Comparing version 1.0.6 to 1.0.7
98
index.js
#!/usr/bin/env node | ||
const fs = require("fs"); | ||
const clear = require("clear"); | ||
const chalk = require("chalk"); | ||
const figlet = require("figlet"); | ||
const bar = require("cli-progress"); | ||
const cli = require("commander"); | ||
const inquirer = require("inquirer"); | ||
const { templates, questions } = require("./lib/constants"); | ||
const { helper } = require("./lib/help"); | ||
const { generateTemplate } = require("./lib/generate") | ||
const path = require("path"); | ||
clear(); | ||
const keza = new cli.Command(); | ||
console.log(chalk.green(figlet.textSync("Keza", { horizontalLayout: "full" }))); | ||
const progress = new bar.SingleBar({ | ||
format: "Done |" + chalk.magenta("{bar}") + "| {percentage}%", | ||
barCompleteChar: "\u2588", | ||
hideCursor: true | ||
}); | ||
if (fs.existsSync("package.json")) { | ||
helper(); | ||
process.argv.slice(2).forEach(function(item) { | ||
if (item === "-b" || item === "--babel") { | ||
generate(__dirname + "/templates/" + ".babelrc", ".babelrc"); | ||
} else if (item === "-d" || item === "--docker") { | ||
generate(__dirname + "/templates/" + ".dockerignore", ".dockerignore"); | ||
generate(__dirname + "/templates/" + "Dockerfile", "Dockerfile"); | ||
} else if (item === "-e" || item === "--eslint") { | ||
generate(__dirname + "/templates/" + ".eslintrc", ".eslintrc"); | ||
generate(__dirname + "/templates/" + ".eslintignore", ".eslintignore"); | ||
} else if (item === "-g" || item === "--gitignore") { | ||
generate(__dirname + "/templates/" + "gitignore", ".gitignore"); | ||
} else if (item === "-ho" || item === "--hound") { | ||
generate(__dirname + "/templates/" + ".hound.yml", ".hound.yml"); | ||
} else if (item === "-r" || item === "--readme") { | ||
generate(__dirname + "/templates/" + "README.md", "README.md"); | ||
} else if (item === "-s" || item === "--sequelizerc") { | ||
generate(__dirname + "/templates/" + ".sequelizerc", ".sequelizerc"); | ||
} else if (item === "-t" || item === "--travis") { | ||
generate(__dirname + "/templates/" + ".travis.yml", ".travis.yml"); | ||
} | ||
}); | ||
if (process.argv.length == 2) { | ||
fs.readdir(__dirname + "/templates", function(err, filenames) { | ||
if (err) { | ||
return; | ||
} | ||
progress.start(filenames.length); | ||
filenames.forEach(file => { | ||
generate(__dirname + "/templates/" + file, file); | ||
progress.update(filenames.length); | ||
helper(); | ||
console.log(chalk.magenta(figlet.textSync("Keza", { horizontalLayout: "full" }))); | ||
console.log(chalk.green("generate template files very fast")); | ||
if (process.argv.length == 2) { | ||
inquirer.prompt(questions).then((answers) => { | ||
let choices = []; | ||
Object.keys(answers).forEach((a) => { | ||
Object.keys(templates).forEach((t) => { | ||
if (a === t && answers[a].length >= 1) { | ||
answers[a].map((file) => { | ||
choices = [...choices, templates[a][file]]; | ||
}); | ||
} | ||
}); | ||
progress.stop(); | ||
}); | ||
} | ||
function generate(from, to) { | ||
progress.start(to.length); | ||
fs.copyFile(from, to, err => { | ||
progress.update(100); | ||
if (err) throw err; | ||
progress.stop(); | ||
choices.forEach((choice) => { | ||
generateTemplate(choice, path.basename(choice)); | ||
}); | ||
} | ||
} else { | ||
helper(); | ||
console.log( | ||
chalk.magenta("You have to initialize your project with npm or yarn first") | ||
); | ||
process.exit(); | ||
} | ||
function helper() { | ||
keza | ||
.version("1.0.4") | ||
.option("-b, --babel", "generate babelrc") | ||
.option("-d, --docker", "generate docker file") | ||
.option("-e, --eslint", "generate eslintrc and eslintignore") | ||
.option("-g, --gitignore", "generate nodejs gitignore") | ||
.option("-ho, --hound", "generate hound file") | ||
.option("-r, --readme", "generate readme file") | ||
.option("-s, --sequelize", "generate sequerizerc") | ||
.option("-t, --travis", "generate travis") | ||
.parse(process.argv); | ||
keza.on("--help", function() { | ||
console.log("Examples:"); | ||
console.log(" $ keza --help"); | ||
console.log(" $ keza -h"); | ||
console.log(" $ keza -b -d -t"); | ||
}); | ||
} |
{ | ||
"name": "keza", | ||
"version": "1.0.6", | ||
"description": "Generate nodejs CI template files, Eslint config files, gitignore and readme file from the command line when keza's CLI is installed globally", | ||
"version": "1.0.7", | ||
"description": "Generate template files(Eslint config files, gitignore, readme..etc) from the command line", | ||
"main": "index.js", | ||
@@ -11,21 +11,18 @@ "bin": { | ||
"lint": "eslint .", | ||
"coverage": "nyc report --reporter=text-lcov | coveralls", | ||
"test": "nyc mocha ./test/* --exit", | ||
"test": "exit 0", | ||
"fix-lint": "eslint . --fix" | ||
}, | ||
"devDependencies": { | ||
"chai": "^4.2.0", | ||
"coveralls": "3.0.9", | ||
"eslint": ">=6.7.2", | ||
"eslint-config-airbnb-base": "14.0.0", | ||
"eslint-plugin-import": "2.18.2", | ||
"mocha": "^6.2.2", | ||
"nyc": "^14.1.1" | ||
"eslint": "^6.8.0", | ||
"eslint-config-airbnb-base": "^14.1.0", | ||
"eslint-plugin-import": "^2.20.2" | ||
}, | ||
"dependencies": { | ||
"chalk": "^3.0.0", | ||
"chalk": "^4.0.0", | ||
"clear": "^0.1.0", | ||
"cli-progress": "^3.4.0", | ||
"commander": "^4.0.1", | ||
"figlet": "^1.2.4" | ||
"cli-progress": "^3.6.1", | ||
"commander": "^5.0.0", | ||
"figlet": "^1.3.0", | ||
"inquirer": "^7.1.0", | ||
"ora": "^4.0.3" | ||
}, | ||
@@ -36,3 +33,8 @@ "repository": { | ||
}, | ||
"keywords": [], | ||
"keywords": [ | ||
"generate", | ||
"generate templates", | ||
"template", | ||
"template files" | ||
], | ||
"author": "Igwaneza Bruce <knowbeeinc@gmail.com>", | ||
@@ -39,0 +41,0 @@ "license": "MIT", |
@@ -10,3 +10,3 @@ # Keza | ||
Generate nodejs CI template files, Eslint config files, gitignore, docker and readme file from the command line when keza's CLI is installed globally | ||
Generate template files(babelrc, eslint, readme, etc...) from the command line | ||
@@ -27,3 +27,5 @@ ## Install | ||
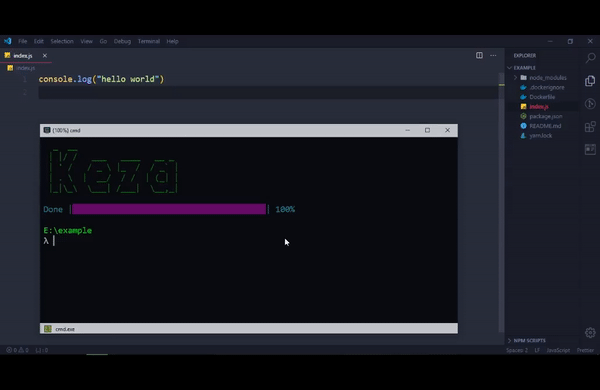 | ||
<p> | ||
<img src="https://raw.githubusercontent.com/knowbee/hosting/master/assets/keza.PNG" width="400px" height="auto" hspace="10"/> | ||
</p> | ||
@@ -35,3 +37,3 @@ ## Using Keza CLI | ||
```cli | ||
$ keza <options> | ||
$ keza | ||
``` | ||
@@ -41,22 +43,29 @@ | ||
- `-h` or `--help`: display helper screen. | ||
- `-b` or `--babel`: generate .babelrc. | ||
- `-d` or `--docker`: generate docker file. | ||
- `-e` or `--eslint`: generate .eslintrc and .eslintignore file. | ||
- `-g` or `--gitignore`: generate nodejs .gitignore. | ||
- `-ho` or `--hound`: generate hound yml file. | ||
- `-r` or `--readme`: generate readme file. | ||
- `-s` or `--sequelize`: generate .sequerizerc. | ||
- `-t` or `--travis`: generate travis yml file. | ||
- `--help`: display helper screen. | ||
## Contribution | ||
- Please before making a PR, read first this [Contributing Guideline](./CONTRIBUTING.md) | ||
Please before making a PR, read first this [Contributing Guideline](./CONTRIBUTING.md) | ||
## License | ||
MIT License | ||
MIT | ||
Copyright (c) 2020 Igwaneza Bruce <knowbeeinc@gmail.com> | ||
## Author | ||
Permission is hereby granted, free of charge, to any person obtaining a copy | ||
of this software and associated documentation files (the "Software"), to deal | ||
in the Software without restriction, including without limitation the rights | ||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell | ||
copies of the Software, and to permit persons to whom the Software is | ||
furnished to do so, subject to the following conditions: | ||
Igwaneza Bruce | ||
The above copyright notice and this permission notice shall be included in all | ||
copies or substantial portions of the Software. | ||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR | ||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, | ||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE | ||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER | ||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, | ||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE | ||
SOFTWARE. |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
3
20
129
68
12160
7
1
+ Addedinquirer@^7.1.0
+ Addedora@^4.0.3
+ Addedansi-escapes@4.3.2(transitive)
+ Addedansi-styles@3.2.1(transitive)
+ Addedchalk@2.4.24.1.2(transitive)
+ Addedchardet@0.7.0(transitive)
+ Addedcli-cursor@3.1.0(transitive)
+ Addedcli-spinners@2.9.2(transitive)
+ Addedcli-width@3.0.0(transitive)
+ Addedclone@1.0.4(transitive)
+ Addedcolor-convert@1.9.3(transitive)
+ Addedcolor-name@1.1.3(transitive)
+ Addedcommander@5.1.0(transitive)
+ Addeddefaults@1.0.4(transitive)
+ Addedescape-string-regexp@1.0.5(transitive)
+ Addedexternal-editor@3.1.0(transitive)
+ Addedfigures@3.2.0(transitive)
+ Addedhas-flag@3.0.0(transitive)
+ Addediconv-lite@0.4.24(transitive)
+ Addedinquirer@7.3.3(transitive)
+ Addedis-interactive@1.0.0(transitive)
+ Addedlodash@4.17.21(transitive)
+ Addedlog-symbols@3.0.0(transitive)
+ Addedmimic-fn@2.1.0(transitive)
+ Addedmute-stream@0.0.8(transitive)
+ Addedonetime@5.1.2(transitive)
+ Addedora@4.1.1(transitive)
+ Addedos-tmpdir@1.0.2(transitive)
+ Addedrestore-cursor@3.1.0(transitive)
+ Addedrun-async@2.4.1(transitive)
+ Addedrxjs@6.6.7(transitive)
+ Addedsafer-buffer@2.1.2(transitive)
+ Addedsignal-exit@3.0.7(transitive)
+ Addedsupports-color@5.5.0(transitive)
+ Addedthrough@2.3.8(transitive)
+ Addedtmp@0.0.33(transitive)
+ Addedtslib@1.14.1(transitive)
+ Addedtype-fest@0.21.3(transitive)
+ Addedwcwidth@1.0.1(transitive)
- Removedcommander@4.1.1(transitive)
Updatedchalk@^4.0.0
Updatedcli-progress@^3.6.1
Updatedcommander@^5.0.0
Updatedfiglet@^1.3.0