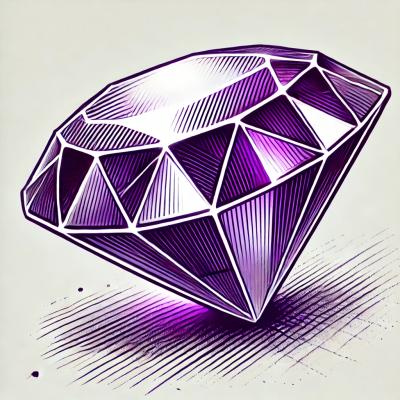
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Requires LLVM 3.2:
Ubuntu: sudo apt-get install libllvm3.2 llvm-3.2-dev
Fedora: sudo yum install llvm llvm-devel llvm-static
examples/
. Expressions must be entered on a single line.For details, see the LLVM C++ API documentation and Reference Manual.
llvm = require('llvm')
.globalContext
.voidTy
.labelTy
.floatTy
.doubleTy
.metadataTy
.x86_FP80Ty
.fP128Ty
.pPC_FP128Ty
.x86_MMXTy
.int1Ty
.int8Ty
.int16Ty
.int32Ty
.int64Ty
.getFunctionType(returnType, argTypes)
.getIntNTy(width)
new llvm.module(id, context)
.context
.moduleIdentifier
.getFunction(name)
.getOrInsertFunction(name, funcType)
.dump()
.parent
.name [r/w]
.dump()
Inherits Value
.module
.context
.functionType
.arguments -- modifications to the array are not reflected in the LLVM structure
.basicBlocks -- modifications to the array are not reflected in the LLVM structure
.dump()
.addBasicBlock(nameOrBlock) - adds an existing block, or creates one if passed a string name. Returns the added block.
Inherits Value
new llvm.BasicBlock(context)
.context
.getPointerTo() -- PointerType to this type
Inherits Type
.const(val, [radix]) -- Get ConstantInteger from JS number or string and radix
Inherits Type
.const(val) -- Get ConstantFP from JS number or string
Inherits Type
new llvm.IRBuilder(context)
.setInsertPoint(block)
.insertBlock -- the current insertion point block
The following methods create an instruction and add it at the current insertion point, returning the instruction's value:
.createRet(val)
.createRetVoid()
.createBr(destBlock)
.createCondBr(cond, destT, destF)
.createSwitch(V, defaultBlock) -- creates SwitchInst with .addCase(value, block)
and .setDefaultDest(block)
.createUnreachable()
.createCall(func, args)
.createAlloca(type, [arraySize], [name])
.createLoad(ptr, [name])
.createStore(val, ptr, [name])
.createGEP(ptr, idxList, [name])
.createPHI(type, [name]) -- creates PHINode with .addIncoming(block, value)
.createSelect(cond, vTrue, vFalse)
.createAdd(l, r, [name], [hasNUW], [hasNSW])
.createSub(l, r, [name], [hasNUW], [hasNSW])
.createMul(l, r, [name], [hasNUW], [hasNSW])
.createShl(l, r, [name], [hasNUW], [hasNSW])
.createUDiv(l, r, [name], [isExact])
.createSDiv(l, r, [name], [isExact])
.createLShr(l, r, [name], [isExact])
.createAShr(l, r, [name], [isExact])
.createNSWAdd(l, r, [name])
.createNUWAdd(l, r, [name])
.createFAdd(l, r, [name])
.createNSWSub(l, r, [name])
.createNUWSub(l, r, [name])
.createFSub(l, r, [name])
.createNSWMul(l, r, [name])
.createNUWMul(l, r, [name])
.createFMul(l, r, [name])
.createExactUDiv(l, r, [name])
.createExactSDiv(l, r, [name])
.createFDiv(l, r, [name])
.createURem(l, r, [name])
.createSRem(l, r, [name])
.createFRem(l, r, [name])
.createAnd(l, r, [name])
.createOr(l, r, [name])
.createXor(l, r, [name])
.createICmpEQ(l, r, [name])
.createICmpNE(l, r, [name])
.createICmpUGT(l, r, [name])
.createICmpUGE(l, r, [name])
.createICmpULT(l, r, [name])
.createICmpULE(l, r, [name])
.createICmpSGT(l, r, [name])
.createICmpSGE(l, r, [name])
.createICmpSLT(l, r, [name])
.createICmpSLE(l, r, [name])
.createFCmpOEQ(l, r, [name])
.createFCmpOGT(l, r, [name])
.createFCmpOGE(l, r, [name])
.createFCmpOLT(l, r, [name])
.createFCmpOLE(l, r, [name])
.createFCmpONE(l, r, [name])
.createFCmpORD(l, r, [name])
.createFCmpUNO(l, r, [name])
.createFCmpUEQ(l, r, [name])
.createFCmpUGT(l, r, [name])
.createFCmpUGE(l, r, [name])
.createFCmpULT(l, r, [name])
.createFCmpULE(l, r, [name])
.createFCmpUNE(l, r, [name])
.createNSWNeg(v, [name])
.createNUWNeg(v, [name])
.createFNeg(v, [name])
.createNot(v, [name])
.createIsNull(v, [name])
.createIsNotNull(v, [name])
.createTrunc(v, type, [name])
.createZExt(v, type, [name])
.createSExt(v, type, [name])
.createFPToUI(v, type, [name])
.createFPToSI(v, type, [name])
.createUIToFP(v, type, [name])
.createSIToFP(v, type, [name])
.createFPTrunc(v, type, [name])
.createFPExt(v, type, [name])
.createPtrToInt(v, type, [name])
.createIntToPtr(v, type, [name])
.createBitCast(v, type, [name])
.createZExtOrBitCast(v, type, [name])
.createSExtOrBitCast(v, type, [name])
.createTruncOrBitCast(v, type, [name])
.createPointerCast(v, type, [name])
.createFPCast(v, type, [name])
Inherits Value
.addIncoming(value, block)
.addCase(value, block)
.setDefaultDest(block)
new llvm.FunctionPassManager(module)
.doInitialization()
.doFinalization()
.run(function)
The following methods create and add an llvm::Pass
to the PassManager:
.addDataLayoutPass(dataLayout)
.addBasicAliasAnalysisPass()
.addInstructionCombiningPass()
.addReassociatePass()
.addGVNPass()
new llvm.ExecutionEngine(module)
.getPointerToFunction(fnName) -- Get 0-byte Buffer at the JIT'd function's address.
.getFFIFunction(fnName) -- Wrap the compiled function with the FFI library, returning a JavaScript callable.
FAQs
Bindings for the LLVM compiler infrastructure
We found that llvm demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.