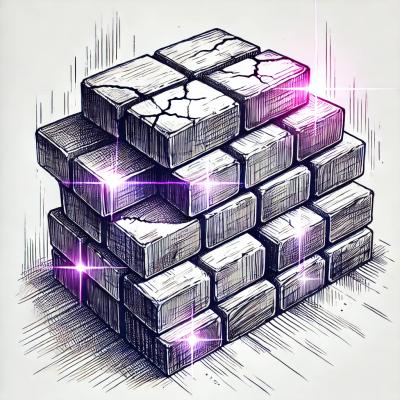
Security News
Input Validation Vulnerabilities Dominate MITRE's 2024 CWE Top 25 List
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
limit-once
Advanced tools
Create a once
function that caches the result of the first function call. limit-once
let's you lazily evaluate a value (using a function), and then hold onto the value forever.
[!NOTE] This package is still under construction
Features:
150B
)460B
)this
controlTypeScript
support# yarn
yarn add limit-once
# npm
npm install limit-once
# bun
bun add limit-once
import { once } from 'limit-once';
function getGreeting(name: string): string {
return `Hello ${name}`;
}
const getGreetingOnce = once(getGreeting);
getGreetingOnce('Alex');
// getGreeting called and "Hello Alex" is returned
// "Hello Alex" is put into the cache.
// returns "Hello Alex"
getGreetingOnce('Sam');
// getGreeting is not called
// "Hello Alex" is returned from the cache.
getGreetingOnce('Greg');
// getGreeting is not called
// "Hello Alex" is returned from the cache.
.clear()
)You can clear the cache of a onced function by using the .clear()
function property.
import { once } from 'limit-once';
function getGreeting(name: string): string {
return `Hello ${name}`;
}
const getGreetingOnce = once(getGreeting);
getGreetingOnce('Alex');
// getGreeting called and "Hello Alex" is returned.
// "Hello Alex" is put into the cache
// getGreetingOnce function returns "Hello Alex"
getGreetingOnce('Sam');
// getGreeting is not called
// "Hello Alex" is returned from cache
getGreetingOnce.clear();
getGreetingOnce('Greg');
// getGreeting is called and "Hello Greg" is returned.
// "Hello Greg" is put into the cache
// "Hello Greg" is returned.
Our async variant allows you to have a once
functionality for functions that Promise
.
import { onceAsync } from 'limit-once';
async function getLoggedInUser() {
await fetch('/user').json();
}
// We don't want every call to `getLoggedInUser()` to call `fetch` again.
// Ideally we would store the result of the first successful call and return that!
const getLoggedInUserOnce = onceAsync(getLoggedInUser);
const user1 = await getLoggedInUserOnce();
// subsequent calls won't call fetch, and will return the previously fulfilled promise value.
const user2 = await getLoggedInUserOnce();
A "rejected" promise call will not be cached and will allow the wrapped function to be called again
import { onceAsync } from 'limit-once';
let callCount = 0;
async function maybeThrow({ shouldThrow }: { shouldThrow: boolean }): Promise<string> {
callCount++;
if (shouldThrow) {
throw new Error(`Call count: ${callCount}`);
}
return `Call count: ${callCount}`;
}
const maybeThrowOnce = onceAsync(maybeThrow);
expect(async () => await maybeThrowOnce({ shouldThrow: true })).toThrowError('Call count: 1');
// failure result was not cached, underlying `maybeThrow` called again
expect(async () => await maybeThrowOnce({ shouldThrow: true })).toThrowError('Call count: 2');
// our first successful result will be cached
expect(await maybeThrowOnce({ shouldThrow: false })).toBe('Call count: 3');
expect(await maybeThrowOnce({ shouldThrow: false })).toBe('Call count: 3');
If multiple calls are made to the onced function while the original promise is still "pending"
, then the original promise is re-used. This prevents multiple calls to the underlying function.
import { onceAsync } from 'limit-once';
async function getLoggedInUser() {
await fetch('/user').json();
}
export const getLoggedInUserOnce = onceAsync(getLoggedInUser);
const promise1 = getLoggedInUserOnce();
// This second call to `getLoggedInUserOnce` while the `getLoggedInUser` promise
// is still "pending" will return the same promise that the first call created.
const promise2 = getLoggedInUserOnce();
console.log(promise1 === promise2); // "true"
.clear()
)You can clear the cache of a onced async function by using the .clear()
function property.
import { onceAsync } from 'limit-once';
let callCount = 0;
async function getCallCount(): Promise<string> {
return `Call count: ${callCount}`;
}
const onced = onceAsync(getCallCount);
expect(await onced({ shouldThrow: false })).toBe('Call count: 1');
expect(await onced({ shouldThrow: false })).toBe('Call count: 1');
onced.clear();
expect(await onced({ shouldThrow: false })).toBe('Call count: 2');
expect(await onced({ shouldThrow: false })).toBe('Call count: 2');
If onced async function is "pending"
when .clear()
is called, then the promise will be rejected.
import { onceAsync } from 'limit-once';
async function getName(): Promise<string> {
return 'Alex';
}
const getNameOnce = onceAsync(getName);
const promise1 = getNameOnce().catch(() => {
console.log('rejected');
});
// cached cleared while promise was pending
// will cause `promise1` to be rejected
getNameOnce.clear();
FAQs
Remember the first result of a function call
The npm package limit-once receives a total of 1 weekly downloads. As such, limit-once popularity was classified as not popular.
We found that limit-once demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.