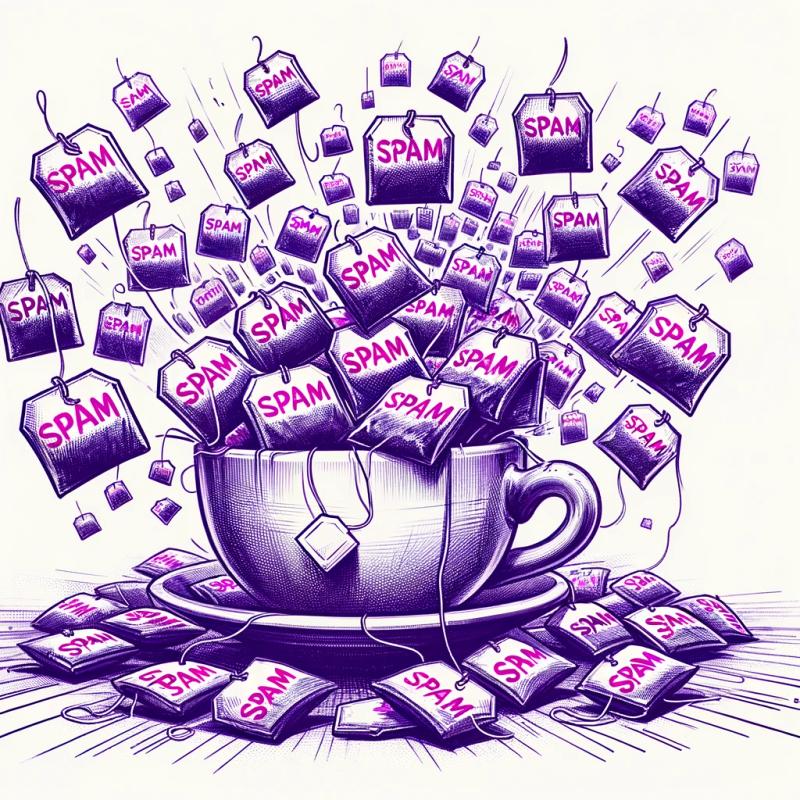
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
koa-static
Advanced tools
Readme
Koa static file serving middleware, wrapper for koa-send
.
$ npm install koa-static
const Koa = require('koa');
const app = new Koa();
app.use(require('koa-static')(root, opts));
root
root directory string. nothing above this root directory can be servedopts
options object.maxage
Browser cache max-age in milliseconds. defaults to 0hidden
Allow transfer of hidden files. defaults to falseindex
Default file name, defaults to 'index.html'defer
If true, serves after return next()
, allowing any downstream middleware to respond first.gzip
Try to serve the gzipped version of a file automatically when gzip is supported by a client and if the requested file with .gz extension exists. defaults to true.br
Try to serve the brotli version of a file automatically when brotli is supported by a client and if the requested file with .br extension exists (note, that brotli is only accepted over https). defaults to true.extensions
Try to match extensions from passed array to search for file when no extension is sufficed in URL. First found is served. (defaults to false
)const serve = require('koa-static');
const Koa = require('koa');
const app = new Koa();
// $ GET /package.json
app.use(serve('.'));
// $ GET /hello.txt
app.use(serve('test/fixtures'));
// or use absolute paths
app.use(serve(__dirname + '/test/fixtures'));
app.listen(3000);
console.log('listening on port 3000');
koa-static
to a specific pathMIT
FAQs
Static file serving middleware for koa
The npm package koa-static receives a total of 657,768 weekly downloads. As such, koa-static popularity was classified as popular.
We found that koa-static demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.