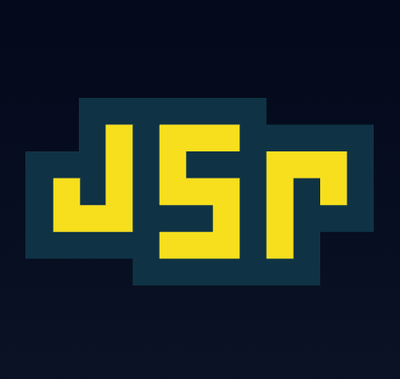
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
larvitdbmigration
Advanced tools
This is used to keep track of the database structure, and update it when need be via deploys.
At the moment only MariaDB(/MySQL) is supported.
A table by default called db_version will be created, containing a single integer.
Scripts will be placed by default in /dbmigration/.js
Each migration script will be ran, and the db_version increased, until no more migration scripts exists.
In your application startup script, do something like this:
'use strict';
var dbMigration = require('larvitdbmigration');
dbMigration({
'host': '127.0.0.1',
'user': 'bar',
'database': 'bar'
})(function(err) {
if (err) {
throw err;
}
// Now database is migrated and ready for use!
});
To use custom table name and/or script path, just change
dbMigration({
'host': '127.0.0.1',
'user': 'bar',
'password': 'bar',
'database': 'bar'
})(function(err) {
to
dbMigration({
'host': '127.0.0.1',
'user': 'bar',
'password': 'bar',
'database': 'bar',
'tableName': 'some_table',
'migrationScriptsPath': './scripts_yo'
})(function(err) {
Lets say the current database have a table like this:
CREATE TABLE bloj (nisse int(11));
And in the next deploy we'd like to change the column name "nisse" to "hasse". For this you can do one of two methods:
Create the file //1.js with this content:
'use strict';
var db = require('db');
exports = module.exports = function(cb) {
db.query('ALTER TABLE bloj CHANGE nisse hasse int(11);', cb);
}
IMPORTANT! SQL files will be ignored if a .js file exists.
ALSO IMPORTANT! SQL files require the mysql client to be installed on the host system.
Create the file //1.sql with this content:
ALTER TABLE bloj CHANGE nisse hasse int(11);
Tadaaa! Now this gets done once and the version will be bumped to 1. If you then create a script named "2.js" or "2.sql" you might guess what happends. :)
FAQs
node.js database migration tool
The npm package larvitdbmigration receives a total of 368 weekly downloads. As such, larvitdbmigration popularity was classified as not popular.
We found that larvitdbmigration demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.