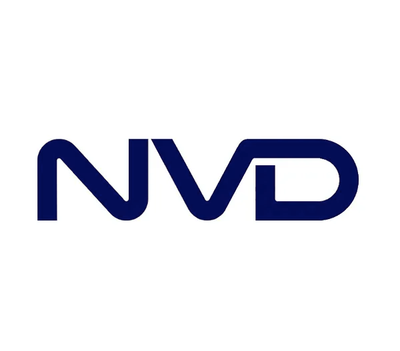
Security News
NIST Misses 2024 Deadline to Clear NVD Backlog
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Koa is a new web framework designed by the team behind Express, which aims to be a smaller, more expressive, and more robust foundation for web applications and APIs. Koa uses async functions to eliminate callback hell and simplify error handling. It does not bundle any middleware within its core, and it provides an elegant suite of methods that make writing servers fast and enjoyable.
HTTP Server
Koa can be used to create an HTTP server that listens on a given port. The example shows a basic server that responds with 'Hello World' to every request.
const Koa = require('koa');
const app = new Koa();
app.use(async ctx => {
ctx.body = 'Hello World';
});
app.listen(3000);
Middleware
Koa is known for its middleware stack that allows for more control over the request/response cycle. The example demonstrates a simple timing middleware that records how long a request takes to process.
const Koa = require('koa');
const app = new Koa();
app.use(async (ctx, next) => {
const start = Date.now();
await next();
const ms = Date.now() - start;
ctx.set('X-Response-Time', `${ms}ms`);
});
app.use(async ctx => {
ctx.body = 'Hello World';
});
app.listen(3000);
Error Handling
Koa provides a structured way to handle errors. In this example, middleware is used to catch and handle errors that may occur during request processing.
const Koa = require('koa');
const app = new Koa();
app.use(async (ctx, next) => {
try {
await next();
} catch (err) {
ctx.status = err.status || 500;
ctx.body = err.message;
ctx.app.emit('error', err, ctx);
}
});
app.on('error', (err, ctx) => {
console.error('server error', err, ctx);
});
app.listen(3000);
Context
Koa provides a context object encapsulating the Node's request and response objects into a single object which provides many helpful methods for writing web applications and APIs.
const Koa = require('koa');
const app = new Koa();
app.use(async ctx => {
ctx.body = `Request Type: ${ctx.method}`;
});
app.listen(3000);
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It is one of the most popular Node.js frameworks and has a large ecosystem of middleware available. Compared to Koa, Express is more established but relies on callback functions rather than async/await for handling asynchronous operations.
Hapi is a rich framework for building applications and services that allows developers to focus on writing reusable application logic instead of spending time building infrastructure. It is known for its powerful plugin system. Hapi is more configuration-driven compared to Koa's minimalistic and middleware-centric approach.
Fastify is a fast and low overhead web framework for Node.js. It is inspired by Hapi and Express and aspires to be faster than them. Fastify emphasizes performance and provides a robust plugin architecture. Unlike Koa, which is minimalist by design, Fastify comes with more built-in features.
Sails.js is a MVC framework for Node.js that is built on top of Express. It is designed to emulate the familiar MVC pattern of frameworks like Ruby on Rails, but with support for the requirements of modern apps: data-driven APIs with scalable, service-oriented architecture. Sails is more opinionated and includes more built-in features compared to Koa's minimalistic approach.
Expressive HTTP middleware framework for node.js to make web applications and APIs more enjoyable to write. Koa's middleware stack flows in a stack-like manner, allowing you to perform actions downstream then filter and manipulate the response upstream.
Only methods that are common to nearly all HTTP servers are integrated directly into Koa's small ~570 SLOC codebase. This includes things like content negotiation, normalization of node inconsistencies, redirection, and a few others.
Koa is not bundled with any middleware.
Koa requires node v7.6.0 or higher for ES2015 and async function support.
$ npm install koa
const Koa = require('koa');
const app = new Koa();
// response
app.use(ctx => {
ctx.body = 'Hello Koa';
});
app.listen(3000);
Koa is a middleware framework that can take two different kinds of functions as middleware:
Here is an example of logger middleware with each of the different functions:
app.use(async (ctx, next) => {
const start = Date.now();
await next();
const ms = Date.now() - start;
console.log(`${ctx.method} ${ctx.url} - ${ms}ms`);
});
// Middleware normally takes two parameters (ctx, next), ctx is the context for one request,
// next is a function that is invoked to execute the downstream middleware. It returns a Promise with a then function for running code after completion.
app.use((ctx, next) => {
const start = Date.now();
return next().then(() => {
const ms = Date.now() - start;
console.log(`${ctx.method} ${ctx.url} - ${ms}ms`);
});
});
The middleware signature changed between v1.x and v2.x. The older signature is deprecated.
Old signature middleware support will be removed in v3
Please see the Migration Guide for more information on upgrading from v1.x and using v1.x middleware with v2.x.
Each middleware receives a Koa Context
object that encapsulates an incoming
http message and the corresponding response to that message. ctx
is often used
as the parameter name for the context object.
app.use(async (ctx, next) => { await next(); });
Koa provides a Request
object as the request
property of the Context
.
Koa's Request
object provides helpful methods for working with
http requests which delegate to an IncomingMessage
from the node http
module.
Here is an example of checking that a requesting client supports xml.
app.use(async (ctx, next) => {
ctx.assert(ctx.request.accepts('xml'), 406);
// equivalent to:
// if (!ctx.request.accepts('xml')) ctx.throw(406);
await next();
});
Koa provides a Response
object as the response
property of the Context
.
Koa's Response
object provides helpful methods for working with
http responses which delegate to a ServerResponse
.
Koa's pattern of delegating to Node's request and response objects rather than extending them
provides a cleaner interface and reduces conflicts between different middleware and with Node
itself as well as providing better support for stream handling. The IncomingMessage
can still be
directly accessed as the req
property on the Context
and ServerResponse
can be directly
accessed as the res
property on the Context
.
Here is an example using Koa's Response
object to stream a file as the response body.
app.use(async (ctx, next) => {
await next();
ctx.response.type = 'xml';
ctx.response.body = fs.createReadStream('really_large.xml');
});
The Context
object also provides shortcuts for methods on its request
and response
. In the prior
examples, ctx.type
can be used instead of ctx.response.type
and ctx.accepts
can be used
instead of ctx.request.accepts
.
For more information on Request
, Response
and Context
, see the Request API Reference,
Response API Reference and Context API Reference.
The object created when executing new Koa()
is known as the Koa application object.
The application object is Koa's interface with node's http server and handles the registration of middleware, dispatching to the middleware from http, default error handling, as well as configuration of the context, request and response objects.
Learn more about the application object in the Application API Reference.
If you're not using node v7.6+
, we recommend setting up babel
with @babel/preset-env
:
$ npm install @babel/register @babel/preset-env @babel/cli --save-dev
In development, you'll want to use @babel/register
:
node --require @babel/register <your-entry-file>
In production, you'll want to build your files with @babel/cli
. Suppose you are compiling a folder src
and you wanted the output to go to a new folder dist
with non-javascript files copied:
babel src --out-dir dist --copy-files
And have your .babelrc
setup:
{
"presets": [
["@babel/preset-env", {
"targets": {
"node": true
}
}]
]
}
Check the Troubleshooting Guide or Debugging Koa in the general Koa guide.
$ npm test
To report a security vulnerability, please do not open an issue, as this notifies attackers of the vulnerability. Instead, please email dead_horse and jonathanong to disclose.
See AUTHORS.
Looking for a career upgrade?
Support us with a monthly donation and help us continue our activities.
Become a sponsor and get your logo on our README on Github with a link to your site.
FAQs
Koa web app framework
The npm package koa receives a total of 1,462,061 weekly downloads. As such, koa popularity was classified as popular.
We found that koa demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NIST has failed to meet its self-imposed deadline of clearing the NVD's backlog by the end of the fiscal year. Meanwhile, CVE's awaiting analysis have increased by 33% since June.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.