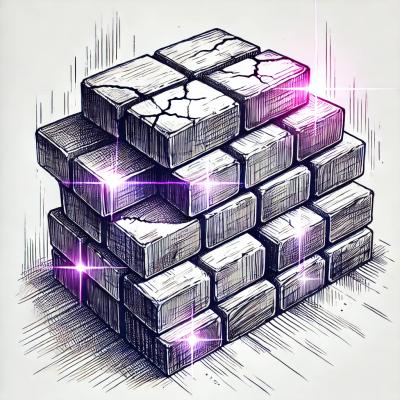
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
lambda-local
Advanced tools
The lambda-local npm package allows you to run and test AWS Lambda functions locally. This can be useful for development and debugging purposes, as it enables you to simulate the AWS Lambda environment on your local machine.
Run Lambda Function Locally
This feature allows you to execute a Lambda function locally by specifying the event, the path to the Lambda function, AWS credentials, and a timeout. The code sample demonstrates how to set up and run a Lambda function locally using lambda-local.
const lambdaLocal = require('lambda-local');
const path = require('path');
lambdaLocal.execute({
event: require('./event.json'),
lambdaPath: path.join(__dirname, 'index.js'),
profilePath: '~/.aws/credentials',
profileName: 'default',
timeoutMs: 3000
}).then(function(done) {
console.log(done);
}).catch(function(err) {
console.log(err);
});
Simulate AWS Context
This feature allows you to simulate the AWS context object that is passed to Lambda functions. The code sample shows how to define a custom context and use it when executing a Lambda function locally.
const lambdaLocal = require('lambda-local');
const path = require('path');
const context = {
functionName: 'myLambdaFunction',
awsRequestId: '1234567890',
logGroupName: '/aws/lambda/myLambdaFunction',
logStreamName: '2021/01/01/[$LATEST]abcdef1234567890'
};
lambdaLocal.execute({
event: require('./event.json'),
lambdaPath: path.join(__dirname, 'index.js'),
context: context,
timeoutMs: 3000
}).then(function(done) {
console.log(done);
}).catch(function(err) {
console.log(err);
});
Custom Environment Variables
This feature allows you to set custom environment variables for your Lambda function. The code sample demonstrates how to set an environment variable and use it when running a Lambda function locally.
const lambdaLocal = require('lambda-local');
const path = require('path');
process.env.MY_ENV_VAR = 'myValue';
lambdaLocal.execute({
event: require('./event.json'),
lambdaPath: path.join(__dirname, 'index.js'),
timeoutMs: 3000
}).then(function(done) {
console.log(done);
}).catch(function(err) {
console.log(err);
});
The serverless-offline package allows you to run Serverless applications and AWS Lambda functions locally. It integrates with the Serverless Framework and provides a more comprehensive local development environment, including support for API Gateway, DynamoDB, and other AWS services. Compared to lambda-local, serverless-offline offers a broader range of features and better integration with the Serverless Framework.
LocalStack is a fully functional local AWS cloud stack that allows you to develop and test cloud applications offline. It supports a wide range of AWS services, including Lambda, S3, DynamoDB, and more. Compared to lambda-local, LocalStack offers a more comprehensive local AWS environment, making it suitable for testing complex applications that rely on multiple AWS services.
Lambda-local lets you test Amazon Lambda functions on your local machine with sample event data.
The context
of the Lambda function is already loaded so you do not have to worry about it.
You can pass any event
JSON object as you please.
npm install -g lambda-local
You can use Lambda-local as a command line tool.
# Usage
lambda-local -l index.js -h handler -e event-samples/s3-put.js
You can also use Lambda local directly in a script. For instance, it is interesting in a mocha test suite in combination with istanbull in order to get test coverage.
const lambdaLocal = require('lambda-local');
var jsonPayload = {
'key1': 'value1',
'key2': 'value2',
'key3': 'value3'
}
lambdaLocal.execute({
event: jsonPayload,
lambdaPath: path.join(__dirname, 'path/to/index.js'),
profilePath: '~/.aws/credentials',
profileName: 'default',
timeoutMs: 3000,
callback: function(err, data) {
if (err) {
console.log(err);
} else {
console.log(data);
}
}
});
Event sample data are placed in event-samples
folder - feel free to use the files in here, or create your own event data.
Event data are just JSON objects exported:
// Sample event data
module.exports = {
foo: "bar"
};
The context
object has been directly extracted from the source visible when running an actual Lambda function on AWS.
They may change the internals of this object, and Lambda-local does not guarantee that this will always be up-to-date with the actual context object.
Since the Amazon Lambda can load the AWS-SDK npm without installation, Lambda-local has also packaged AWS-SDK in its dependencies. If you want to use this, please use the "-p" option with the aws credentials file. More infos here: http://docs.aws.amazon.com/cli/latest/userguide/cli-chap-getting-started.html#cli-config-files
execute(options)
Executes a lambda given the options
object where keys are:
event
- requested event as a json object,lambdaPath
- requested path to the lambda function,profilePath
- optional path to your AWS credentials fileprofileName
- optional aws profile namelambdaHandler
- optional handler name, default to handler
region
- optional AWS region, default to us-east-1
callbackWaitsForEmptyEventLoop
- optional, default to true
which forces the function to stop after having called the handler function even if context.done/succeed/fail was not called.timeoutMs
- optional timeout, default to 3000 msmute
- optional, allows to mute console.log calls in the lambda function, default falsecallback
- optional lambda third parameter callbackThis library is released under the MIT license.
FAQs
Commandline tool and API to run Lambda functions on your local machine.
We found that lambda-local demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.