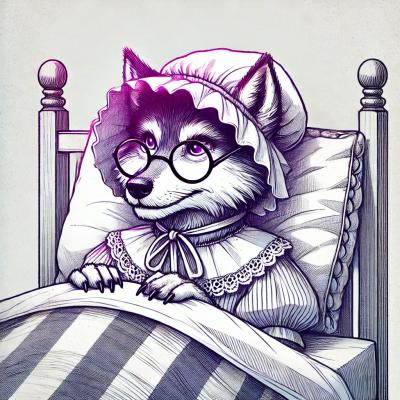
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
The libmime npm package is a utility library for handling MIME types. It provides functionalities for parsing and formatting MIME types, which are essential for handling file types and content negotiation in web and email development. With libmime, developers can easily determine the MIME type of a file, create MIME-encoded words, and parse MIME-encoded strings.
Determining MIME type from a filename
This feature allows you to get the MIME type of a file based on its extension. It's useful for setting Content-Type headers when serving files over HTTP.
const libmime = require('libmime');
const mimeType = libmime.getType('example.pdf');
console.log(mimeType); // 'application/pdf'
Getting a file extension from a MIME type
This functionality enables you to find the appropriate file extension for a given MIME type, which can be useful when generating files dynamically.
const libmime = require('libmime');
const extension = libmime.getExtension('application/pdf');
console.log(extension); // 'pdf'
Encoding and decoding MIME-encoded words
libmime can encode and decode MIME-encoded words, which is particularly useful for handling email headers that include characters outside the ASCII range.
const libmime = require('libmime');
const encodedWord = libmime.encodeWord('Hello äöü', 'Q');
const decodedWord = libmime.decodeWord(encodedWord);
console.log(encodedWord);
console.log(decodedWord);
The 'mime' package offers similar functionalities for determining MIME types and extensions. However, it focuses more on the mapping between file extensions and MIME types and does not include functionality for encoding and decoding MIME-encoded words.
Similar to 'mime', the 'mime-types' package provides utility functions for working with MIME types and file extensions. It is a lightweight alternative, offering a simple API for MIME type lookup and extension retrieval without the encoding/decoding capabilities of libmime.
The 'content-type' package is designed to parse and format HTTP Content-Type headers. While it deals with MIME types as part of its functionality, its focus is narrower, targeting HTTP header manipulation rather than the broader scope of MIME type handling offered by libmime.
libmime
provides useful MIME related functions. For Quoted-Printable and Base64 encoding and decoding see libqp and libbase64.
npm install libmime
var libmime = require('libmime');
Encodes a string into mime encoded word format.
libmime.encodeWord(str [, mimeWordEncoding[, maxLength]]) → String
Example
libmime.encodeWord('See on õhin test', 'Q');
Becomes with UTF-8 and Quoted-printable encoding
=?UTF-8?Q?See_on_=C3=B5hin_test?=
Decodes a string from mime encoded word format.
libmime.decodeWord(str) → String
Example
libmime.decodeWord('=?UTF-8?Q?See_on_=C3=B5hin_test?=');
will become
See on õhin test
Encodes non ascii sequences in a string to mime words.
libmime.encodeWords(str[, mimeWordEncoding[, maxLength]) → String
Decodes a string that might include one or several mime words. If no mime words are found from the string, the original string is returned
libmime.decodeWords(str) → String
Folds a long line according to the RFC 5322. Mostly needed for folding header lines.
libmime.foldLines(str [, lineLength[, afterSpace]]) → String
Example
libmime.foldLines('Content-Type: multipart/alternative; boundary="----zzzz----"')
results in
Content-Type: multipart/alternative;
boundary="----zzzz----"
Adds soft line breaks to content marked with format=flowed
options to ensure that no line in the message is never longer than lineLength.
libmime.encodeFlowed(str [, lineLength]) → String
Unwraps a plaintext string in format=flowed wrapping.
libmime.decodeFlowed(str [, delSp]) → String
Unfolds a header line and splits it to key and value pair. The return value is in the form of {key: 'subject', value: 'test'}
. The value is not mime word decoded, you need to do your own decoding based on the rules for the specific header key.
libmime.decodeHeader(headerLine) → Object
Parses a block of header lines. Does not decode mime words as every header might have its own rules (eg. formatted email addresses and such).
Return value is an object of headers, where header keys are object keys and values are arrays.
libmime.decodeHeaders(headers) → Object
Parses a header value with key=value
arguments into a structured object. Useful when dealing with
content-type
and such. Continuation encoded params are joined into mime encoded words.
parseHeaderValue(valueString) → Object
Example
parseHeaderValue('content-type: text/plain; CHARSET="UTF-8"');
Outputs
{
"value": "text/plain",
"params": {
"charset": "UTF-8"
}
}
Joins structured header value together as 'value; param1=value1; param2=value2'
buildHeaderValue(structuredHeader) → String
parseHeaderValue
filename
argument is encoded with continuation encoding if needed
Encodes and splits a header param value according to RFC2231 Parameter Value Continuations.
libmime.buildHeaderParam(key, str, maxLength) → Array
filename
)The method returns an array of encoded parts with the following structure: [{key:'...', value: '...'}]
Example
libmime.buildHeaderParam('filename', 'filename õäöü.txt', 20);
→
[ { key: 'filename*0*', value: 'utf-8\'\'filename%20' },
{ key: 'filename*1*', value: '%C3%B5%C3%A4%C3%B6' },
{ key: 'filename*2*', value: '%C3%BC.txt' } ]
This can be combined into a properly formatted header:
Content-disposition: attachment; filename*0*=utf-8''filename%20
filename*1*=%C3%B5%C3%A4%C3%B6; filename*2*=%C3%BC.txt
Returns file extension for a content type string. If no suitable extensions are found, 'bin' is used as the default extension.
libmime.detectExtension(mimeType) → String
Example
libmime.detectExtension('image/jpeg') // returns 'jpeg'
Returns content type for a file extension. If no suitable content types are found, 'application/octet-stream' is used as the default content type
libmime.detectMimeType(extension) → String
Example
libmime.detectExtension('logo.jpg') // returns 'image/jpeg'
MIT
v3.0.0 2016-12-08
FAQs
Encode and decode quoted printable and base64 strings
The npm package libmime receives a total of 1,239,166 weekly downloads. As such, libmime popularity was classified as popular.
We found that libmime demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.