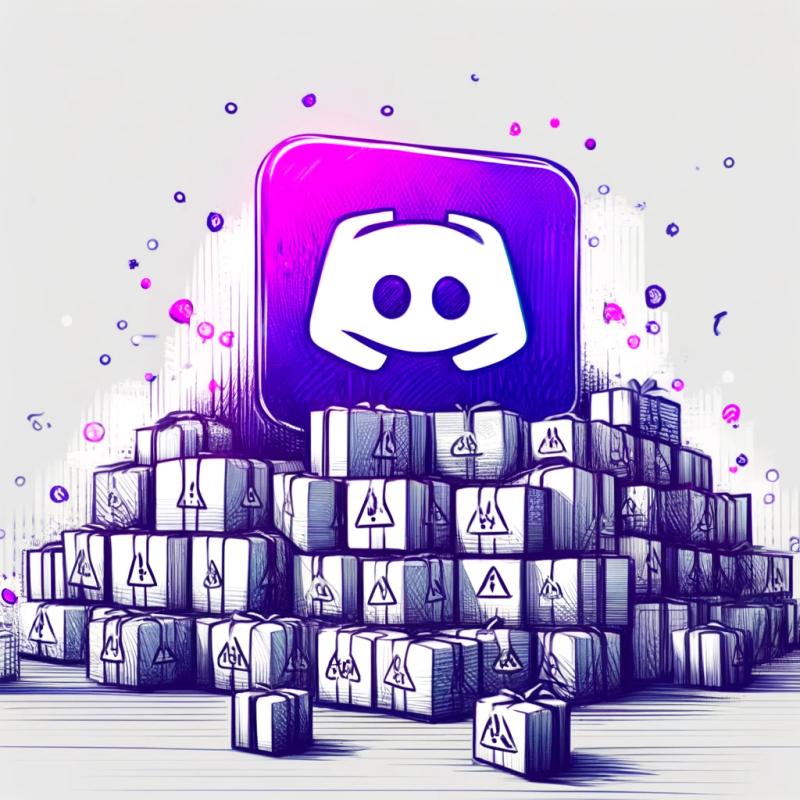
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
key-definitions
Advanced tools
Readme
Key-definitions module is made by one purpose: to have key definitions in one place. Key definitions are followed by MDN documentation and as recommended a web developers should check event.key
on keyup
event to detect pressed key (not a keydown
event nor keyCode
property). The reason for this read more in documentation.
Because there are numerous keyboard layouts this library is not 100% bulletproof.
Defined layouts follow QWERTY keyboards.
Before
const onKeyUpHandler = (event) => {
if (event.keyCode === 65) {
// this approach is deprecated by MDN
}
if (event.key === "ArrowLeft") {
// check if pressed key is arrow left
}
if (event.key === " ") {
// check if the key represents space
}
if (event.key === "A" || event.key === "B" || event.key === "C") {
// check if event key is one of the
}
};
useEffect(() => {
document.addEventListener("keyup", onKeyUpHandler);
return () => {
document.removeEventListener("keyup", onKeyUpHandler);
};
}, []);
Now
With new approach you can easily:
import { UpperCase, ARROW_LEFT, SPACE } from "key-definitions";
const onKeyUpHandler = (event) => {
if (compare(event, ARROW_LEFT)) {
// you can check if given event represents space
}
if (event.key === ARROW_LEFT.key) {
// you can check value by _key_ property
}
if (compare(event, [UpperCase.A, UpperCase.B, UpperCase.C])) {
// check if any character is matching with event key
}
};
useEffect(() => {
document.addEventListener("keyup", onKeyUpHandler);
return () => {
document.removeEventListener("keyup", onKeyUpHandler);
};
}, []);
npm install key-definitions
or
yarn add key-definitions
Each key inherits KeyInterface
that has following structure:
interface KeyInterface {
keyCode: number | number[]; // event.keyCode, it can be an array in case when numerous supports for different operation systems exists
keyCodeDefinitions?: KeyCodeSupportOS[]; // description of keyCode in case if multiple keyCode values exists
hex?: hexNumber | hexNumber[]; // key's hex number, it's array if multiple keyCodeDefinitions exists
code: string; // the same as event.code
key: string; // the same as event.key
isAltKey?: boolean;
isMetaKey?: boolean;
isShiftKey?: boolean;
isCtrlKey?: boolean;
}
Name | Description | Return value |
---|---|---|
isCharacter | Detect if pressed value is a character a-z and A-Z. | boolean |
compare | compare two values; compares key property and code in case of SHIFT, ALT and CONTROL keyboard events | boolean |
Compare event with key definitions from library.
import { SPACE } from "key-definitions";
const onKeyUp = (e: KeyboardEvent) => {
compare(event, SPACE); // true
};
Compare does event is one of the several key definitions from library.
import { SPACE, TAB } from "key-definitions";
const onKeyUp = (e: KeyboardEvent) => {
compare(e.key, [SPACE, TAB]); // true
};
Function will check key
, code
or keyCode
values of KeyboardEvent.
const onKeyUp = (e: KeyboardEvent) => {
isCharacter(e); // you can forward KeyboardEvent to detect if pressed key is a character
};
document.addEventListener("keyup", onKeyUp);
isCharacter("A"); // true
isCharacter("a"); // true
isCharacter("?"); // false
isCharacter("č"); // true
isCharacter("1"); // false
isCharacter("KeyA"); // true
isCharacter("KeyZ"); // true
isCharacter("Enter"); // false
Even keyCode
is deprecated it's still in usage by some developers. Forward keyCode
value from keyup
event.
isCharacter(64); // check 'a' value --> true
isCharacter(90); // check 'Z' value --> true
isCharacter(191); // check '?' value --> false
isCharacter(49); // check '1' value --> false
Language | Layout | FileName |
---|---|---|
English | US | - |
Croatian | CRO | Layout_CRO |
German | DE | Layout_DE |
By default US layout is set, for different layout you need to import it independently.
import { LowerCase }, Layout_CRO, Layout_DE from "key-definitions";
console.log(LowerCase.A.key); // --> returns US layout --> value 'a'
console.log(Layout_CRO.LowerCase.A.key); // --> returns croatian layout --> value 'a'
console.log(Layout_CRO.LowerCase.Č.key); // --> returns croatian layout --> value 'č'
console.log(LowerCase.Č.key); // --> undefined (not supported for US layout)
console.log(Layout_DE.LowerCase.Ü.key); // --> returns german layout ---> value 'ü'
console.log(Layout_DE.LowerCase.UMLAUT_U.key); // --> returns german layout ---> value 'ü'
Includes lower and upper case a-zA-Z
.
Key | Layout |
---|---|
A-Z | all |
Č | cro |
Ć | cro |
Ž | cro |
Đ | cro |
Š | cro |
Ü (or UMLAUT_U) | de |
Ö (or UMLAUT_O) | de |
Ä (or UMLAUT_A) | de |
ẞ (or UMLAUT_S) | de |
import { LowerCase, UpperCase } from "key-definitions";
console.log(LowerCase.A.key); // --> returns value 'a'
console.log(UpperCase.A.key); // --> returns value 'A'
Includes all numerical keys 0-9
in alphanumeric keyboard section.
Key | Name |
---|---|
0 | ZERO |
1 | ONE |
2 | TWO |
3 | THREE |
4 | FOUR |
5 | FIVE |
6 | SIX |
7 | SEVEN |
8 | EIGHT |
9 | NINE |
import { ZERO, SEVEN } from "key-definitions";
console.log(ZERO.key); // returns value '0'
console.log(SEVEN.key); // returns value '7'
Includes all keys in numpad section - digits and general (functional keys such as enter, num lock). Example: 0-9, NumLock, Enter
; We have two sections General
(functional keys) and Digits
(0-9 numbers).
Key | Name |
---|---|
0-9 | ZERO-NINE |
= (equal sign) | EQUAL |
enter | ENTER |
arrow up | ARROW_UP |
arrow down | ARROW_DOWN |
arrow right | ARROW_RIGHT |
arrow left | ARROW_LEFT |
* | MULTIPLY |
+ | ADD |
- | SUBSTRACT |
, | COMMA |
/ | DIVIDE |
. | DECIMAL |
insert | INSERT |
end | END |
page down | PAGE_DOWN |
home | HOME |
page up | PAGE_UP |
delete | DELETE |
import { Numpad } from "key-definitions";
console.log(Numpad.ARROW_DOWN.key); // returns value 'ArrowDown'
console.log(Numpad.ZERO.key); // returns '0'
Includes all keys from F1-F12
in function section.
import { F1 } from "key-definitions";
console.log(F1.key); // returns value 'F1'
Includes functional keys in alphanumeric keyboard section. Example: AltLeft, Shift, Enter, Tab, CapsLock
.
Key | Name |
---|---|
tab | TAB |
enter | ENTER |
shift left | SHIFT_LEFT |
shift right | SHIFT RIGHT |
ctrl left | CTRL_LEFT |
ctrl right | CTRL_RIGHT |
alt left | ALT_LEFT |
alt right | ALT_RIGHT |
caps lock | CAPS_LOCK |
escape | ESC |
space | SPACE |
page up | PAGE_UP |
page down | PAGE_DOWN |
end | END |
home | HOME |
arrow left | ARROW_LEFT |
arrow up | ARROW_UP |
arrow right | ARROW_RIGHT |
arrow down | ARROW_DOWN |
print screen | PRINT_SCREEN |
insert | INSERT |
delete | DELETE |
num lock | NUM_LOCK |
scroll lock | SCROLL_LOCK |
help | HELP |
pause | PAUSE |
import { ALT_LEFT, ALT_RIGHT } from "key-definitions";
console.log(ALT_LEFT.key); // returns value 'Alt'
console.log(ALT_LEFT.code); // returns value 'AltLeft'
console.log(ALT_RIGHT.key); // returns 'Alt'
console.log(ALT_RIGHT.code); // returns 'AltRight'
Includes special keys like question marks )!?.$&%/#"\*)
as part of alphanumeric keyboard section.
Key | Name |
---|---|
! | EXCLAMATION_MARK |
" | QUOTATION_MARK |
# | HASH |
$ | DOLLAR_SIGN |
% | PERCENT |
& | AMPERSAND |
' | APOSTROPHE |
( | OPEN_PARENTHESIS |
) | CLOSE_PARENTHESIS |
* | ASTERISK |
+ | PLUS |
, | COMMA |
- | DASH |
. | DOT |
/ | SLASH |
: | COLON |
; | SEMICOLON |
< | LESS_THAN_BRACKET |
= | EQUAL |
> | GREATER_THAN_BRACKET |
? | QUESTION_MARK |
@ | AMPERSAT |
[ | OPEN_BRACKET |
\ | BACKSLASH |
] | CLOSE_BRACKET |
^ | CARET |
_ | UNDERSCORE |
` | BACKQUOTE |
{ | OPEN_BRACE |
| | VERTICAL_BAR |
} | CLOSE_BRACE |
~ | TILDA |
import { DOLLAR_SIGN } from "key-definitions";
console.log(DOLLAR_SIGN.key); // returns value '$'
https://www.computerhope.com/jargon/s/specchar.htm
https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/code
https://developer.mozilla.org/en-US/docs/Web/API/KeyboardEvent/keyCode
FAQs
get a keyboard key definition from event
The npm package key-definitions receives a total of 18 weekly downloads. As such, key-definitions popularity was classified as not popular.
We found that key-definitions demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.