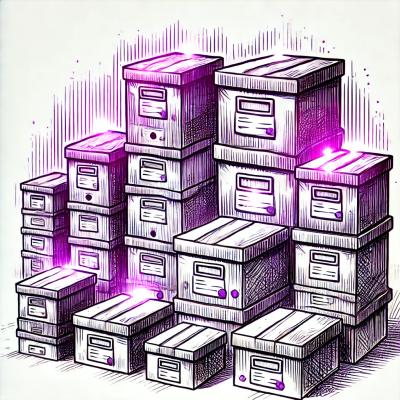
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
For inheritance in javascript.
$ npm install jujsoop
var juoop = require('jujsoop');
function Person(name) {
this.name = name;
}
function Male (name) {
this.gender = "M";
}
// Change the person class to person abtraction.
Person = juoop.abstract(Person);
// The male class inherits from the person class.
Male = juoop.inherit(Male, Person);
// Add prototype to the person class.
Person.prototype.cv = function () {
console.log("Person.prototype.cv");
console.log("Name: " + this.name);
}
// The male class shows the cv prototype of the person class.
new Male("JosephUz").cv();
// Override the cv prototype of the male class.
Male.prototype.cv = function () {
console.log("Male.prototype.cv");
console.log("Name: " + this.name + '\nGender: ' + this.gender);
}
// The male class shows own cv prototype.
new Male("JosephUz").cv();
juoop.abstract(type)
Parameters:
type
: Type to be used.juoop.inherit(type, base)
Anymore, when creating a new instance of type, the last parameter of constructor will be base and in the same way the last parameter of base constructor will be type. Also, type constructor can only be called with "new" keyword or when base constructor has no returns. However, base constructor is always called.
Parameters:
type
: Type to be used.
base
: Inherited type.
juoop.type
The object from which the related methods are handled.
juoop.type.derived(type, base)
Checking that the type is derived from the base.
Parameters:
type
: Type to be used.
base
: Inherited type.
juoop.type.clone(type)
Cloning with all the features of a type.
Parameters:
type
: Type to be used.juoop.attribute
Used to manage operations before or after functions.
juoop.attribute.inherit(attr)
Used to create a new attribute.
Parameters:
attr
: Constructor function of the attribute to be derived.juoop.attribute.bind(func, attr, ...attr)
Used to bind the attribute with the function.
Parameters:
func
: Function to which the attribute will be bound.
attr
: Constructor function of the derived attribute. Multiple attributes can be written as parameters at the same bind process.
juoop.attribute.getAppliedAttributes(scope, attr)
Used to get the applied attributes of a variable.
Parameters:
scope
: The variable that attributes are applied to.
attr
: Optional attribute parameter that is used to get the value of the attribute.
juoop.attribute.getBoundAttributes(func, attr)
Used to get the applied attributes of a function.
Parameters:
func
: The function that attributes are bound to.
attr
: Optional attribute parameter that is used to get the value of the attribute.
juoop.attribute.getAttributeResult(scope)
Used to get the result of the applied attributes to variable.
Parameters:
scope
: The variable that attributes are applied to.This example shows the most basic way of usage.
This example shows a way of the attribute usage.
This software is free to use under the JosephUz. See the LICENSE file for license text and copyright information.
FAQs
For inheritance in javascript.
We found that jujsoop demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.