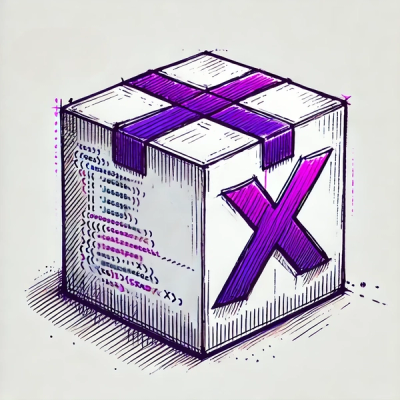
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
koa-error-mapper
Advanced tools
koa-error-mapper
is a middleware that handles application errors by mapping them to a custom format.
Install the package via yarn
:
❯ yarn add koa-error-mapper
or via npm
:
❯ npm install koa-error-mapper --save
Mappers are responsible for handling errors and normalizing the response. A generic fallback mapper is included in case no custom mapper is available.
The only interface for a mapper is a map()
function. A mapper should return undefined
if it is not capable or responsible for mapping a specific error. It must return an object with status
and body
. If not returned on the object, the value on the response will be undefined
for precaution. It may also contain a headers
property.
module.exports = {
map(e) {
if (!(e instanceof CustomError)) {
return;
}
// Add your own custom logic here.
return { status: e.code, body: { message: e.message }, headers: { Foo: 'Bar' }};
}
};
Now use the error mapper and register CustomMapper
:
'use strict';
const CustomError = require('path/to/my/custom/error');
const Koa = require('koa');
const app = new Koa();
const customMapper = require('path/to/my/custom/mapper');
app.use(errorMapper([customMapper]);
app.get('/', async () => {
throw new CustomError(401, 'Ah-ah!');
});
app.listen(3000);
Result:
GET /
HTTP/1.1 401 Unauthorized
Foo: Bar
{ "message": "Ah-ah!" }
❯ yarn test
❯ npm version [<new version> | major | minor | patch] -m "Release %s"
MIT
FAQs
An error mapper for Koa applications
The npm package koa-error-mapper receives a total of 2,646 weekly downloads. As such, koa-error-mapper popularity was classified as popular.
We found that koa-error-mapper demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.