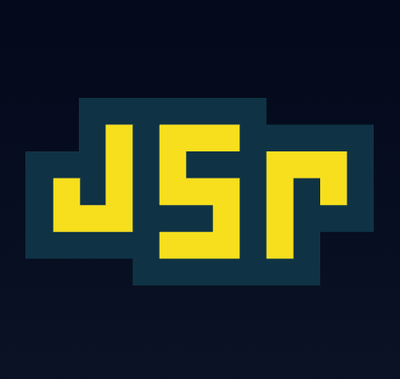
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
The keccak npm package provides an implementation of the Keccak cryptographic hash function, which is the basis for the SHA-3 (Secure Hash Algorithm 3) standard. It allows developers to generate hash digests of data, which can be used for data integrity verification, digital signatures, and other cryptographic applications.
Hashing a string
This feature allows you to hash a string using the Keccak-256 algorithm. The code sample demonstrates how to hash the string 'hello world' and output the resulting hash in hexadecimal format.
const keccak = require('keccak');
const hash = keccak('keccak256').update('hello world').digest('hex');
console.log(hash);
Hashing a buffer
This feature allows you to hash a buffer using the Keccak-256 algorithm. The code sample demonstrates how to hash a buffer containing the string 'hello world' and output the resulting hash in hexadecimal format.
const keccak = require('keccak');
const buffer = Buffer.from('hello world');
const hash = keccak('keccak256').update(buffer).digest('hex');
console.log(hash);
Hashing with different Keccak variants
This feature allows you to use different variants of the Keccak algorithm, such as Keccak-256 and Keccak-512. The code sample demonstrates how to hash the string 'hello world' using both Keccak-256 and Keccak-512, and output the resulting hashes in hexadecimal format.
const keccak = require('keccak');
const hash256 = keccak('keccak256').update('hello world').digest('hex');
const hash512 = keccak('keccak512').update('hello world').digest('hex');
console.log('Keccak-256:', hash256);
console.log('Keccak-512:', hash512);
The sha3 package provides an implementation of the SHA-3 (Secure Hash Algorithm 3) standard, which is based on the Keccak algorithm. It offers similar functionality to the keccak package, allowing you to generate SHA-3 hash digests of data. The main difference is that sha3 focuses on the standardized SHA-3 variants, while keccak provides more direct access to the Keccak algorithm itself.
The crypto-js package is a widely-used library that provides a variety of cryptographic algorithms, including SHA-3. It offers a broader range of cryptographic functions compared to keccak, such as AES encryption, HMAC, and PBKDF2. While it includes SHA-3 hashing, it is more general-purpose and not specifically focused on the Keccak algorithm.
The js-sha3 package is a lightweight library that provides an implementation of the SHA-3 (Keccak) hash functions. It is similar to keccak in that it focuses on the Keccak algorithm, but it is designed to be minimalistic and easy to use in web applications. It supports various SHA-3 hash lengths, including SHA3-224, SHA3-256, SHA3-384, and SHA3-512.
This module provides native bindings to Keccak sponge function family from Keccak Code Package. In browser pure JavaScript implementation will be used.
You can use this package as node Hash.
const createKeccakHash = require('keccak')
console.log(createKeccakHash('keccak256').digest().toString('hex'))
// => c5d2460186f7233c927e7db2dcc703c0e500b653ca82273b7bfad8045d85a470
console.log(createKeccakHash('keccak256').update('Hello world!').digest('hex'))
// => ecd0e108a98e192af1d2c25055f4e3bed784b5c877204e73219a5203251feaab
Also object has two useful methods: _resetState
and _clone
const createKeccakHash = require('keccak')
console.log(createKeccakHash('keccak256').update('Hello World!')._resetState().digest('hex'))
// => c5d2460186f7233c927e7db2dcc703c0e500b653ca82273b7bfad8045d85a470
const hash1 = createKeccakHash('keccak256').update('Hello')
const hash2 = hash1._clone()
console.log(hash1.digest('hex'))
// => 06b3dfaec148fb1bb2b066f10ec285e7c9bf402ab32aa78a5d38e34566810cd2
console.log(hash1.update(' world!').digest('hex'))
// => throw Error: Digest already called
console.log(hash2.update(' world!').digest('hex'))
// => ecd0e108a98e192af1d2c25055f4e3bed784b5c877204e73219a5203251feaab
I thought it will be popular question, so I decide write explanation in readme.
I know a few popular packages on npm related with Keccak:
This library is free and open-source software released under the MIT license.
FAQs
Keccak sponge function family
The npm package keccak receives a total of 798,343 weekly downloads. As such, keccak popularity was classified as popular.
We found that keccak demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.