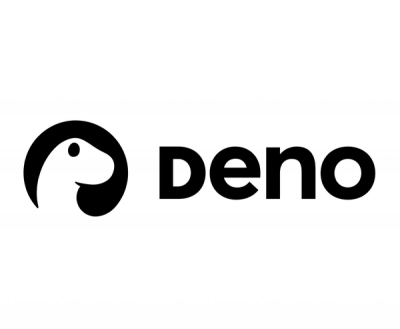
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Libraries.io minimalistic GitHub client.
const Client = require('libhub')
let github = new Client(token)
// GET
github.get(`/repos/librariesio/libhub/issues`)
.then( (issues) => {
console.log(issues)
})
// POST
github.post(`/repos/librariesio/libhub/issues`, {}, issueBody)
.then( (issue) => {
console.log(issue)
})
Available methods: get
, post
, patch
, put
, delete
// 3xx/4xx/5xx errors
github.get(`/repos/librariesio/${invalidId}`)
.then( (repo) => {
// This won't happen
})
.catch( (err) => {
console.log(err.statusCode) // 404
})
The Client constructor takes an optional cache object that should have a get
and a set
method. Both should return a Promise.
const Client = require('libhub')
const InMemoryCache = require('./test/cache')
let github = new Client(token, { cache: InMemoryCache })
github.get(`/repos/librariesio/libhub/issues`)
.then( (issues) => {
console.log(issues)
})
github.get(`/events`, { allPages: true })
.then( (events) => {
console.log(events.length) // All the user public events ~300
})
Well, simplicity. Most GitHub clients I used implement unnecessary abstractions. For example, octonode. This means that you need to check GitHub API documentation and Octonode documentation to perform any action. This gets boring pretty quickly.
With LibHub, you just need to lookup the resource URL and use it. Also, ES6 interpolation.
github.get(`/repos/${owner}/${repo}/branches/${branch}`)
MIT
FAQs
Libraries.io Simple GitHub Client
The npm package libhub receives a total of 2 weekly downloads. As such, libhub popularity was classified as not popular.
We found that libhub demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.