = {
button: {
color: props => props.color,
background: 'white',
border: '1px solid blue'
}
};
const sheet = jss.createStyleSheet(styles);
const { classes } = sheet.update({ color: 'red' }).attach();
// Usage in a component
const button = document.createElement('button');
button.className = classes.button;
button.textContent = 'Click me';
document.body.appendChild(button);
Theming
This feature allows you to create themes that can be applied to your styles. The styles can reference theme variables, making it easy to switch themes or update theme values.
const theme = {
primaryColor: 'blue',
secondaryColor: 'green'
};
const styles = theme => ({
button: {
color: theme.primaryColor,
background: 'white',
border: `1px solid ${theme.primaryColor}`
}
});
const sheet = jss.createStyleSheet(styles(theme)).attach();
const { classes } = sheet;
// Usage in a component
const button = document.createElement('button');
button.className = classes.button;
button.textContent = 'Click me';
document.body.appendChild(button);
Styled-components is a library for styling React components using tagged template literals. It allows you to write actual CSS code to style your components and supports theming and dynamic styling. Compared to JSS, styled-components is more tightly integrated with React and uses a different syntax for defining styles.
Emotion is a library designed for writing CSS styles with JavaScript. It provides both a styled API similar to styled-components and a css API for defining styles as objects. Emotion is known for its performance and flexibility, offering a similar feature set to JSS but with a different API and additional performance optimizations.
Aphrodite is a library for styling React components with JavaScript. It allows you to define styles as JavaScript objects and provides support for media queries and pseudo-selectors. Aphrodite is simpler and more lightweight compared to JSS, but it may lack some of the advanced features and flexibility that JSS offers.

JSS is a very thin layer which compiles JSON structures to CSS.
Why do we need transpilers like sass or stylus when we can use javascript to do the same and much more?
By leveraging namespaces we can solve the cascading problem better than bem and make our components truly reusable and composable.
Access css declarations and values from js without DOM round trip.
Smaller footprint because of code reuse and no vendor specific declarations
Take a look at examples directory.
Jss styles are just plain javascript objects. They map 1:1 to css rules, except of those modified by plugins.
// Some random jss code example
{
'.carousel-caption': {
'position': 'absolute',
'z-index': '10',
},
'hr': {
'border': '0',
'border-top': '1px solid #eee'
},
'@media (min-width: 768px)': {
'.modal-dialog': {
'width': '600px',
'margin': '30px auto'
},
'.modal-content': {
'box-shadow': '0 5px 15px rgba(0, 0, 0, .5)'
},
'.modal-sm': {
'width': '300px'
}
}
}
I recommend to not to use this if you use jss on the client. Instead you should write a function, which makes a test for this feature support and generates just one final declaration.
In case you are using jss as a server side precompiler, you might want to have more than one property with identical name. This is not possible in js, but you can use an array.
{
'.container': {
background: [
'red',
'-moz-linear-gradient(left, red 0%, green 100%)',
'-webkit-linear-gradient(left, red 0%, green 100%)',
'-o-linear-gradient(left, red 0%, green 100%)',
'-ms-linear-gradient(left, red 0%, green 100%)',
'linear-gradient(to right, red 0%, green 100%)'
]
}
}
.container {
background: red;
background: -moz-linear-gradient(left, red 0%, green 100%);
background: -webkit-linear-gradient(left, red 0%, green 100%);
background: -o-linear-gradient(left, red 0%, green 100%);
background: -ms-linear-gradient(left, red 0%, green 100%);
background: linear-gradient(to right, red 0%, green 100%);
}
// Pure js
var jss = window.jss
// Commonjs
var jss = require('jss')
jss.createStyleSheet([rules], [named], [attributes])
rules
is an object, where keys are selectors if named
is not truenamed
rules keys are not used as selectors, but as names, will cause auto generated class names and selectors. It will also make class names accessible via styleSheet.classes
.attributes
allows to set any attributes on style element.var styleSheet = jss.createStyleSheet({
'.selector': {
width: '100px'
}
}, {media: 'print'}).attach()
<style media="print">
.selector {
width: 100px;
}
</style>
Create a style sheet with namespaced rules. For this set second parameter to true
.
var styleSheet = jss.createStyleSheet({
myButton: {
width: '100px',
height: '100px'
}
}, true).attach()
console.log(styleSheet.classes.myButton) // .jss-0
<style>
.jss-0 {
width: 100px;
height: 100px;
}
</style>
styleSheet.attach()
Insert style sheet into render tree.
styleSheet.attach()
styleSheet.detach()
Remove style sheet from render tree to increase runtime performance.
styleSheet.detach()
styleSheet.addRule([selector], rule)
Returns an array of rules, because you might have a nested rule in your style.
var rules = styleSheet.addRule('.my-button', {
padding: '20px',
background: 'blue'
})
var rules = styleSheet.addRule({
padding: '20px',
background: 'blue'
})
document.body.innerHTML = '<button class="' + rules[0].className + '">Button</button>'
styleSheet.getRule(selector)
// Using selector
var rule = styleSheet.getRule('.my-button')
// Using name, if named rule was added.
var rule = styleSheet.getRule('myButton')
styleSheet.addRules(rules)
Add a list of rules.
styleSheet.addRules({
'.my-button': {
float: 'left',
},
'.something': {
display: 'none'
}
})
jss.createRule([selector], rule)
var rule = jss.createRule({
padding: '20px',
background: 'blue'
})
// Apply styles directly using jquery.
$('.container').css(rule.style)
jss.use(fn)
Passed function will be invoked with Rule instance. Take a look at plugins like extend
, nested
or vendorPrefixer
.
jss.use(function(rule) {
// Your modifier.
})
styleSheet.toString()
If you want to get a pure CSS string from jss e.g. for preprocessing jss on the server.
var jss = require('jss')
var styleSheet = jss.createStyleSheet({
'.my-button': {
float: 'left',
}
})
console.log(styleSheet.toString())
.my-button {
float: left;
}
Things you know from stylus like @extend, nested selectors, vendor prefixer are separate plugins.
Full list of available plugins
npm install jss
#or
bower install jsstyles
# print help
jss
# convert css
jss source.css -p > source.jss
How fast would bootstrap css lib render?
I have converted bootstraps css to jss. In bench/bootstrap
folder you will find jss and css files. You need to try more than once to have some average value.
On my machine overhead is about 10-15ms.
Rendering jss vs. css (same styles) jsperf bench.
npm i
open test/local.html
MIT
FAQs
A lib for generating Style Sheets with JavaScript.
The npm package jss receives a total of 1,686,577 weekly downloads. As such, jss popularity was classified as popular.
We found that jss demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.