Comparing version 1.0.9 to 1.1.1
@@ -0,16 +1,30 @@ | ||
var __assign = (this && this.__assign) || function () { | ||
__assign = Object.assign || function(t) { | ||
for (var s, i = 1, n = arguments.length; i < n; i++) { | ||
s = arguments[i]; | ||
for (var p in s) if (Object.prototype.hasOwnProperty.call(s, p)) | ||
t[p] = s[p]; | ||
} | ||
return t; | ||
}; | ||
return __assign.apply(this, arguments); | ||
}; | ||
import React from 'react'; | ||
import defaultOptions from '../../defaultOptions'; | ||
import { isEditableCell } from '../../Utils/CellUtils'; | ||
import { getRowEditableCells } from '../../Utils/FilterUtils'; | ||
import CellComponent from '../CellComponent/CellComponent'; | ||
import DataRowContent from '../DataRowContent/DataRowContent'; | ||
import EmptyCells from '../EmptyCells/EmptyCells'; | ||
var DataRow = function (_a) { | ||
var columns = _a.columns, editableCells = _a.editableCells, editingMode = _a.editingMode, groupColumnsCount = _a.groupColumnsCount, dispatch = _a.dispatch, onRowDataChanged = _a.onRowDataChanged, rowData = _a.rowData, rowKeyField = _a.rowKeyField, _b = _a.selectedRows, selectedRows = _b === void 0 ? [] : _b, height = _a.height, trRef = _a.trRef; | ||
var DataRow = function (props) { | ||
var editableCells = props.editableCells, groupColumnsCount = props.groupColumnsCount, height = props.height, rowData = props.rowData, rowKeyField = props.rowKeyField, dataRow = props.dataRow, _a = props.selectedRows, selectedRows = _a === void 0 ? [] : _a, trRef = props.trRef; | ||
var rowKeyValue = rowData[rowKeyField]; | ||
var rowEditableCells = getRowEditableCells(rowKeyValue, editableCells); | ||
var isSelectedRow = selectedRows.some(function (s) { return s === rowKeyValue; }); | ||
var dataRowProps = __assign(__assign({}, props), { isSelectedRow: isSelectedRow, rowEditableCells: rowEditableCells, rowKeyValue: rowKeyValue }); | ||
var dataRowContent = dataRow && dataRow(dataRowProps); | ||
return (React.createElement("tr", { ref: trRef, style: { height: height }, className: defaultOptions.css.row + " " + (isSelectedRow ? defaultOptions.css.rowSelected : '') }, | ||
React.createElement(EmptyCells, { count: groupColumnsCount }), | ||
columns.map(function (column) { return (React.createElement(CellComponent, { column: column, editingMode: editingMode, isEditableCell: isEditableCell(editingMode, column, rowEditableCells), isSelectedRow: isSelectedRow, key: column.key, dispatch: dispatch, onRowDataChanged: onRowDataChanged, rowData: rowData, rowKeyField: rowKeyField })); }))); | ||
dataRowContent | ||
? React.createElement("td", { className: defaultOptions.css.cell }, dataRowContent) | ||
: React.createElement(DataRowContent, __assign({}, dataRowProps)))); | ||
}; | ||
export default DataRow; |
@@ -29,3 +29,4 @@ var __assign = (this && this.__assign) || function () { | ||
var itemHeight = firstRowRef.current.offsetHeight || 40; | ||
var tbodyHeight = (firstRowRef.current.parentElement && firstRowRef.current.parentElement.offsetHeight) || 600; | ||
var tbodyHeight = (firstRowRef.current.parentElement && firstRowRef.current.parentElement.offsetHeight) | ||
|| 600; | ||
var newVirtualScrolling = __assign({ itemHeight: itemHeight, | ||
@@ -32,0 +33,0 @@ tbodyHeight: tbodyHeight }, virtualScrolling); |
{ | ||
"name": "ka-table", | ||
"version": "1.0.9", | ||
"version": "1.1.1", | ||
"license": "MIT", | ||
@@ -16,2 +16,3 @@ "repository": "github:komarovalexander/ka-table", | ||
"lint:fix": "tslint --fix -c tslint.json 'src/**/*.{ts,tsx}'", | ||
"coveralls": "cat ./coverage/lcov.info | node node_modules/.bin/coveralls", | ||
"pack": "gulp build && cd dist && npm pack && cd .." | ||
@@ -18,0 +19,0 @@ }, |
@@ -8,43 +8,5 @@ [](https://github.com/komarovalexander/ka-table/blob/master/LICENSE) | ||
Can easily be included in react projects, never mind it is ts or js | ||
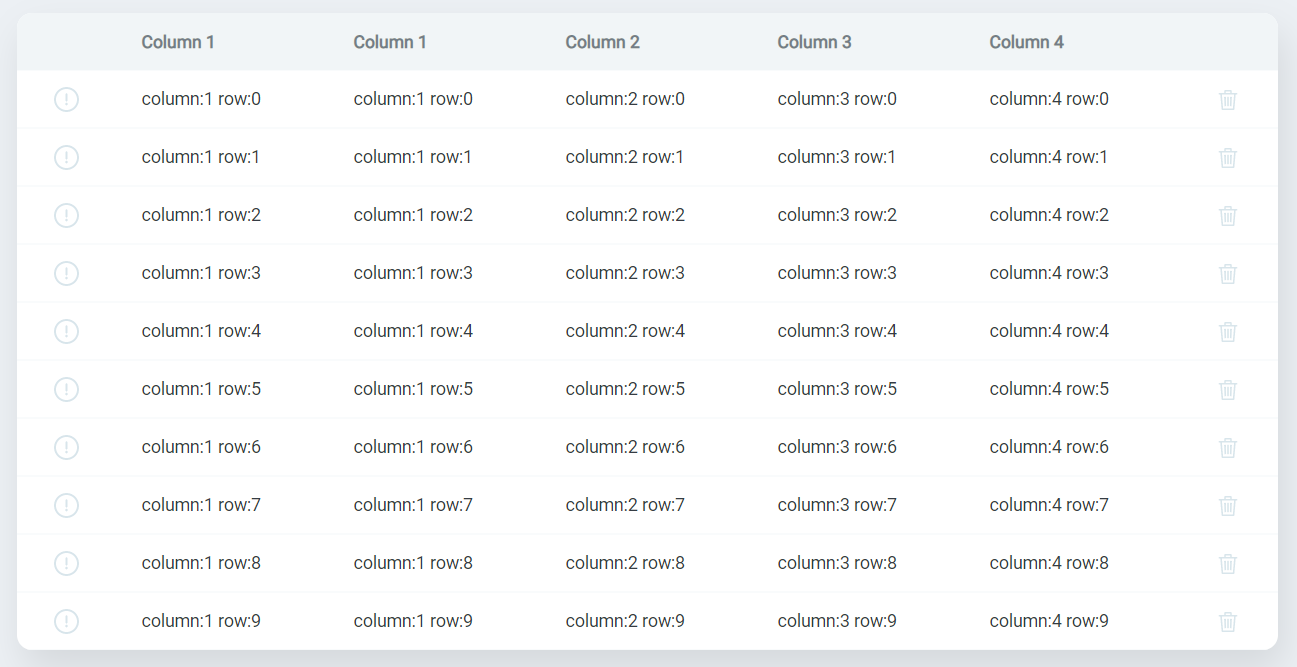 | ||
[Demo link](https://komarovalexander.github.io/ka-table/#/command-column) | ||
## Examples | ||
[Command Column](https://komarovalexander.github.io/ka-table/#/command-column) - Functional columns which are not bound to data and used to add custom command to table | ||
[Custom Cell](https://komarovalexander.github.io/ka-table/#/custom-cell) - Best way to customise look of every column in table | ||
[Custom Editor](https://komarovalexander.github.io/ka-table/#/custom-editor) - Table supports user created editors | ||
[Custom Header Cell](https://komarovalexander.github.io/ka-table/#/custom-header-cell) - Customisation of header cell | ||
[Editing](https://komarovalexander.github.io/ka-table/#/editing) - Editing out of the box | ||
[Events](https://komarovalexander.github.io/ka-table/#/events) - Most events are trackable | ||
[Filter Extended](https://komarovalexander.github.io/ka-table/#/filter-extended) - Easy filtered by extended filters | ||
[Filter Row](https://komarovalexander.github.io/ka-table/#/filter-row) - Built-in filter row | ||
[Filter Row - Custom Editor](https://komarovalexander.github.io/ka-table/#/filter-row-custom-editor) - Customise filter cell every way you want | ||
[Grouping](https://komarovalexander.github.io/ka-table/#/grouping) - Group data for most convenient work with it | ||
[25000 Rows](https://komarovalexander.github.io/ka-table/#/many-rows) - Virtualisation are supported | ||
[10000 Grouped Rows](https://komarovalexander.github.io/ka-table/#/many-rows-grouping) - Virtualisation work well with grouping | ||
[Search](https://komarovalexander.github.io/ka-table/#/search) - Search by the whole Table is easy | ||
[Selection](https://komarovalexander.github.io/ka-table/#/selection) - Select and process specific rows | ||
[Sorting](https://komarovalexander.github.io/ka-table/#/sorting) | ||
[State Storing](https://komarovalexander.github.io/ka-table/#/state-storing) - Save Table's state and restore it after page reload | ||
[Validation](https://komarovalexander.github.io/ka-table/#/validation) | ||
## Installation | ||
@@ -307,2 +269,2 @@ npm | ||
| openEditor | () => void | call this method to open editor of the cell | | ||
| rowData | any | data of the row in which editor is shown | | ||
| rowData | any | data of the row in which editor is shown | |
@@ -5,2 +5,3 @@ import { PropsWithChildren } from 'react'; | ||
import { ICellEditorProps } from './Components/CellEditor/CellEditor'; | ||
import { IDataRowProps } from './Components/DataRowContent/DataRowContent'; | ||
import { IHeadCellProps } from './Components/HeadCell/HeadCell'; | ||
@@ -12,2 +13,4 @@ import { Column } from './models'; | ||
export type DataChangeFunc = (data: any[]) => void; | ||
export type DataRowFunc = (props: DataRowFuncPropsWithChildren) => any; | ||
export type DataRowFuncPropsWithChildren = PropsWithChildren<IDataRowProps>; | ||
export type EditorFunc = (props: EditorFuncPropsWithChildren) => any; | ||
@@ -14,0 +17,0 @@ export type EditorFuncPropsWithChildren = PropsWithChildren<ICellEditorProps>; |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
162749
118
2695
269