loco-js-model
Advanced tools
Comparing version 1.1.1 to 2.0.0
@@ -1,10 +0,16 @@ | ||
export default (responseJSON) => { | ||
const createMockXHR = (responseJSON) => { | ||
return { | ||
open: jest.fn(), | ||
send: jest.fn(), | ||
responseText: JSON.stringify( | ||
responseJSON || {} | ||
), | ||
setRequestHeader: jest.fn() | ||
open: jest.fn(), | ||
send: jest.fn(), | ||
responseText: JSON.stringify( | ||
responseJSON || {} | ||
), | ||
setRequestHeader: jest.fn() | ||
}; | ||
} | ||
export default () => { | ||
const mock = createMockXHR(); | ||
window.XMLHttpRequest = jest.fn(() => mock); | ||
return mock; | ||
}; |
@@ -5,6 +5,14 @@ import { Config } from "index"; | ||
it("provides getter and setter", () => { | ||
expect(Config.cookiesByCORS).toEqual(false); | ||
expect(Config.cookiesByCORS).toEqual(undefined); | ||
Config.cookiesByCORS = true; | ||
expect(Config.cookiesByCORS).toEqual(true); | ||
}); | ||
}); | ||
}); | ||
describe("authorizationHeader", () => { | ||
it("provides getter and setter", () => { | ||
expect(Config.authorizationHeader).toEqual(undefined); | ||
Config.authorizationHeader = "Bearer XXX"; | ||
expect(Config.authorizationHeader).toEqual("Bearer XXX"); | ||
}); | ||
}); |
import nock from 'nock'; | ||
import createMockXHR from "../../__mock__/xhr"; | ||
import mockXHR from "../../__mock__/xhr"; | ||
import { Config, Models } from "index"; | ||
class Comment extends Models.Base { | ||
static authorizationHeader = "Bearer XXX"; | ||
static identity = "Article.Comment"; | ||
@@ -64,2 +66,4 @@ static remoteName = "Comment"; | ||
class Dummy extends Models.Base { | ||
static protocolWithHost = "https://myapp.test"; | ||
static identity = "Dummy"; | ||
@@ -82,8 +86,2 @@ | ||
const mockXHR = () => { | ||
const mock = createMockXHR(); | ||
window.XMLHttpRequest = jest.fn(() => mock); | ||
return mock; | ||
}; | ||
afterEach(() => { | ||
@@ -93,3 +91,3 @@ window.XMLHttpRequest = oldXMLHttpRequest; | ||
it("does not send param if was used in URL", () => { | ||
it("does not send param if was used in URL + .all uses Authorization header if defined", () => { | ||
const mock = mockXHR(); | ||
@@ -101,5 +99,10 @@ Comment.all({ articleId: 1 }); | ||
); | ||
expect(mock.setRequestHeader).toBeCalledWith("Authorization", "Bearer XXX"); | ||
}); | ||
describe("requests", () => { | ||
afterEach(() => { | ||
Config.authorizationHeader = null; | ||
}); | ||
it("does not set withCredentials by default", () => { | ||
@@ -119,2 +122,9 @@ const mock = mockXHR(); | ||
}); | ||
it("is possible to change Authorization header via Config", () => { | ||
const mock = mockXHR(); | ||
Config.authorizationHeader = "Bearer YYY"; | ||
Comment.find({id: 25, articleId: 4}); | ||
expect(mock.setRequestHeader).toBeCalledWith("Authorization", "Bearer YYY"); | ||
}); | ||
}); | ||
@@ -173,6 +183,7 @@ | ||
it("uses a correct URL", () => { | ||
it("uses a correct URL and sets Authorization if defined", () => { | ||
const mock = mockXHR(); | ||
Comment.find({id: 25, articleId: 4}); | ||
expect(mock.open).toBeCalledWith("GET", "/user/articles/4/comments/25?"); | ||
expect(mock.setRequestHeader).toBeCalledWith("Authorization", "Bearer XXX"); | ||
}); | ||
@@ -188,4 +199,10 @@ | ||
describe(".__getResourcesUrl", () => { | ||
it("returns a correct URL", () => { | ||
expect(Dummy.__getResourcesUrl()).toEqual("https://myapp.test/dummys"); | ||
}); | ||
}); | ||
describe("#save", () => { | ||
it("properly builds URL for nested models", () => { | ||
it("properly builds URL for nested models and sets Authorization header if defined", () => { | ||
const mock = mockXHR(); | ||
@@ -199,2 +216,3 @@ const comment = new Comment({ | ||
expect(mock.open).toBeCalledWith("POST", "/user/articles/1/comments"); | ||
expect(mock.setRequestHeader).toBeCalledWith("Authorization", "Bearer XXX"); | ||
}); | ||
@@ -201,0 +219,0 @@ }); |
@@ -1,1 +0,1 @@ | ||
!function(t,r){"object"==typeof exports&&"object"==typeof module?module.exports=r():"function"==typeof define&&define.amd?define([],r):"object"==typeof exports?exports.LocoModel=r():t.LocoModel=r()}(window,(function(){return function(t){var r={};function e(o){if(r[o])return r[o].exports;var n=r[o]={i:o,l:!1,exports:{}};return t[o].call(n.exports,n,n.exports,e),n.l=!0,n.exports}return e.m=t,e.c=r,e.d=function(t,r,o){e.o(t,r)||Object.defineProperty(t,r,{enumerable:!0,get:o})},e.r=function(t){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(t,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(t,"__esModule",{value:!0})},e.t=function(t,r){if(1&r&&(t=e(t)),8&r)return t;if(4&r&&"object"==typeof t&&t&&t.__esModule)return t;var o=Object.create(null);if(e.r(o),Object.defineProperty(o,"default",{enumerable:!0,value:t}),2&r&&"string"!=typeof t)for(var n in t)e.d(o,n,function(r){return t[r]}.bind(null,n));return o},e.n=function(t){var r=t&&t.__esModule?function(){return t.default}:function(){return t};return e.d(r,"a",r),r},e.o=function(t,r){return Object.prototype.hasOwnProperty.call(t,r)},e.p="",e(e.s=0)}([function(t,r,e){"use strict";function o(t,r){for(var e=0;e<r.length;e++){var o=r[e];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(t,o.key,o)}}e.r(r),e.d(r,"Config",(function(){return n})),e.d(r,"I18n",(function(){return s})),e.d(r,"IdentityMap",(function(){return p})),e.d(r,"Models",(function(){return C})),e.d(r,"Validators",(function(){return k}));var n=new(function(){function t(){!function(t,r){if(!(t instanceof r))throw new TypeError("Cannot call a class as a function")}(this,t),this.localeVar="en",this.protocolWithHostVar=null,this.scopeVar=null,this.cookiesByCORSVar=!1}var r,e,n;return r=t,(e=[{key:"cookiesByCORS",get:function(){return this.cookiesByCORSVar},set:function(t){return this.cookiesByCORSVar=t,this.cookiesByCORSVar}},{key:"locale",get:function(){return this.localeVar},set:function(t){return this.localeVar=t,this.localeVar}},{key:"protocolWithHost",get:function(){return this.protocolWithHostVar},set:function(t){return t?"/"===t[t.length-1]?(this.protocolWithHostVar=t.slice(0,t.length-1),this.protocolWithHostVar):(this.protocolWithHostVar=t,this.protocolWithHostVar):(this.protocolWithHostVar=null,this.protocolWithHostVar)}},{key:"scope",get:function(){return this.scopeVar},set:function(t){return this.scopeVar=t,this.scopeVar}}])&&o(r.prototype,e),n&&o(r,n),t}()),s={en:{variants:{},models:{},attributes:{},errors:{messages:{accepted:"must be accepted",blank:"can't be blank",confirmation:"doesn't match %{attribute}",empty:"can't be empty",equal_to:"must be equal to %{count}",even:"must be even",exclusion:"is reserved",greater_than:"must be greater than %{count}",greater_than_or_equal_to:"must be greater than or equal to %{count}",inclusion:"is not included in the list",invalid:"is invalid",less_than:"must be less than %{count}",less_than_or_equal_to:"must be less than or equal to %{count}",not_a_number:"is not a number",not_an_integer:"must be an integer",odd:"must be odd",present:"must be blank",too_long:{one:"is too long (maximum is 1 character)",other:"is too long (maximum is %{count} characters)"},too_short:{one:"is too short (minimum is 1 character)",other:"is too short (minimum is %{count} characters)"},wrong_length:{one:"is the wrong length (should be 1 character)",other:"is the wrong length (should be %{count} characters)"},other_than:"must be other than %{count}"}}}};function i(t){return(i="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t})(t)}var a,u={},l=function(t,r){if(-1!==t.indexOf(r))return null;var e=function(t){var r=t.length;return t.find((function(t,e){if(null===t)return r=e,!0})),r}(t);return t[e]=r,e},c=function(t,r,e){return u[t][r][e]=null},h=function(t){var r=t.getIdentity();void 0===u[r]&&(u[r]={}),void 0===u[r][t.id]&&(u[r][t.id]=[]),u[r][t.id][0]=t},p={get imap(){return u},clear:function(){return u={}},subscribe:function(t){var r=function(){};if("object"===i(t.to)){var e=function(t){var r=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{},e=r.with;h(e);var o=u[e.getIdentity()][e.id];return l(o,t)}(t.with,{with:t.to});return null===e?r:function(){c(t.to.getIdentity(),t.to.id,e)}}if("function"==typeof t.to){var o=function(t){var r=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{};void 0===u[t]&&(u[t]={}),void 0===u[t].collection&&(u[t].collection=[]);var e=u[t].collection;return l(e,r.to)}(t.to.getIdentity(),{to:t.with});return null===o?r:function(){c(t.to.getIdentity(),"collection",o)}}},unsubscribe:c,add:h,find:function(t,r){return void 0!==u[t]&&null!=u[t][r]?u[t][r][0]:null},findConnected:function(t,r){return void 0!==u[t]&&void 0!==u[t][r]&&u[t][r].length>1?u[t][r].slice(1):[]}},f=function(){function t(){this.obj=null,this.attr=null,this.val=null,this.opts=null}return t.sharedInstances={},t.instance=function(t,r,e){var o,n;return n=this.identity,null==this.sharedInstances[n]&&(this.sharedInstances[n]=new k[n]),(o=this.sharedInstances[n]).assignAttribs(t,r,e),o},t.prototype.assignAttribs=function(t,r,e){return this.obj=t,this.attr=r,this.val=this.obj[this.attr],this.opts=e},t}(),d={}.hasOwnProperty,_=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)d.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Absence",r.prototype.validate=function(){switch(typeof this.val){case"string":if(null!=this.val&&0===this.val.length)return;break;default:if(null==this.val)return}return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.present,this.obj.addErrorMessage(t,{for:this.attr})},r}(f),g={}.hasOwnProperty,y=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)g.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Confirmation",r.prototype.validate=function(){var t;if(t=this.obj[this._properAttr()],null==this.val||null==t||this.val!==t)return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t,r,e,o;return e=this.attr.charAt(0).toUpperCase()+this.attr.slice(1),t=(r=s[n.locale].attributes[this.obj.getIdentity()])&&r[this.attr]||e,o=(o=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.confirmation).replace("%{attribute}",t),this.obj.addErrorMessage(o,{for:this._properAttr()})},r.prototype._properAttr=function(){return this.attr+"Confirmation"},r}(f),m={}.hasOwnProperty,v=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)m.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Exclusion",r.prototype.validate=function(){if(-1!==(this.opts.in||this.opts.within||[]).indexOf(this.val))return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.exclusion,this.obj.addErrorMessage(t,{for:this.attr})},r}(f),b={}.hasOwnProperty,E=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)b.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Format",r.prototype.validate=function(){if(null==this.opts.with.exec(this.val))return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.invalid,this.obj.addErrorMessage(t,{for:this.attr})},r}(f),w={}.hasOwnProperty,M=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)w.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Inclusion",r.prototype.validate=function(){if(-1===(this.opts.in||this.opts.within||[]).indexOf(this.val))return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.inclusion,this.obj.addErrorMessage(t,{for:this.attr})},r}(f),O={}.hasOwnProperty,P=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)O.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Length",r.prototype.validate=function(){var t;if(null!=this.val&&null!==(t=null!=this._range()[0]&&null!=this._range()[1]&&this._range()[0]===this._range()[1]&&this.val.length!==this._range()[0]?this._selectErrorMessage("wrong_length",this._range()[0]):null!=this._range()[0]&&this.val.length<this._range()[0]?this._selectErrorMessage("too_short",this._range()[0]):null!=this._range()[1]&&this.val.length>this._range()[1]?this._selectErrorMessage("too_long",this._range()[1]):null))return this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._range=function(){return[this.opts.minimum||this.opts.is||null!=this.opts.within&&this.opts.within[0]||null,this.opts.maximum||this.opts.is||null!=this.opts.within&&this.opts.within[1]||null]},r.prototype._selectErrorMessage=function(t,r){var e,o,i,a,u;if(1===r)return s[n.locale].errors.messages[t].one;for(i=null,e=0,o=(a=["few","many"]).length;e<o;e++)if(u=a[e],this._checkVariant(u,r)){i=s[n.locale].errors.messages[t][u];break}return null==i&&(i=s[n.locale].errors.messages[t].other),null!=this.opts.message&&(i=this.opts.message),/%{count}/.exec(i)&&(i=i.replace("%{count}",r)),i},r.prototype._checkVariant=function(t,r){if(null!=s[n.locale].variants[t])return s[n.locale].variants[t](r)},r}(f),j={}.hasOwnProperty,N=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)j.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Numericality",r.prototype.validate=function(){return isNaN(this.val)?this._addNaNErrorMessage():null!=this.opts.only_integer&&Number(this.val)!==parseInt(this.val)?this._addIntErrorMessage():null!=this.opts.greater_than&&Number(this.val)<=this.opts.greater_than?this._addGreatherThanErrorMessage():null!=this.opts.greater_than_or_equal_to&&Number(this.val)<this.opts.greater_than_or_equal_to?this._addGreatherThanOrEqualToErrorMessage():null!=this.opts.equal_to&&Number(this.val)!==this.opts.equal_to?this._addEqualToErrorMessage():null!=this.opts.less_than&&Number(this.val)>=this.opts.less_than?this._addLessThanErrorMessage():null!=this.opts.less_than_or_equal_to&&Number(this.val)>this.opts.less_than_or_equal_to?this._addLessThanOrEqualToErrorMessage():null!=this.opts.other_than&&Number(this.val)===this.opts.other_than?this._addOtherThanErrorMessage():null!=this.opts.odd&&Number(this.val)%2!=1?this._addOddErrorMessage():null!=this.opts.even&&Number(this.val)%2!=0?this._addEvenErrorMessage():void 0},r.prototype._addNaNErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.not_a_number,this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addIntErrorMessage=function(){var t;return t=s[n.locale].errors.messages.not_an_integer,this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addGreatherThanErrorMessage=function(){var t;return t=(t=s[n.locale].errors.messages.greater_than).replace("%{count}",this.opts.greater_than),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addGreatherThanOrEqualToErrorMessage=function(){var t;return t=(t=s[n.locale].errors.messages.greater_than_or_equal_to).replace("%{count}",this.opts.greater_than_or_equal_to),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addEqualToErrorMessage=function(){var t;return t=(t=s[n.locale].errors.messages.equal_to).replace("%{count}",this.opts.equal_to),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addLessThanErrorMessage=function(){var t;return t=(t=s[n.locale].errors.messages.less_than).replace("%{count}",this.opts.less_than),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addLessThanOrEqualToErrorMessage=function(){var t;return t=(t=s[n.locale].errors.messages.less_than_or_equal_to).replace("%{count}",this.opts.less_than_or_equal_to),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addOtherThanErrorMessage=function(){var t;return t=(t=s[n.locale].errors.messages.other_than).replace("%{count}",this.opts.other_than),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addOddErrorMessage=function(){var t;return t=s[n.locale].errors.messages.odd,this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addEvenErrorMessage=function(){var t;return t=s[n.locale].errors.messages.even,this.obj.addErrorMessage(t,{for:this.attr})},r}(f),S={}.hasOwnProperty,T=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)S.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Presence",r.prototype.validate=function(){switch(typeof this.val){case"string":if(null!=this.val&&this.val.length>0)return;break;default:if(null!=this.val)return}return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[n.locale].errors.messages.blank,this.obj.addErrorMessage(t,{for:this.attr})},r}(f),R={}.hasOwnProperty;a=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)R.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Size",r.prototype.validate=function(){return P.instance(this.obj,this.attr,this.opts).validate()},r}(f);var k={Absence:_,Base:f,Confirmation:y,Exclusion:v,Format:E,Inclusion:M,Length:P,Numericality:N,Presence:T,Size:a},A=function(t){var r="";return Object.keys(t).forEach((function(e){""!==r&&(r="".concat(r,"&")),r="".concat(r).concat(e,"=").concat(encodeURIComponent(t[e]))})),r},I=function(t,r,e){var o=function(t){var r={};if(!t)return r;var e=["resource","total","count"];return Object.keys(t).forEach((function(o){-1===e.indexOf(o)&&(r[o]=t[o])})),r}(e),s="GET"===t?"".concat(r,"?").concat(A(o)):r,i=document.querySelector("meta[name='csrf-token']"),a=new XMLHttpRequest;return a.open(t,s),a.setRequestHeader("Accept","application/json"),a.setRequestHeader("Content-Type","application/json"),i&&a.setRequestHeader("X-CSRF-Token",i.content),a.withCredentials=n.cookiesByCORS,a.send(JSON.stringify(o)),a},q=function(){function t(t){null==t&&(t={}),this.id=null,this.errors=null,this.resource=t.resource,null!=this.constructor.attributes&&this.__initAttributes(),null!=t&&this.__assignAttributes(t)}return t.getIdentity=function(){if(null!=this.identity)return this.identity;throw"Specify Model's identity!"},t.getRemoteName=function(){return null!=this.remoteName?this.remoteName:this.getIdentity()},t.all=function(t){return null==t&&(t={}),this.get("all",t)},t.get=function(t,r){return null==r&&(r={}),this.__send("GET",t,r)},t.post=function(t,r){return null==r&&(r={}),this.__send("POST",t,r)},t.put=function(t,r){return null==r&&(r={}),this.__send("PUT",t,r)},t.patch=function(t,r){return null==r&&(r={}),this.__send("PATCH",t,r)},t.delete=function(t,r){return null==r&&(r={}),this.__send("DELETE",t,r)},t.find=function(t){var r,e,o,n,s;return n={},"object"==typeof t?(n=t,r=t.id,delete n.id):r=t,o=this.__getResourcesUrl(n)+"/"+r,e=I("GET",o,n),new Promise((s=this,function(r,o){return e.onerror=function(t){return o(t)},e.onload=function(e){var o;if(404!==e.target.status)return o=JSON.parse(e.target.response),r(s.__initFromJSON(o,t.resource));r(null)}}))},t.getAttribRemoteName=function(t){return null==this.attributes||null==this.attributes[t]?null:null==this.attributes[t].remoteName?t:this.attributes[t].remoteName},t.getResourcesUrlParams=function(t){var r,e,o,n;for(n=this.__getResourcesUrl({resource:t.resource}),o=/:(\w+)\/?/,e=[];r=o.exec(n);)e.push(r[1]),n=n.replace(r[0],r[1]);return e},t.__getResourcesUrl=function(t){var r,e;return e=null==this.resources?"/"+this.getRemoteName().toLowerCase()+"s":t.resource?this.resources[t.resource].url:null!=n.scope&&null!=this.resources[n.scope]?this.resources[n.scope].url:this.resources.url,null!=n.protocolWithHost&&(e=""+n.protocolWithHost+e),null==(r=/:([a-zA-Z]+)\/?/.exec(e))||(null!=t[r[1]]?(e=e.replace(":"+r[1],t[r[1]]),delete t[r[1]]):null!=t.obj&&null!=t.obj[r[1]]&&(e=e.replace(":"+r[1],t.obj[r[1]]))),e},t.__page=function(t,r,e){var o,n,s;return n=r.url,r.params[r.pageParam]=t,o=I(r.method,n,r.params),new Promise((s=this,function(t,n){return o.onerror=function(t){return n(t)},o.onload=function(o){var n,i,a,u,l,c,h,p,f,d;if((n=JSON.parse(o.target.response)).constructor===Array)for(i=0,l=n.length;i<l;i++)p=n[i],h=s.__initFromJSON(p,r.resource),e.push(h);else if(null!=n.resources){for(e.constructor===Array&&(e={resources:[],count:0}),a=0,c=(f=n.resources).length;a<c;a++)p=f[a],h=s.__initFromJSON(p,r.resource),e.resources.push(h);e.count=n.count}else for(u in n)d=n[u],e[u]=d;return t(e)}}))},t.__paginate=function(t){var r,e;return r={method:t.method,url:t.url,params:t.params,pageParam:t.pageParam,resource:t.resource},this.__page(t.pageNum||1,r,[]).then((e=this,function(o){var n,s,i,a,u,l;if(l=o.count||t.total,a=Promise.resolve(o),null!=t.pageNum)return a;if(l<=t.perPage)return a;if((i=parseInt(l/t.perPage))!==l/t.perPage&&(i+=1),1===i)return a;for(n=s=2,u=i;2<=u?s<=u:s>=u;n=2<=u?++s:--s)!function(t){a=a.then((function(n){return e.__page(t,r,o)}))}(n);return a}))},t.__getPaginationParam=function(t){var r,e,o,s,i;return"page",null!=t&&null!=this.resources&&this.resources[t]?(null!=(r=this.resources[t].paginate)?r.param:void 0)||"page":null!=n.scope&&null!=this.resources&&null!=this.resources[n.scope]?(null!=(e=this.resources[n.scope])&&null!=(o=e.paginate)?o.param:void 0)||"page":null!=(null!=(s=this.resources)&&null!=(i=s.paginate)?i.param:void 0)?this.resources.paginate.param:"page"},t.__getPaginationPer=function(t){var r,e,o,s,i;return null!=t&&null!=this.resources&&this.resources[t]?null!=(r=this.resources[t].paginate)?r.per:void 0:null!=n.scope&&null!=this.resources&&null!=this.resources[n.scope]?null!=(e=this.resources[n.scope])&&null!=(o=e.paginate)?o.per:void 0:null!=(null!=(s=this.resources)&&null!=(i=s.paginate)?i.per:void 0)?this.resources.paginate.per:null},t.__send=function(t,r,e){var o,n;return n=this.__getResourcesUrl(e),"all"!==r&&(n=n+"/"+r),o={method:t,url:n,params:e,resource:e.resource,perPage:this.__getPaginationPer(e.resource),pageNum:e.page,pageParam:this.__getPaginationParam(e.resource),total:e.total||e.count},this.__paginate(o)},t.__initFromJSON=function(t,r){var e;return(e=new this(t)).resource=r,p.add(e),e},t.prototype.setResource=function(t){return this.resource=t},t.prototype.getIdentity=function(){return this.constructor.getIdentity()},t.prototype.getAttrRemoteName=function(t){return null==this.constructor.attributes||null==this.constructor.attributes[t]?null:this.constructor.attributes[t].remoteName||t},t.prototype.getAttrName=function(t){var r,e;if(null==this.constructor.attributes)return t;if(null!=this.constructor.attributes[t])return t;for(r in e=this.constructor.attributes)if(e[r].remoteName===t)return r;return t},t.prototype.getAttrType=function(t){return null==this.constructor.attributes||null==this.constructor.attributes[t]?null:this.constructor.attributes[t].type},t.prototype.assignAttr=function(t,r){var e;if(e=this.getAttrType(t),null!=r){switch(e){case"Date":r=new Date(Date.parse(r));break;case"Integer":case"Int":r=parseInt(r);break;case"Float":r=parseFloat(r);break;case"Boolean":case"Bool":r="boolean"==typeof r?r:Boolean(parseInt(r));break;case"Number":r=Number(r);break;case"String":r=String(r)}return this[t]=r}this[t]=null},t.prototype.attributes=function(){var t,r,e;if(t={id:this.id},null==this.constructor.attributes)return t;for(r in e=this.constructor.attributes)e[r],t[r]=this[r];return t},t.prototype.isValid=function(){var t,r,e,o,n,s,i,a,u,l,c;if(null==this.constructor.attributes)return!0;for(o in this.errors=null,s=this.constructor.attributes)if(null!=(t=s[o]).validations)for(u in i=t.validations)l=i[u],null!=this.id&&"create"===l.on||null==this.id&&"update"===l.on||(null==l.if||l.if(this))&&(c=u.charAt(0).toUpperCase()+u.slice(1),null!=k[c]?(n=this.__processedValidationSettings(l),k[c].instance(this,o,n).validate()):console.warn('"'+c+'" validator is not implemented!'));if(null!=this.constructor.validate)for(r=0,e=(a=this.constructor.validate).length;r<e;r++)this[a[r]]();return null==this.errors},t.prototype.isInvalid=function(){return!this.isValid()},t.prototype.isEmpty=function(){var t,r;for(t in r=this.attributes())if(r[t],null!==this[t])return!1;return!0},t.prototype.addErrorMessage=function(t,r){return null==r&&(r={}),null==this.errors&&(this.errors={}),null==this.errors[r.for]&&(this.errors[r.for]=[]),this.errors[r.for].push(t)},t.prototype.save=function(){var t,r,e;return t=null!=this.id?"PUT":"POST",r=I(t,this.__getResourceUrl(),this.serialize()),new Promise((e=this,function(t,o){return r.onerror=function(t){return o(t)},r.onload=function(r){var o;if(!(o=JSON.parse(r.target.response)).success)return null!=o.errors&&e.__assignRemoteErrorMessages(o.errors),t(o);t(o)}}))},t.prototype.updateAttribute=function(t){var r,e;return r=I("PUT",this.__getResourceUrl(),this.serialize(t)),new Promise((e=this,function(t,o){return r.onerror=function(t){return o(t)},r.onload=function(r){var n;return r.target.status>=200&&r.target.status<400?(n=JSON.parse(r.target.response)).success?void t(n):(null!=n.errors&&e.__assignRemoteErrorMessages(n.errors),t(n)):r.target.status>=500?o(r):void 0}}))},t.prototype.serialize=function(t){var r,e,o,n;if(null==t&&(t=null),null==this.constructor.attributes)return{};for(t in(e={})[o=this.constructor.getRemoteName().toLowerCase()]={},r={},null!=t?r[t]=null:r=this.constructor.attributes,r)r[t],n=this.getAttrRemoteName(t),e[o][n]=this[t];return e},t.prototype.reload=function(){var t,r,e,o,n;for(t={id:this.id,resource:this.resource},r=0,e=(n=this.constructor.getResourcesUrlParams({resource:this.resource})).length;r<e;r++)t[o=n[r]]=this[o];return this.constructor.find(t)},t.prototype.changes=function(){var t,r,e,o,n;for(r in o={},t=p.find(this.getIdentity(),this.id),e=this.attributes())if((n=e[r])!==t[r]){if(null!=n&&n.constructor===Date&&t[r]-n==0)continue;n!==t[r]&&(o[r]={is:t[r],was:n})}return o},t.prototype.applyChanges=function(){var t,r,e,o;for(t in e=[],r=this.changes())o=r[t],e.push(this[t]=o.is);return e},t.prototype.toKey=function(){return this.getIdentity().toLowerCase()+"_"+this.id},t.prototype.get=function(t,r){return null==r&&(r={}),this.__send("GET",t,r)},t.prototype.post=function(t,r){return null==r&&(r={}),this.__send("POST",t,r)},t.prototype.put=function(t,r){return null==r&&(r={}),this.__send("PUT",t,r)},t.prototype.patch=function(t,r){return null==r&&(r={}),this.__send("PATCH",t,r)},t.prototype.delete=function(t,r){return null==r&&(r={}),this.__send("DELETE",t,r)},t.prototype.__send=function(t,r,e){var o,n;return n=this.__getResourceUrl(),null!=r&&(n=n+"/"+r),o=I(t,n,e),new Promise((function(t,r){return o.onerror=function(t){return r(t)},o.onload=function(o){return o.target.status>=200&&o.target.status<400?(e=JSON.parse(o.target.response),t(e)):o.target.status>=500?r(o):void 0}}))},t.prototype.__assignAttributes=function(t){var r,e,o,n;for(e in o=[],t)n=t[e],r=this.getAttrName(e),o.push(this.assignAttr(r,n));return o},t.prototype.__initAttributes=function(){var t,r,e;for(t in e=[],r=this.constructor.attributes)r[t],e.push(this[t]=null);return e},t.prototype.__assignRemoteErrorMessages=function(t){var r,e,o,n,s;for(n in s=[],t)o=t[n],r=this.getAttrName(n),s.push(function(){var t,n,s;for(s=[],t=0,n=o.length;t<n;t++)e=o[t],s.push(this.addErrorMessage(e,{for:r}));return s}.call(this));return s},t.prototype.__getResourceUrl=function(){var t;return t=this.constructor.__getResourcesUrl({resource:this.resource,obj:this}),null==this.id?t:t+"/"+this.id},t.prototype.__processedValidationSettings=function(t){var r,e,o;for(r in o={},t)e=t[r],o[r]="function"==typeof e?e(this):e;return o},t}();function V(t,r){var e=Object.keys(t);if(Object.getOwnPropertySymbols){var o=Object.getOwnPropertySymbols(t);r&&(o=o.filter((function(r){return Object.getOwnPropertyDescriptor(t,r).enumerable}))),e.push.apply(e,o)}return e}function x(t,r,e){return r in t?Object.defineProperty(t,r,{value:e,enumerable:!0,configurable:!0,writable:!0}):t[r]=e,t}q.prototype.clone=function(){return new this.constructor(function(t){for(var r=1;r<arguments.length;r++){var e=null!=arguments[r]?arguments[r]:{};r%2?V(Object(e),!0).forEach((function(r){x(t,r,e[r])})):Object.getOwnPropertyDescriptors?Object.defineProperties(t,Object.getOwnPropertyDescriptors(e)):V(Object(e)).forEach((function(r){Object.defineProperty(t,r,Object.getOwnPropertyDescriptor(e,r))}))}return t}({},this.attributes()))};var C={Base:q}}])})); | ||
!function(t,r){"object"==typeof exports&&"object"==typeof module?module.exports=r():"function"==typeof define&&define.amd?define([],r):"object"==typeof exports?exports.LocoModel=r():t.LocoModel=r()}(self,(function(){return(()=>{"use strict";var t={d:(r,e)=>{for(var o in e)t.o(e,o)&&!t.o(r,o)&&Object.defineProperty(r,o,{enumerable:!0,get:e[o]})},o:(t,r)=>Object.prototype.hasOwnProperty.call(t,r),r:t=>{"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(t,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(t,"__esModule",{value:!0})}},r={};function e(t,r){for(var e=0;e<r.length;e++){var o=r[e];o.enumerable=o.enumerable||!1,o.configurable=!0,"value"in o&&(o.writable=!0),Object.defineProperty(t,o.key,o)}}t.r(r),t.d(r,{Config:()=>o,I18n:()=>s,IdentityMap:()=>c,Models:()=>U,Validators:()=>q});const o=new(function(){function t(){!function(t,r){if(!(t instanceof r))throw new TypeError("Cannot call a class as a function")}(this,t),this.localeVar="en",this.protocolWithHostVar=null,this.scopeVar=null}var r,o;return r=t,(o=[{key:"locale",get:function(){return this.localeVar},set:function(t){this.localeVar=t}},{key:"protocolWithHost",get:function(){return this.protocolWithHostVar},set:function(t){t?"/"===t[t.length-1]?this.protocolWithHostVar=t.slice(0,t.length-1):this.protocolWithHostVar=t:this.protocolWithHostVar=null}},{key:"scope",get:function(){return this.scopeVar},set:function(t){this.scopeVar=t}}])&&e(r.prototype,o),t}()),s={en:{variants:{},models:{},attributes:{},errors:{messages:{accepted:"must be accepted",blank:"can't be blank",confirmation:"doesn't match %{attribute}",empty:"can't be empty",equal_to:"must be equal to %{count}",even:"must be even",exclusion:"is reserved",greater_than:"must be greater than %{count}",greater_than_or_equal_to:"must be greater than or equal to %{count}",inclusion:"is not included in the list",invalid:"is invalid",less_than:"must be less than %{count}",less_than_or_equal_to:"must be less than or equal to %{count}",not_a_number:"is not a number",not_an_integer:"must be an integer",odd:"must be odd",present:"must be blank",too_long:{one:"is too long (maximum is 1 character)",other:"is too long (maximum is %{count} characters)"},too_short:{one:"is too short (minimum is 1 character)",other:"is too short (minimum is %{count} characters)"},wrong_length:{one:"is the wrong length (should be 1 character)",other:"is the wrong length (should be %{count} characters)"},other_than:"must be other than %{count}"}}}};function n(t){return n="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t},n(t)}var i={},a=function(t,r){if(-1!==t.indexOf(r))return null;var e=function(t){var r=t.length;return t.find((function(t,e){if(null===t)return r=e,!0})),r}(t);return t[e]=r,e},u=function(t,r,e){return i[t][r][e]=null},l=function(t){var r=t.getIdentity();void 0===i[r]&&(i[r]={}),void 0===i[r][t.id]&&(i[r][t.id]=[]),i[r][t.id][0]=t};const c={get imap(){return i},clear:function(){return i={}},subscribe:function(t){var r=function(){};if("object"===n(t.to)){var e=function(t){var r=(arguments.length>1&&void 0!==arguments[1]?arguments[1]:{}).with;l(r);var e=i[r.getIdentity()][r.id];return a(e,t)}(t.with,{with:t.to});return null===e?r:function(){u(t.to.getIdentity(),t.to.id,e)}}if("function"==typeof t.to){var o=function(t){var r=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{};void 0===i[t]&&(i[t]={}),void 0===i[t].collection&&(i[t].collection=[]);var e=i[t].collection;return a(e,r.to)}(t.to.getIdentity(),{to:t.with});return null===o?r:function(){u(t.to.getIdentity(),"collection",o)}}},unsubscribe:u,add:l,find:function(t,r){return void 0!==i[t]&&null!=i[t][r]?i[t][r][0]:null},findConnected:function(t,r){return void 0!==i[t]&&void 0!==i[t][r]&&i[t][r].length>1?i[t][r].slice(1):[]}};const h=function(){function t(){this.obj=null,this.attr=null,this.val=null,this.opts=null}return t.sharedInstances={},t.instance=function(t,r,e){var o,s;return s=this.identity,null==this.sharedInstances[s]&&(this.sharedInstances[s]=new q[s]),(o=this.sharedInstances[s]).assignAttribs(t,r,e),o},t.prototype.assignAttribs=function(t,r,e){return this.obj=t,this.attr=r,this.val=this.obj[this.attr],this.opts=e},t}();var p={}.hasOwnProperty;const f=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)p.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Absence",r.prototype.validate=function(){if("string"==typeof this.val){if(null!=this.val&&0===this.val.length)return}else if(null==this.val)return;return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.present,this.obj.addErrorMessage(t,{for:this.attr})},r}(h);var _={}.hasOwnProperty;const d=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)_.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Confirmation",r.prototype.validate=function(){var t;if(t=this.obj[this._properAttr()],null==this.val||null==t||this.val!==t)return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t,r,e,n;return e=this.attr.charAt(0).toUpperCase()+this.attr.slice(1),t=(r=s[o.locale].attributes[this.obj.getIdentity()])&&r[this.attr]||e,n=(n=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.confirmation).replace("%{attribute}",t),this.obj.addErrorMessage(n,{for:this._properAttr()})},r.prototype._properAttr=function(){return this.attr+"Confirmation"},r}(h);var g={}.hasOwnProperty;const y=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)g.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Exclusion",r.prototype.validate=function(){if(-1!==(this.opts.in||this.opts.within||[]).indexOf(this.val))return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.exclusion,this.obj.addErrorMessage(t,{for:this.attr})},r}(h);var m={}.hasOwnProperty;const v=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)m.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Format",r.prototype.validate=function(){if(null==this.opts.with.exec(this.val))return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.invalid,this.obj.addErrorMessage(t,{for:this.attr})},r}(h);var b={}.hasOwnProperty;const E=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)b.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Inclusion",r.prototype.validate=function(){if(-1===(this.opts.in||this.opts.within||[]).indexOf(this.val))return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.inclusion,this.obj.addErrorMessage(t,{for:this.attr})},r}(h);var O={}.hasOwnProperty;const M=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)O.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Length",r.prototype.validate=function(){var t;if(null!=this.val&&null!==(t=null!=this._range()[0]&&null!=this._range()[1]&&this._range()[0]===this._range()[1]&&this.val.length!==this._range()[0]?this._selectErrorMessage("wrong_length",this._range()[0]):null!=this._range()[0]&&this.val.length<this._range()[0]?this._selectErrorMessage("too_short",this._range()[0]):null!=this._range()[1]&&this.val.length>this._range()[1]?this._selectErrorMessage("too_long",this._range()[1]):null))return this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._range=function(){return[this.opts.minimum||this.opts.is||null!=this.opts.within&&this.opts.within[0]||null,this.opts.maximum||this.opts.is||null!=this.opts.within&&this.opts.within[1]||null]},r.prototype._selectErrorMessage=function(t,r){var e,n,i,a,u;if(1===r)return s[o.locale].errors.messages[t].one;for(i=null,e=0,n=(a=["few","many"]).length;e<n;e++)if(u=a[e],this._checkVariant(u,r)){i=s[o.locale].errors.messages[t][u];break}return null==i&&(i=s[o.locale].errors.messages[t].other),null!=this.opts.message&&(i=this.opts.message),/%{count}/.exec(i)&&(i=i.replace("%{count}",r)),i},r.prototype._checkVariant=function(t,r){if(null!=s[o.locale].variants[t])return s[o.locale].variants[t](r)},r}(h);var w={}.hasOwnProperty;const P=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)w.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Numericality",r.prototype.validate=function(){return isNaN(this.val)?this._addNaNErrorMessage():null!=this.opts.only_integer&&Number(this.val)!==parseInt(this.val)?this._addIntErrorMessage():null!=this.opts.greater_than&&Number(this.val)<=this.opts.greater_than?this._addGreatherThanErrorMessage():null!=this.opts.greater_than_or_equal_to&&Number(this.val)<this.opts.greater_than_or_equal_to?this._addGreatherThanOrEqualToErrorMessage():null!=this.opts.equal_to&&Number(this.val)!==this.opts.equal_to?this._addEqualToErrorMessage():null!=this.opts.less_than&&Number(this.val)>=this.opts.less_than?this._addLessThanErrorMessage():null!=this.opts.less_than_or_equal_to&&Number(this.val)>this.opts.less_than_or_equal_to?this._addLessThanOrEqualToErrorMessage():null!=this.opts.other_than&&Number(this.val)===this.opts.other_than?this._addOtherThanErrorMessage():null!=this.opts.odd&&Number(this.val)%2!=1?this._addOddErrorMessage():null!=this.opts.even&&Number(this.val)%2!=0?this._addEvenErrorMessage():void 0},r.prototype._addNaNErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.not_a_number,this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addIntErrorMessage=function(){var t;return t=s[o.locale].errors.messages.not_an_integer,this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addGreatherThanErrorMessage=function(){var t;return t=(t=s[o.locale].errors.messages.greater_than).replace("%{count}",this.opts.greater_than),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addGreatherThanOrEqualToErrorMessage=function(){var t;return t=(t=s[o.locale].errors.messages.greater_than_or_equal_to).replace("%{count}",this.opts.greater_than_or_equal_to),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addEqualToErrorMessage=function(){var t;return t=(t=s[o.locale].errors.messages.equal_to).replace("%{count}",this.opts.equal_to),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addLessThanErrorMessage=function(){var t;return t=(t=s[o.locale].errors.messages.less_than).replace("%{count}",this.opts.less_than),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addLessThanOrEqualToErrorMessage=function(){var t;return t=(t=s[o.locale].errors.messages.less_than_or_equal_to).replace("%{count}",this.opts.less_than_or_equal_to),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addOtherThanErrorMessage=function(){var t;return t=(t=s[o.locale].errors.messages.other_than).replace("%{count}",this.opts.other_than),this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addOddErrorMessage=function(){var t;return t=s[o.locale].errors.messages.odd,this.obj.addErrorMessage(t,{for:this.attr})},r.prototype._addEvenErrorMessage=function(){var t;return t=s[o.locale].errors.messages.even,this.obj.addErrorMessage(t,{for:this.attr})},r}(h);var j={}.hasOwnProperty;const N=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)j.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Presence",r.prototype.validate=function(){if("string"==typeof this.val){if(null!=this.val&&this.val.length>0)return}else if(null!=this.val)return;return this._addErrorMessage()},r.prototype._addErrorMessage=function(){var t;return t=null!=this.opts.message?this.opts.message:s[o.locale].errors.messages.blank,this.obj.addErrorMessage(t,{for:this.attr})},r}(h);var S,T={}.hasOwnProperty;S=function(t){function r(){r.__super__.constructor.call(this)}return function(t,r){for(var e in r)T.call(r,e)&&(t[e]=r[e]);function o(){this.constructor=t}o.prototype=r.prototype,t.prototype=new o,t.__super__=r.prototype}(r,t),r.identity="Size",r.prototype.validate=function(){return M.instance(this.obj,this.attr,this.opts).validate()},r}(h);const q={Absence:f,Base:h,Confirmation:d,Exclusion:y,Format:v,Inclusion:E,Length:M,Numericality:P,Presence:N,Size:S};var R,A=function(t){var r="";return Object.keys(t).forEach((function(e){""!==r&&(r="".concat(r,"&")),r="".concat(r).concat(e,"=").concat(encodeURIComponent(t[e]))})),r},I=function(t){var r={};if(!t)return r;var e=["resource","total","count"];return Object.keys(t).forEach((function(o){-1===e.indexOf(o)&&(r[o]=t[o])})),r},k=function(t,r,e){var o=arguments.length>3&&void 0!==arguments[3]?arguments[3]:{},s=I(e),n="GET"===t?"".concat(r,"?").concat(A(s)):r,i=document.querySelector("meta[name='csrf-token']"),a=new XMLHttpRequest;return a.withCredentials=!0===o.cookiesByCORS,a.open(t,n),a.setRequestHeader("Accept","application/json"),a.setRequestHeader("Content-Type","application/json"),i&&a.setRequestHeader("X-CSRF-Token",i.content),null!=o.authorizationHeader&&a.setRequestHeader("Authorization",o.authorizationHeader),a.send(JSON.stringify(s)),a};R=function(){function t(t){null==t&&(t={}),this.id=null,this.errors=null,this.resource=t.resource,null!=this.constructor.attributes&&this.__initAttributes(),null!=t&&this.__assignAttributes(t)}return t.getIdentity=function(){if(null!=this.identity)return this.identity;throw"Specify Model's identity!"},t.getRemoteName=function(){return null!=this.remoteName?this.remoteName:this.getIdentity()},t.all=function(t){return null==t&&(t={}),this.get("all",t)},t.get=function(t,r){return null==r&&(r={}),this.__send("GET",t,r)},t.post=function(t,r){return null==r&&(r={}),this.__send("POST",t,r)},t.put=function(t,r){return null==r&&(r={}),this.__send("PUT",t,r)},t.patch=function(t,r){return null==r&&(r={}),this.__send("PATCH",t,r)},t.delete=function(t,r){return null==r&&(r={}),this.__send("DELETE",t,r)},t.find=function(t){var r,e,o,s,n;return s={},"object"==typeof t?(s=t,r=t.id,delete s.id):r=t,o=this.__getResourcesUrl(s)+"/"+r,e=k("GET",o,s,this.__requestOpts()),new Promise((n=this,function(r,o){return e.onerror=function(t){return o(t)},e.onload=function(e){var o;if(404!==e.target.status)return o=JSON.parse(e.target.response),r(n.__initFromJSON(o,t.resource));r(null)}}))},t.getAttribRemoteName=function(t){return null==this.attributes||null==this.attributes[t]?null:null==this.attributes[t].remoteName?t:this.attributes[t].remoteName},t.getResourcesUrlParams=function(t){var r,e,o,s;for(s=this.__getResourcesUrl({resource:t.resource}),o=/:(\w+)\/?/,e=[];r=o.exec(s);)e.push(r[1]),s=s.replace(r[0],r[1]);return e},t.__getResourcesUrl=function(t){var r,e;return e=null==this.resources?"/"+this.getRemoteName().toLowerCase()+"s":t.resource?this.resources[t.resource].url:null!=o.scope&&null!=this.resources[o.scope]?this.resources[o.scope].url:this.resources.url,null!=this.protocolWithHost?e=""+this.protocolWithHost+e:null!=o.protocolWithHost&&(e=""+o.protocolWithHost+e),null==(r=/:([a-zA-Z]+)\/?/.exec(e))||(null!=t[r[1]]?(e=e.replace(":"+r[1],t[r[1]]),delete t[r[1]]):null!=t.obj&&null!=t.obj[r[1]]&&(e=e.replace(":"+r[1],t.obj[r[1]]))),e},t.__requestOpts=function(){return{authorizationHeader:o.authorizationHeader||this.authorizationHeader,cookiesByCORS:null!=this.cookiesByCORS?this.cookiesByCORS:o.cookiesByCORS}},t.__page=function(t,r,e){var o,s,n;return s=r.url,r.params[r.pageParam]=t,o=k(r.method,s,r.params,this.__requestOpts()),new Promise((n=this,function(t,s){return o.onerror=function(t){return s(t)},o.onload=function(o){var s,i,a,u,l,c,h,p,f,_;if((s=JSON.parse(o.target.response)).constructor===Array)for(i=0,l=s.length;i<l;i++)p=s[i],h=n.__initFromJSON(p,r.resource),e.push(h);else if(null!=s.resources){for(e.constructor===Array&&(e={resources:[],count:0}),a=0,c=(f=s.resources).length;a<c;a++)p=f[a],h=n.__initFromJSON(p,r.resource),e.resources.push(h);e.count=s.count}else for(u in s)_=s[u],e[u]=_;return t(e)}}))},t.__paginate=function(t){var r,e;return r={method:t.method,url:t.url,params:t.params,pageParam:t.pageParam,resource:t.resource},this.__page(t.pageNum||1,r,[]).then((e=this,function(o){var s,n,i,a,u,l,c;if(c=o.count||t.total,u=Promise.resolve(o),null!=t.pageNum)return u;if(c<=t.perPage)return u;if((a=parseInt(c/t.perPage))!==c/t.perPage&&(a+=1),1===a)return u;for(n=i=2,l=a;2<=l?i<=l:i>=l;n=2<=l?++i:--i)s=function(t){return u=u.then((function(s){return e.__page(t,r,o)}))},s(n);return u}))},t.__getPaginationParam=function(t){var r,e,s,n,i,a;return r="page",null!=t&&null!=this.resources&&this.resources[t]?(null!=(e=this.resources[t].paginate)?e.param:void 0)||r:null!=o.scope&&null!=this.resources&&null!=this.resources[o.scope]?(null!=(s=this.resources[o.scope])&&null!=(n=s.paginate)?n.param:void 0)||r:null!=(null!=(i=this.resources)&&null!=(a=i.paginate)?a.param:void 0)?this.resources.paginate.param:r},t.__getPaginationPer=function(t){var r,e,s,n,i;return null!=t&&null!=this.resources&&this.resources[t]?null!=(r=this.resources[t].paginate)?r.per:void 0:null!=o.scope&&null!=this.resources&&null!=this.resources[o.scope]?null!=(e=this.resources[o.scope])&&null!=(s=e.paginate)?s.per:void 0:null!=(null!=(n=this.resources)&&null!=(i=n.paginate)?i.per:void 0)?this.resources.paginate.per:null},t.__send=function(t,r,e){var o,s;return s=this.__getResourcesUrl(e),"all"!==r&&(s=s+"/"+r),o={method:t,url:s,params:e,resource:e.resource,perPage:this.__getPaginationPer(e.resource),pageNum:e.page,pageParam:this.__getPaginationParam(e.resource),total:e.total||e.count},this.__paginate(o)},t.__initFromJSON=function(t,r){var e;return(e=new this(t)).resource=r,c.add(e),e},t.prototype.setResource=function(t){return this.resource=t},t.prototype.getIdentity=function(){return this.constructor.getIdentity()},t.prototype.getAttrRemoteName=function(t){return null==this.constructor.attributes||null==this.constructor.attributes[t]?null:this.constructor.attributes[t].remoteName||t},t.prototype.getAttrName=function(t){var r,e;if(null==this.constructor.attributes)return t;if(null!=this.constructor.attributes[t])return t;for(r in e=this.constructor.attributes)if(e[r].remoteName===t)return r;return t},t.prototype.getAttrType=function(t){return null==this.constructor.attributes||null==this.constructor.attributes[t]?null:this.constructor.attributes[t].type},t.prototype.assignAttr=function(t,r){var e;if(e=this.getAttrType(t),null!=r){switch(e){case"Date":r=new Date(Date.parse(r));break;case"Integer":case"Int":r=parseInt(r);break;case"Float":r=parseFloat(r);break;case"Boolean":case"Bool":r="boolean"==typeof r?r:Boolean(parseInt(r));break;case"Number":r=Number(r);break;case"String":r=String(r)}return this[t]=r}this[t]=null},t.prototype.attributes=function(){var t,r,e;if(t={id:this.id},null==this.constructor.attributes)return t;for(r in e=this.constructor.attributes)e[r],t[r]=this[r];return t},t.prototype.isValid=function(){var t,r,e,o,s,n,i,a,u,l,c;if(null==this.constructor.attributes)return!0;for(o in this.errors=null,n=this.constructor.attributes)if(null!=(t=n[o]).validations)for(u in i=t.validations)l=i[u],null!=this.id&&"create"===l.on||null==this.id&&"update"===l.on||(null==l.if||l.if(this))&&(c=u.charAt(0).toUpperCase()+u.slice(1),null!=q[c]?(s=this.__processedValidationSettings(l),q[c].instance(this,o,s).validate()):console.warn('"'+c+'" validator is not implemented!'));if(null!=this.constructor.validate)for(r=0,e=(a=this.constructor.validate).length;r<e;r++)this[a[r]]();return null==this.errors},t.prototype.isInvalid=function(){return!this.isValid()},t.prototype.isEmpty=function(){var t,r;for(t in r=this.attributes())if(r[t],null!==this[t])return!1;return!0},t.prototype.addErrorMessage=function(t,r){return null==r&&(r={}),null==this.errors&&(this.errors={}),null==this.errors[r.for]&&(this.errors[r.for]=[]),this.errors[r.for].push(t)},t.prototype.save=function(){var t,r,e;return t=null!=this.id?"PUT":"POST",r=k(t,this.__getResourceUrl(),this.serialize(),this.constructor.__requestOpts()),new Promise((e=this,function(t,o){return r.onerror=function(t){return o(t)},r.onload=function(r){var o;if(!(o=JSON.parse(r.target.response)).success)return null!=o.errors&&e.__assignRemoteErrorMessages(o.errors),t(o);t(o)}}))},t.prototype.updateAttribute=function(t){var r,e;return r=k("PUT",this.__getResourceUrl(),this.serialize(t),this.constructor.__requestOpts()),new Promise((e=this,function(t,o){return r.onerror=function(t){return o(t)},r.onload=function(r){var s;return r.target.status>=200&&r.target.status<400?(s=JSON.parse(r.target.response)).success?void t(s):(null!=s.errors&&e.__assignRemoteErrorMessages(s.errors),t(s)):r.target.status>=500?o(r):void 0}}))},t.prototype.serialize=function(t){var r,e,o,s;if(null==t&&(t=null),null==this.constructor.attributes)return{};for(t in(e={})[o=this.constructor.getRemoteName().toLowerCase()]={},r={},null!=t?r[t]=null:r=this.constructor.attributes,r)r[t],s=this.getAttrRemoteName(t),e[o][s]=this[t];return e},t.prototype.reload=function(){var t,r,e,o,s;for(t={id:this.id,resource:this.resource},r=0,e=(s=this.constructor.getResourcesUrlParams({resource:this.resource})).length;r<e;r++)t[o=s[r]]=this[o];return this.constructor.find(t)},t.prototype.changes=function(){var t,r,e,o,s;for(r in o={},t=c.find(this.getIdentity(),this.id),e=this.attributes())if((s=e[r])!==t[r]){if(null!=s&&s.constructor===Date&&t[r]-s==0)continue;s!==t[r]&&(o[r]={is:t[r],was:s})}return o},t.prototype.applyChanges=function(){var t,r,e,o;for(t in e=[],r=this.changes())o=r[t],e.push(this[t]=o.is);return e},t.prototype.toKey=function(){return this.getIdentity().toLowerCase()+"_"+this.id},t.prototype.get=function(t,r){return null==r&&(r={}),this.__send("GET",t,r)},t.prototype.post=function(t,r){return null==r&&(r={}),this.__send("POST",t,r)},t.prototype.put=function(t,r){return null==r&&(r={}),this.__send("PUT",t,r)},t.prototype.patch=function(t,r){return null==r&&(r={}),this.__send("PATCH",t,r)},t.prototype.delete=function(t,r){return null==r&&(r={}),this.__send("DELETE",t,r)},t.prototype.__send=function(t,r,e){var o,s;return s=this.__getResourceUrl(),null!=r&&(s=s+"/"+r),o=k(t,s,e,this.constructor.__requestOpts()),new Promise((function(t,r){return o.onerror=function(t){return r(t)},o.onload=function(o){return o.target.status>=200&&o.target.status<400?(e=JSON.parse(o.target.response),t(e)):o.target.status>=500?r(o):void 0}}))},t.prototype.__assignAttributes=function(t){var r,e,o,s;for(e in o=[],t)s=t[e],r=this.getAttrName(e),o.push(this.assignAttr(r,s));return o},t.prototype.__initAttributes=function(){var t,r,e;for(t in e=[],r=this.constructor.attributes)r[t],e.push(this[t]=null);return e},t.prototype.__assignRemoteErrorMessages=function(t){var r,e,o,s,n;for(s in n=[],t)o=t[s],r=this.getAttrName(s),n.push(function(){var t,s,n;for(n=[],t=0,s=o.length;t<s;t++)e=o[t],n.push(this.addErrorMessage(e,{for:r}));return n}.call(this));return n},t.prototype.__getResourceUrl=function(){var t;return t=this.constructor.__getResourcesUrl({resource:this.resource,obj:this}),null==this.id?t:t+"/"+this.id},t.prototype.__processedValidationSettings=function(t){var r,e,o;for(r in o={},t)e=t[r],o[r]="function"==typeof e?e(this):e;return o},t}();const C=R;function H(t,r){var e=Object.keys(t);if(Object.getOwnPropertySymbols){var o=Object.getOwnPropertySymbols(t);r&&(o=o.filter((function(r){return Object.getOwnPropertyDescriptor(t,r).enumerable}))),e.push.apply(e,o)}return e}function x(t,r,e){return r in t?Object.defineProperty(t,r,{value:e,enumerable:!0,configurable:!0,writable:!0}):t[r]=e,t}C.prototype.clone=function(){return new this.constructor(function(t){for(var r=1;r<arguments.length;r++){var e=null!=arguments[r]?arguments[r]:{};r%2?H(Object(e),!0).forEach((function(r){x(t,r,e[r])})):Object.getOwnPropertyDescriptors?Object.defineProperties(t,Object.getOwnPropertyDescriptors(e)):H(Object(e)).forEach((function(r){Object.defineProperty(t,r,Object.getOwnPropertyDescriptor(e,r))}))}return t}({},this.attributes()))};const U={Base:C};return r})()})); |
@@ -14,3 +14,3 @@ /* eslint-env node */ | ||
return src; | ||
} | ||
}, | ||
}; |
@@ -8,4 +8,4 @@ /* eslint-env node */ | ||
"^.+\\.js$": "babel-jest", | ||
"^.+\\.coffee$": "<rootDir>/jest.coffeescript.preprocessor.js" | ||
} | ||
"^.+\\.coffee$": "<rootDir>/jest.coffeescript.preprocessor.js", | ||
}, | ||
}; |
{ | ||
"name": "loco-js-model", | ||
"version": "1.1.1", | ||
"version": "2.0.0", | ||
"description": "Model part of loco-js", | ||
@@ -8,5 +8,5 @@ "main": "dist/loco-model.js", | ||
"server": "node dev/server.js", | ||
"start": "webpack --watch --config webpack.config.js", | ||
"start": "webpack --mode=development -d inline-source-map -c webpack.config.js --watch", | ||
"test": "jest", | ||
"build": "webpack -p --config webpack.config.js", | ||
"build": "webpack --mode=production --node-env=production -c webpack.config.js --progress", | ||
"lint-staged": "lint-staged" | ||
@@ -31,3 +31,2 @@ }, | ||
}, | ||
"dependencies": {}, | ||
"devDependencies": { | ||
@@ -37,22 +36,22 @@ "@babel/core": "^7.11.1", | ||
"@babel/preset-env": "^7.11.0", | ||
"babel-eslint": "^10.1.0", | ||
"babel-jest": "^25.5.1", | ||
"@babel/eslint-parser": "^7.16.3", | ||
"babel-jest": "^26.6.3", | ||
"babel-loader": "^8.1.0", | ||
"coffee-loader": "^0.9.0", | ||
"coffeescript": "^1.12.7", | ||
"eslint": "^6.8.0", | ||
"eslint-config-prettier": "^6.11.0", | ||
"eslint": "^8.3.0", | ||
"eslint-config-prettier": "^8.3.0", | ||
"eslint-plugin-import": "^2.22.0", | ||
"eslint-plugin-jest": "^23.20.0", | ||
"eslint-plugin-prettier": "^3.1.4", | ||
"eslint-plugin-jest": "^25.3.0", | ||
"eslint-plugin-prettier": "^4.0.0", | ||
"express": "^4.17.1", | ||
"husky": "^3.1.0", | ||
"jest": "^25.5.4", | ||
"lint-staged": "^9.5.0", | ||
"jest": "^26.6.3", | ||
"lint-staged": "^12.1.2", | ||
"nock": "^13.0.5", | ||
"prettier": "^1.19.1", | ||
"webpack": "^4.44.1", | ||
"webpack-cli": "^3.3.12", | ||
"webpack-dev-middleware": "^3.7.2" | ||
"prettier": "^2.4.1", | ||
"webpack": "^5.64.3", | ||
"webpack-cli": "^4.9.1", | ||
"webpack-dev-middleware": "^5.2.2" | ||
} | ||
} |
@@ -70,2 +70,5 @@ 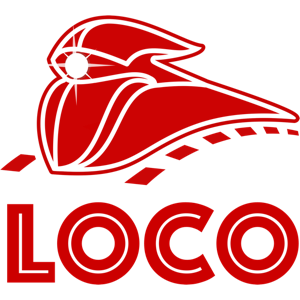 | ||
// Loco-JS-Model sends Authorization header in all XHR requests if provided | ||
Config.authorizationHeader = "Bearer XXX"; | ||
// If provided - Loco-JS-Model uses an absolute path instead of a site-root-relative path in all XHR requests | ||
@@ -102,2 +105,5 @@ Config.protocolWithHost = "http://localhost:3000"; | ||
static identity = "Coupon"; | ||
// (optional) it overrides the protocolWithHost passed during configuration | ||
static protocolWithHost = "https://myapp.test"; | ||
@@ -504,2 +510,8 @@ // You can fetch the same type of resource from different API endpoints. | ||
### 2.0.0 _(2022-02-03)_ | ||
* Ability to set an individual `protocolWithHost` for a given model | ||
* `Config.authorizationHeader` getter / setter | ||
* `Config` setters don't return a value | ||
### 1.1.1 _(2020-12-09)_ | ||
@@ -506,0 +518,0 @@ |
@@ -6,14 +6,4 @@ class Configurator { | ||
this.scopeVar = null; | ||
this.cookiesByCORSVar = false; | ||
} | ||
get cookiesByCORS() { | ||
return this.cookiesByCORSVar; | ||
} | ||
set cookiesByCORS(val) { | ||
this.cookiesByCORSVar = val; | ||
return this.cookiesByCORSVar; | ||
} | ||
get locale() { | ||
@@ -25,3 +15,2 @@ return this.localeVar; | ||
this.localeVar = val; | ||
return this.localeVar; | ||
} | ||
@@ -36,10 +25,7 @@ | ||
this.protocolWithHostVar = null; | ||
return this.protocolWithHostVar; | ||
} | ||
if (val[val.length - 1] === "/") { | ||
} else if (val[val.length - 1] === "/") { | ||
this.protocolWithHostVar = val.slice(0, val.length - 1); | ||
return this.protocolWithHostVar; | ||
} else { | ||
this.protocolWithHostVar = val; | ||
} | ||
this.protocolWithHostVar = val; | ||
return this.protocolWithHostVar; | ||
} | ||
@@ -53,3 +39,2 @@ | ||
this.scopeVar = val; | ||
return this.scopeVar; | ||
} | ||
@@ -56,0 +41,0 @@ } |
@@ -1,9 +0,8 @@ | ||
import Config from "../config"; | ||
import { toURIParams } from "./obj"; | ||
const filterParams = data => { | ||
const filterParams = (data) => { | ||
const params = {}; | ||
if (!data) return params; | ||
const forbidden = ["resource", "total", "count"]; | ||
Object.keys(data).forEach(prop => { | ||
Object.keys(data).forEach((prop) => { | ||
if (forbidden.indexOf(prop) === -1) { | ||
@@ -16,3 +15,3 @@ params[prop] = data[prop]; | ||
export const sendReq = (httpMeth, url, data) => { | ||
export const sendReq = (httpMeth, url, data, opts = {}) => { | ||
const params = filterParams(data); | ||
@@ -22,2 +21,3 @@ const finalURL = httpMeth === "GET" ? `${url}?${toURIParams(params)}` : url; | ||
const req = new XMLHttpRequest(); | ||
req.withCredentials = opts.cookiesByCORS === true ? true : false; | ||
req.open(httpMeth, finalURL); | ||
@@ -29,3 +29,5 @@ req.setRequestHeader("Accept", "application/json"); | ||
} | ||
req.withCredentials = Config.cookiesByCORS; | ||
if (opts.authorizationHeader != null) { | ||
req.setRequestHeader("Authorization", opts.authorizationHeader); | ||
} | ||
req.send(JSON.stringify(params)); | ||
@@ -32,0 +34,0 @@ return req; |
@@ -1,4 +0,4 @@ | ||
export const toURIParams = obj => { | ||
export const toURIParams = (obj) => { | ||
let str = ""; | ||
Object.keys(obj).forEach(key => { | ||
Object.keys(obj).forEach((key) => { | ||
if (str !== "") str = `${str}&`; | ||
@@ -5,0 +5,0 @@ str = `${str}${key}=${encodeURIComponent(obj[key])}`; |
import en from "./locales/en.coffee"; | ||
const I18n = { | ||
en | ||
en, | ||
}; | ||
export default I18n; |
@@ -19,3 +19,3 @@ /* | ||
const findPosition = arr => { | ||
const findPosition = (arr) => { | ||
let idx = arr.length; | ||
@@ -53,9 +53,6 @@ arr.find((element, index) => { | ||
const subscribe = args => { | ||
const subscribe = (args) => { | ||
const forExistingElement = () => {}; | ||
if (typeof args.to === "object") { | ||
const idx = connect( | ||
args.with, | ||
{ with: args.to } | ||
); | ||
const idx = connect(args.with, { with: args.to }); | ||
if (idx === null) return forExistingElement; | ||
@@ -67,3 +64,3 @@ return () => { | ||
const idx = addCollection(args.to.getIdentity(), { | ||
to: args.with | ||
to: args.with, | ||
}); | ||
@@ -79,3 +76,3 @@ if (idx === null) return forExistingElement; | ||
const add = obj => { | ||
const add = (obj) => { | ||
const identity = obj.getIdentity(); | ||
@@ -114,3 +111,3 @@ if (imap[identity] === undefined) imap[identity] = {}; | ||
find, | ||
findConnected | ||
findConnected, | ||
}; |
import Base from "./base.coffee"; | ||
Base.prototype.clone = function() { | ||
Base.prototype.clone = function () { | ||
return new this.constructor({ ...this.attributes() }); | ||
@@ -5,0 +5,0 @@ }; |
@@ -22,5 +22,5 @@ import Absence from "./validators/absence.coffee"; | ||
Presence, | ||
Size | ||
Size, | ||
}; | ||
export default Validators; |
@@ -18,9 +18,9 @@ /* eslint-env node */ | ||
transpile: { | ||
presets: ["@babel/preset-env"] | ||
} | ||
} | ||
} | ||
] | ||
} | ||
] | ||
presets: ["@babel/preset-env"], | ||
}, | ||
}, | ||
}, | ||
], | ||
}, | ||
], | ||
}, | ||
@@ -31,4 +31,4 @@ output: { | ||
library: "LocoModel", | ||
libraryTarget: "umd" | ||
} | ||
libraryTarget: "umd", | ||
}, | ||
}; |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is not supported yet
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
License Policy Violation
LicenseThis package is not allowed per your license policy. Review the package's license to ensure compliance.
Found 1 instance in 1 package
112177
1507
539