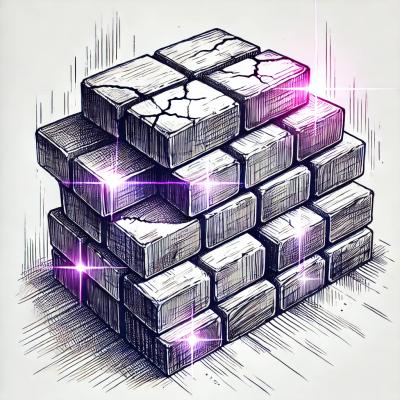
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
kitsu-core
Advanced tools
Simple, lightweight & framework agnostic JSON:API (de)serialsation components
Simple, lightweight & framework agnostic JSON:API (de)serialisation components
Migration guide for v9 & previous major releases
Package | Package Size* | Node | Chrome | Firefox | Safari | Edge |
---|---|---|---|---|---|---|
kitsu-core | ≤ 1.5 kb | 10+ | 83+ | 78+ | 13.1+ | 95+ |
* Minified with brotli
yarn add kitsu-core
npm install kitsu-core
import { camel } from 'kitsu-core' // ES Modules and Babel
const { camel } = require('kitsu-core') // CommonJS and Browserify
camel(...)
<!-- jsDelivr -->
<script src="https://cdn.jsdelivr.net/npm/kitsu-core"></script>
<!-- unpkg -->
<script src="https://unpkg.com/kitsu-core"></script>
kitsuCore.camel(...)
packages/kitsu-core/src/deattribute/index.js:29-51
Hoists attributes to be top-level
Deattribute an array of resources
// JSON:API 'data' field
const data = [
{
id: '1',
type: 'users',
attributes: { slug: 'wopian' }
}
]
const output = deattribute(data) // [ { id: '1', type: 'users', slug: 'wopian' } ]
Deattribute a resource
// JSON:API 'data' field
const data = {
id: '1',
type: 'users',
attributes: { slug: 'wopian' }
}
const output = deattribute(data) // { id: '1', type: 'users', slug: 'wopian' }
Returns (Object | Array<Object>) Deattributed resource data
packages/kitsu-core/src/deserialise/index.js:57-72
Deserialises a JSON-API response
response
Object The raw JSON:API response objectDeserialise with a basic data object
deserialise({
data: {
id: '1',
attributes: { liked: true }
},
meta: { hello: 'world' }
}) // { data: { id: '1', liked: true }, meta: { hello: 'world' } }
Deserialise with relationships
deserialise({
data: {
id: '1',
relationships: {
user: {
data: {
type: 'users',
id: '2' }
}
}
},
included: [
{
type: 'users',
id: '2',
attributes: { slug: 'wopian' }
}
]
}) // { data: { id: '1', user: { data: { type: 'users', id: '2', slug: 'wopian' } } } }
Returns Object The deserialised response
packages/kitsu-core/src/error/index.js:27-33
Uniform error handling for Axios, JSON:API and internal package errors. Mutated Error object is rethrown to the caller.
Error
Object The Errorerror('Hello')
error({errors: [ { code: 400 } ]})
error({
response: {
data: {
errors: [ {
title: 'Filter is not allowed',
detail: 'x is not allowed',
code: '102',
status: '400'
} ]
}
}
})
packages/kitsu-core/src/filterIncludes/index.js:33-46
Filters includes for the specific relationship requested
relationship
Object
const includes = [
{
id: '1',
type: 'users',
attributes: { name: 'Emma' }
},
{
id: '2',
type: 'users',
attributes: { name: 'Josh' }
}
]
const relationship = { id: '1', type: 'users' }
const response = filterIncludes(includes, relationship)
// {
// id: '1',
// type: 'users',
// attributes: { name: 'Emma' }
// }
Returns Array<Object> The matched includes
packages/kitsu-core/src/linkRelationships/index.js:97-117
Links relationships to included data
data
Object The response data objectincluded
Array<Object>? The response included object (optional, default []
)const data = {
attributes: { author: 'Joe' },
relationships: {
author: {
data: { id: '1', type: 'people' }
}
}
}
const included = [ {
id: '1',
type: 'people',
attributes: { name: 'Joe' }
} ]
const output = linkRelationships(data, included)
// {
// attributes: { author: 'Joe' },
// author: {
// data: { id: '1', name: 'Joe', type: 'people' }
// }
// }
Returns any Parsed data
packages/kitsu-core/src/query/index.js:33-44
Constructs a URL query string for JSON:API parameters
params
Object? Parameters to parseprefix
string? Prefix for nested parameters - used internally (optional, default null
)query({
filter: {
slug: 'cowboy-bebop',
title: {
value: 'foo'
}
}
sort: '-id'
})
// filter%5Bslug%5D=cowboy-bebop&filter%5Btitle%5D%5Bvalue%5D=foo&sort=-id
Returns string URL query string
packages/kitsu-core/src/serialise/index.js:249-260
Serialises an object into a JSON-API structure
type
string Resource type
data
(Object | Array<Object>)? The data (optional, default {}
)
method
string? Request type (PATCH, POST, DELETE) (optional, default 'POST'
)
options
Object? Optional configuration for camelCase and pluralisation handling (optional, default {}
)
options.camelCaseTypes
Function Convert library-entries and library_entries to libraryEntries (default no conversion). To use parameter, import camel from kitsu-core (optional, default s=>s
)options.pluralTypes
Function Pluralise types (default no pluralisation). To use parameter, import pluralize (or another pluralisation npm package) (optional, default s=>s
)Setting camelCaseTypes and pluralTypes options (example shows options used by kitsu
by default)
import { serialise, camel } from 'kitsu-core'
import pluralize from 'pluralize'
const model = 'anime'
const obj = { id: '1', slug: 'shirobako' }
// { data: { id: '1', type: 'anime', attributes: { slug: 'shirobako' } } }
const output = serialise(model, obj, 'PATCH', { camelCaseTypes: camel, pluralTypes: pluralize })
Basic usage (no case conversion or pluralisation)
import { serialise } from 'kitsu-core'
const model = 'anime'
const obj = { id: '1', slug: 'shirobako' }
// { data: { id: '1', type: 'anime', attributes: { slug: 'shirobako' } } }
const output = serialise(model, obj, 'PATCH')
Returns Object The serialised data
packages/kitsu-core/src/splitModel/index.js:29-39
Split model name from the model's resource URL
url
string URL path for the model
options
Object? Optional configuration for camelCase and pluralisation handling
options.resourceCase
Function Convert libraryEntries to library-entries or library_entries (default no conversion). To use parameter, import kebab or snake from kitsu-core (optional, default s=>s
)options.pluralModel
Function Pluralise models (default no pluralisation). To use parameter, import pluralize (or another pluralisation npm package) (optional, default s=>s
)splitModel('posts/1/comments')
// [ 'comments', 'posts/1/comments' ]
With pluralModel option
import plural from 'pluralize'
splitModel('posts/1/comment', { pluralModel: plural })
// [ 'comment', 'posts/1/comments' ]
With resourceCase option
import { kebab, snake } from 'kitsu-core'
splitModel('libraryEntries', { resourceCase: kebab })
// [ 'libraryEntries', 'library-entries' ]
splitModel('libraryEntries', { resourceCase: snake })
// [ 'libraryEntries', 'library_entries' ]
Returns [string, string] } Array containing the model name and the resource URL with pluralisation applied
packages/kitsu-core/src/camel/index.js:14-14
Converts kebab-case and snake_case into camelCase
input
string String to convertConvert kebab-case
camel('hello-world') // 'helloWorld'
Convert snake_case
camel('hello_world') // 'helloWorld'
Returns string camelCase formatted string
packages/kitsu-core/src/kebab/index.js:11-11
Converts camelCase into kebab-case
input
string camelCase stringkebab('helloWorld') // 'hello-world'
Returns string kebab-case formatted string
packages/kitsu-core/src/snake/index.js:11-11
Converts camelCase into snake_case
input
string camelCase stringsnake('helloWorld') // 'hello_world'
Returns string snake_case formatted string
See CONTRIBUTING
See CHANGELOG
All code released under MIT
FAQs
Simple, lightweight & framework agnostic JSON:API (de)serialsation components
The npm package kitsu-core receives a total of 6,250 weekly downloads. As such, kitsu-core popularity was classified as popular.
We found that kitsu-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.