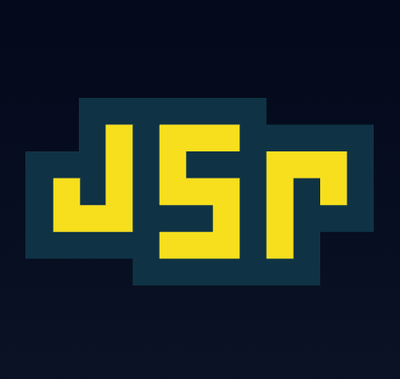
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
karma-webpack
Advanced tools
karma-webpack is a plugin that allows you to use Webpack to preprocess files in Karma. It enables you to bundle your test files using Webpack, which is particularly useful for projects that already use Webpack for their build process. This integration helps in leveraging Webpack's features like module bundling, code splitting, and asset management in your test environment.
Preprocessing Files
This feature allows you to preprocess your test files using Webpack. By specifying the files and preprocessors in the Karma configuration, you can bundle your test files with Webpack before running them in the browser.
module.exports = function(config) {
config.set({
frameworks: ['mocha'],
files: [
'test/**/*.spec.js'
],
preprocessors: {
'test/**/*.spec.js': ['webpack']
},
webpack: {
// Webpack configuration
},
browsers: ['Chrome'],
singleRun: true
});
};
Using Webpack Loaders
This feature allows you to use Webpack loaders in your test environment. For example, you can use Babel to transpile your ES6 code to ES5 before running your tests.
module.exports = function(config) {
config.set({
frameworks: ['jasmine'],
files: [
'test/**/*.spec.js'
],
preprocessors: {
'test/**/*.spec.js': ['webpack']
},
webpack: {
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: 'babel-loader'
}
]
}
},
browsers: ['Firefox'],
singleRun: true
});
};
Source Maps
This feature allows you to generate source maps for your test files. Source maps help in debugging by mapping the transpiled code back to the original source code.
module.exports = function(config) {
config.set({
frameworks: ['mocha'],
files: [
'test/**/*.spec.js'
],
preprocessors: {
'test/**/*.spec.js': ['webpack']
},
webpack: {
devtool: 'inline-source-map'
},
browsers: ['Chrome'],
singleRun: true
});
};
karma-browserify is a plugin that allows you to use Browserify to bundle your test files in Karma. Like karma-webpack, it preprocesses your test files, but it uses Browserify instead of Webpack. Browserify is another popular module bundler that transforms and bundles JavaScript files.
karma-rollup-preprocessor is a plugin that integrates Rollup with Karma. Rollup is a module bundler that compiles small pieces of code into something larger and more complex, such as a library or application. This plugin allows you to preprocess your test files using Rollup, similar to how karma-webpack uses Webpack.
karma-esbuild is a plugin that uses esbuild to bundle and transpile your test files in Karma. esbuild is an extremely fast JavaScript bundler and minifier. This plugin provides similar functionality to karma-webpack but leverages the speed and performance of esbuild.
npm npm i -D karma-webpack
yarn yarn add -D karma-webpack
karma.conf.js
module.exports = (config) => {
config.set({
// ... normal karma configuration
// make sure to include webpack as a framework
frameworks: ['mocha', 'webpack'],
plugins: [
'karma-webpack',
'karma-mocha',
],
files: [
// all files ending in ".test.js"
// !!! use watched: false as we use webpacks watch
{ pattern: 'test/**/*.test.js', watched: false }
],
preprocessors: {
// add webpack as preprocessor
'test/**/*.test.js': [ 'webpack' ]
},
webpack: {
// karma watches the test entry points
// Do NOT specify the entry option
// webpack watches dependencies
// webpack configuration
},
});
}
This configuration will be merged with what gets provided via karma's config.webpack.
const defaultWebpackOptions = {
mode: 'development',
output: {
filename: '[name].js',
path: path.join(os.tmpdir(), '_karma_webpack_') + Math.floor(Math.random() * 1000000),
},
stats: {
modules: false,
colors: true,
},
watch: false,
optimization: {
runtimeChunk: 'single',
splitChunks: {
chunks: 'all',
minSize: 0,
cacheGroups: {
commons: {
name: 'commons',
chunks: 'initial',
minChunks: 1,
},
},
},
},
plugins: [],
};
This project is a framework and preprocessor for Karma that combines test files and dependencies into 2 shared bundles and 1 chunk per test file. It relies on webpack to generate the bundles/chunks and to keep it updated during autoWatch=true
.
The first preproccessor triggers the build of all the bundles/chunks and all following files just return the output of this one build process.
By default karma-webpack forces *.js files so if you test *.ts files and use webpack to build typescript to javascript it works out of the box.
If you have a different need you can override by settig webpack.transformPath
// this is the by default applied transformPath
webpack: {
transformPath: (filepath) => {
// force *.js files by default
const info = path.parse(filepath);
return `${path.join(info.dir, info.name)}.js`;
},
},
Source Maps
You can use the karma-sourcemap-loader
to get the source maps generated for your test bundle.
npm i -D karma-sourcemap-loader
And then add it to your preprocessors.
karma.conf.js
preprocessors: {
'test/test_index.js': [ 'webpack', 'sourcemap' ]
}
And tell webpack
to generate sourcemaps.
webpack.config.js
webpack: {
// ...
devtool: 'inline-source-map'
}
Cody Mikol |
Previous maintainers of the karma-webpack
plugin that have done such amazing work to get it to where it is today.
Ryan Clark |
April Arcus |
Mika Kalathil |
|
|
|
|
|
FAQs
Use webpack with karma
We found that karma-webpack demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.