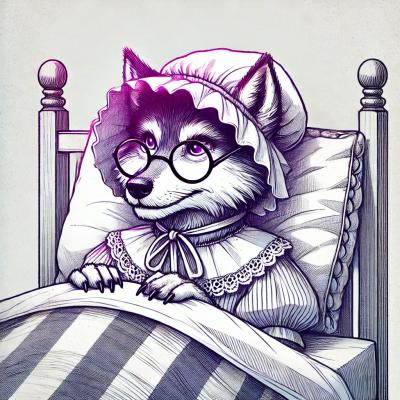
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
A very polite lock file utility, which endeavors to not litter, and to wait patiently for others.
The lockfile npm package is used to create and manage lock files, which are useful for ensuring that only one instance of a process is running at a time. This can be particularly useful in scenarios where you want to prevent race conditions or ensure that a resource is not accessed concurrently by multiple processes.
Creating a Lockfile
This feature allows you to create a lockfile to ensure that only one instance of a process is running. The code sample demonstrates how to acquire a lock with specific options and release it after the work is done.
const lockFile = require('lockfile');
const options = {
wait: 10000, // Wait for 10 seconds
pollPeriod: 100, // Check every 100ms
stale: 30000, // Consider the lock stale after 30 seconds
retries: 3, // Retry 3 times
retryWait: 100 // Wait 100ms between retries
};
lockFile.lock('path/to/file.lock', options, function (err) {
if (err) {
console.error('Failed to acquire lock:', err);
} else {
console.log('Lock acquired');
// Do some work
lockFile.unlock('path/to/file.lock', function (err) {
if (err) {
console.error('Failed to release lock:', err);
} else {
console.log('Lock released');
}
});
}
});
Checking Lockfile Status
This feature allows you to check if a lockfile is currently active. The code sample demonstrates how to check the status of a lockfile and handle the result.
const lockFile = require('lockfile');
lockFile.check('path/to/file.lock', function (err, isLocked) {
if (err) {
console.error('Error checking lock status:', err);
} else if (isLocked) {
console.log('File is locked');
} else {
console.log('File is not locked');
}
});
Updating Lockfile Options
This feature allows you to update the options for a lockfile, such as the stale time. The code sample demonstrates how to acquire a lock with updated options and release it after the work is done.
const lockFile = require('lockfile');
const options = {
stale: 60000 // Consider the lock stale after 60 seconds
};
lockFile.lock('path/to/file.lock', options, function (err) {
if (err) {
console.error('Failed to acquire lock:', err);
} else {
console.log('Lock acquired with updated options');
// Do some work
lockFile.unlock('path/to/file.lock', function (err) {
if (err) {
console.error('Failed to release lock:', err);
} else {
console.log('Lock released');
}
});
}
});
The proper-lockfile package provides similar functionality to lockfile, allowing you to create and manage lock files. It offers additional features such as automatic lock renewal and customizable lockfile paths. Compared to lockfile, proper-lockfile provides a more modern API and additional configuration options.
The async-lock package is another alternative that provides locking mechanisms for asynchronous code. It allows you to create locks for specific keys and ensures that only one function can execute for a given key at a time. While it does not create physical lock files, it provides a similar functionality for managing concurrency in asynchronous code.
The redlock package is a distributed lock manager for Redis. It allows you to create and manage locks across multiple Redis instances, providing high availability and fault tolerance. Compared to lockfile, redlock is designed for distributed systems and offers more robust locking mechanisms for distributed environments.
A very polite lock file utility, which endeavors to not litter, and to wait patiently for others.
var lockFile = require('lockfile')
// opts is optional, and defaults to {}
lockFile.lock('some-file.lock', opts, function (er) {
// if the er happens, then it failed to acquire a lock.
// if there was not an error, then the file was created,
// and won't be deleted until we unlock it.
// do my stuff, free of interruptions
// then, some time later, do:
lockFile.unlock('some-file.lock', function (er) {
// er means that an error happened, and is probably bad.
})
})
Sync methods return the value/throw the error, others don't. Standard node fs stuff.
All known locks are removed when the process exits. Of course, it's possible for certain types of failures to cause this to fail, but a best effort is made to not be a litterbug.
Acquire a file lock on the specified path
Acquire a file lock on the specified path
Close and unlink the lockfile.
Close and unlink the lockfile.
Check if the lockfile is locked and not stale.
Returns boolean.
Check if the lockfile is locked and not stale.
Callback is called with cb(error, isLocked)
.
A number of milliseconds to wait for locks to expire before giving up. Only used by lockFile.lock. Relies on fs.watch. If the lock is not cleared by the time the wait expires, then it returns with the original error.
A number of milliseconds before locks are considered to have expired.
Used by lock and lockSync. Retry n
number of times before giving up.
Used by lock. Wait n
milliseconds before retrying.
FAQs
A very polite lock file utility, which endeavors to not litter, and to wait patiently for others.
We found that lockfile demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.