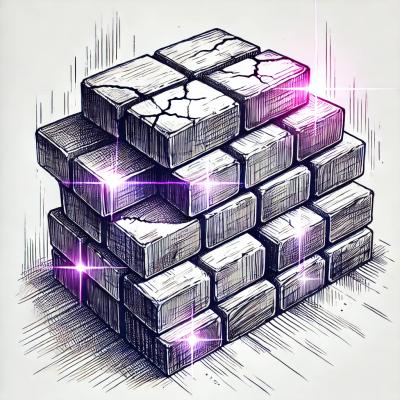
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
klark-js-plugins
Advanced tools
Plugin modules for KlarkJS
We are trying to create an ecosystem of utilities and functionalities that improve dramatically the automation of creating a robust API in NodeJS, ExpressJS and KlarkJS.
The main architecture that came up when we integrate a NodeJS API is the following:
Hopefully, express js is organized in middlewares. We created a collection of middlewares and utilities in order to automate the CRUD functionality. The CRUD model is based on the Mongoose models.
npm install --save klark-js-plugins
var modules = `plugins/**/index.js`;
var subModules = `plugins/**/*.module.js`;
// locate the klark plugins inside the node_modules folder
var klarkPlugins = `node_modules/klark-js-plugins/plugins/**/*.js`;
klark.run({
predicateFilePicker: function() {
return [
modules,
subModules,
klarkPlugins
]
}
});
Notice
If you want to include a subset of the plugins, you should include only the corresponding internal dependencies.
Let's assume that we only want to use the generators/create-user.module.js
. Our configuration should look like this:
var modules = `plugins/**/index.js`;
var subModules = `plugins/**/*.module.js`;
// locate only the necessary klark plugins inside the node_modules folder
var klarkPlugins = [
`node_modules/klark-js-plugins/plugins/generators/create-user.module.js`,
`node_modules/klark-js-plugins/plugins/models/user/index.js`
];
klark.run({
predicateFilePicker: function() {
return [
modules,
subModules,
klarkPlugins
]
}
});
Considering the following mongoose model:
var todoSchema = new $mongoose.Schema({
content: {type: String, maxlength: [16], required: true}
});
var todoModel = $mongoose.model('Todo', schema);
krkCrudGenerator creates and registers the CRUD functionality for a mongoose model.
KlarkModule(module, 'createSimpleTodoApi', function(krkCrudGenerator) {
var app = // express app
krkCrudGenerator(app, todoModel, {
apiUrlPrefix: 'v1'
});
});
Request
POST http://.../v1/todo
{
"content": "hi"
}
Response
401
{
"code": 4001,
"msg": "unauthorized user"
}
Request
HEADER Authorization: JWT ...
POST http://.../v1/todo
{
"content": "hiiiiiiiiiiiiiiii"
}
Response
400
{
"code": 1003,
"msg": "invalid params, 'content' length"
}
KlarkModule(module, 'createCustomTodoApi', function(krkCrudGenerator) {
var app = // express app
app.get(crudUrls.retrieveAll('Application'), [
// everybody can access this route
krkMiddlewarePermissions.check('FREE'),
// check and sanitize all the necessary arguments like page, count etc
krkMiddlewareParameterValidator.crud.retrieveAll(modelsApplication),
// retrieves the records from the MongoDB
middlewareRetrieveAllController,
// response
krkMiddlewareResponse.success
]);
});
Name: krkCrudGenerator
Path: /plugins/crud-generator/index.js
Dependencies: lodash, krkMiddlewareParameterValidator, krkMiddlewarePermissions, krkMiddlewareCrudController, krkMiddlewareResponse, krkCrudGeneratorUrls
Name: krkCrudGeneratorUrls
Path: /plugins/crud-generator/urls.module.js
Name: krkDbMongooseBinders
Path: /plugins/db/mongoose-binders/index.js
Dependencies: lodash, mongoose, krkLogger, krkModelsApp
Name: krkDbMongooseConnector
Path: /plugins/db/mongoose-connector/index.js
Dependencies: q, mongoose, krkLogger
Name: krkDbMongoosePluginsPassword
Path: /plugins/db/mongoose-plugins/password.module.js
Dependencies: lodash, q, bcrypt, krkLogger
Name: krkErrors
Path: /plugins/errors/index.js
Dependencies: lodash, krkLogger
Name: krkGeneratorsCreateUser
Path: /plugins/generators/create-user.module.js
Dependencies: mongoose, krkModelsUser
Name: krkGeneratorsLogin
Path: /plugins/generators/login.module.js
Dependencies: krkMiddlewarePermissions
Name: krkLogger
Path: /plugins/logger/index.js
Name: krkMiddlewareCrudController
Path: /plugins/middleware/crud-controller/index.js
Dependencies: lodash, q, krkDbMongooseBinders
Name: krkMiddlewareInitiateResponseParams
Path: /plugins/middleware/initiate-response-params/index.js
Dependencies: lodash, krkLogger, krkErrors
Name: krkMiddlewareParameterValidator
Path: /plugins/middleware/parameter-validator/index.js
Dependencies: q, lodash, krkParameterValidator
Name: krkMiddlewarePermissionsAuthorizeStrategy
Path: /plugins/middleware/permissions/authorize-strategy.module.js
Dependencies: lodash, passport-jwt, krkModelsUser
Name: krkMiddlewarePermissions
Path: /plugins/middleware/permissions/index.js
Dependencies: lodash, passport, jwt-simple, krkLogger, krkMiddlewarePermissionsRoles
Name: krkMiddlewarePermissionsRoles
Path: /plugins/middleware/permissions/roles.module.js
Name: krkMiddlewareResponse
Path: /plugins/middleware/response/index.js
Dependencies: lodash
Name: krkModelsApp
Path: /plugins/models/app/index.js
Dependencies: mongoose
Name: krkModelsUser
Path: /plugins/models/user/index.js
Dependencies: lodash, q, mongoose, mongoose-type-email, mongoose-createdmodified, krkMiddlewarePermissionsRoles, krkDbMongoosePluginsPassword
Name: krkNotificationsEmail
Path: /plugins/notifications/email.module.js
Dependencies: lodash, q, nodemailer
Name: krkParameterValidator
Path: /plugins/parameter-validator/index.js
Dependencies: q, lodash, express-validator
Name: krkPromiseExtension
Path: /plugins/promise-extension/index.js
Dependencies: lodash
Name: krkRouter
Path: /plugins/router/index.js
Dependencies: express
Name: krkRoutesAuthorize
Path: /plugins/routers/authorize/index.js
Dependencies: lodash, q, crypto, krkLogger, krkDbMongooseBinders, krkRoutersAuthorizeVerifyAccountEmailTmpl, krkNotificationsEmail, krkMiddlewareResponse, krkParameterValidator, krkMiddlewarePermissions, krkModelsUser
Name: krkRoutersAuthorizeVerifyAccountEmailTmpl
Path: /plugins/routers/authorize/verify-account-email-tmpl.module.js
Dependencies: config
Name: krkRoutesMultimedia
Path: /plugins/routers/multimedia/index.js
Dependencies: q, lodash, fs, multer, crypto, mkdirp, krkMiddlewarePermissions, krkMiddlewareResponse
Name: krkRoutesServerInfo
Path: /plugins/routers/server-info/index.js
Dependencies: krkMiddlewareResponse
Name: krkRoutesUsers
Path: /plugins/routers/users/index.js
Dependencies: crypto, q, lodash, krkModelsUser, krkRoutersAuthorizeVerifyAccountEmailTmpl, krkParameterValidator, krkNotificationsEmail, krkMiddlewareResponse, krkMiddlewareCrudController, krkMiddlewarePermissions
Name: krkServer
Path: /plugins/server/index.js
Name: krkUtilitiesDate
Path: /plugins/utilities/date.module.js
FAQs
Plugin modules for KlarkJS
The npm package klark-js-plugins receives a total of 4 weekly downloads. As such, klark-js-plugins popularity was classified as not popular.
We found that klark-js-plugins demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.