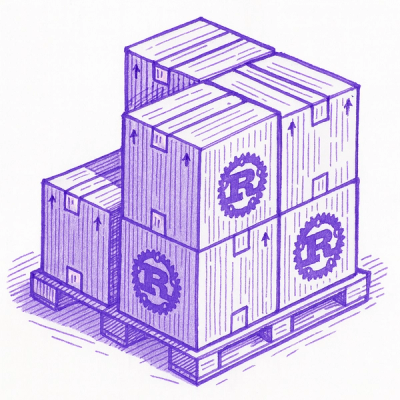
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
A drop-in replacement for Underscore.js delivering performance, bug fixes, and additional features.
Lodash is a JavaScript library that provides utility functions for common programming tasks using a functional programming paradigm. It includes functions for manipulating and traversing arrays, objects, and strings, as well as utilities for functions, language, math, number, object, sequence, and utility methods.
Array Manipulation
Lodash provides a rich set of array manipulation functions such as map, filter, find, and sort. The code sample demonstrates sorting an array in ascending order using a custom comparator.
[3, 2, 1].sort(_.compareWith(function(a, b) { return a - b; }))
Object Manipulation
Lodash allows for easy manipulation and traversal of objects. The code sample shows how to assign properties from source objects to a destination object.
_.assign({ 'a': 1 }, { 'b': 2 }, { 'c': 3 })
String Manipulation
Lodash includes functions to manipulate strings, such as converting to different cases, trimming, padding, etc. The code sample demonstrates converting a string to kebab-case.
_.kebabCase('Foo Bar')
Function Utilities
Lodash provides function utilities like debounce and throttle to control function invocation. The code sample shows a debounced function that will only be invoked after 250 milliseconds have passed without it being called again.
_.debounce(function() { console.log('Debounced'); }, 250)
Language Utilities
Lodash includes utilities for deep cloning, merging, and comparing objects. The code sample demonstrates deep cloning an object to ensure nested objects are cloned as well.
_.cloneDeep({ 'a': 1, 'b': { 'c': 2 } })
Underscore is a utility library with similar functionality to Lodash, offering a range of functions for manipulating arrays, objects, and functions. It is generally considered to be the predecessor to Lodash, which provides a superset of Underscore's features with additional performance optimizations.
Ramda is a functional programming library that emphasizes a more functional and composable approach compared to Lodash. It provides similar utilities but focuses on immutability and side-effect free functions, which can lead to a different programming style.
Immutable.js offers a different take on data manipulation by providing persistent immutable data structures. Unlike Lodash, which works with standard JavaScript objects and arrays, Immutable.js uses its own data structures, which can lead to better performance and easier reasoning about state changes in certain applications.
A drop-in replacement* for Underscore.js, from the devs behind jsPerf.com, delivering performance, bug fixes, and additional features.
Lo-Dash’s performance is gained by avoiding slower native methods, instead opting for simplified non-ES5 compliant methods optimized for common usage, and by leveraging function compilation to reduce the number of overall function calls.
We’ve got API docs, benchmarks, and unit tests.
Create your own benchmarks at jsPerf, or search for existing ones.
For a list of upcoming features, check out our roadmap.
For more information check out these screencasts over Lo-Dash:
fromIndex
argumentthis
bindingcallback
and thisArg
argumentsthisArg
argumentLo-Dash has been tested in at least Chrome 5-21, Firefox 1-15, IE 6-9, Opera 9.25-12, Safari 3-6, Node.js 0.4.8-0.8.8, Narwhal 0.3.2, RingoJS 0.8, and Rhino 1.7RC5.
Custom builds make it easy to create lightweight versions of Lo-Dash containing only the methods you need. To top it off, we handle all method dependency and alias mapping for you.
backbone
modifier argument.lodash backbone
csp
modifier argument.lodash csp
legacy
modifier argument.lodash legacy
mobile
modifier argument.lodash mobile
_.bindAll
, _.defaults
, and _.extend
in strict mode, may be created using the strict
modifier argument.lodash strict
underscore
modifier argument.lodash underscore
Custom builds may be created using the following commands:
category
argument to pass comma separated categories of methods to include in the build.lodash category=collections,functions
lodash category="collections, functions"
exclude
argument to pass comma separated names of methods to exclude from the build.lodash exclude=union,uniq,zip
lodash exclude="union, uniq, zip"
exports
argument to pass comma separated names of ways to export the LoDash
function.lodash exports=amd,commonjs,node
lodash include="amd, commonjs, node"
iife
argument to specify code to replace the immediately-invoked function expression that wraps Lo-Dash.lodash iife="!function(window,undefined){%output%}(this)"
include
argument to pass comma separated names of methods to include in the build.lodash include=each,filter,map
lodash include="each, filter, map"
All arguments, except exclude
with include
and legacy
with csp
/mobile
, may be combined.
lodash backbone legacy exports=global category=utilities exclude=first,last
lodash -s underscore mobile strict exports=amd category=functions include=pick,uniq
The following options are also supported:
-c
, --stdout
Write output to standard output-h
, --help
Display help information-o
, --output
Write output to a given path/filename-s
, --silent
Skip status updates normally logged to the console-V
, --version
Output current version of Lo-DashThe lodash
command-line utility is available when Lo-Dash is installed as a global package (i.e. npm install -g lodash
).
Custom builds are saved to lodash.custom.js
and lodash.custom.min.js
.
In browsers:
<script src="lodash.js"></script>
Using npm:
npm install lodash
npm install -g lodash
In Node.js and RingoJS v0.8.0+:
var _ = require('lodash');
In RingoJS v0.7.0-:
var _ = require('lodash')._;
In Rhino:
load('lodash.js');
In an AMD loader like RequireJS:
require({
'paths': {
'underscore': 'path/to/lodash'
}
},
['underscore'], function(_) {
console.log(_.VERSION);
});
length
property [#148, #154, #252, #448, #659, test]length
properties are treated like regular objects [#741, test]collection
arguments [#247, #276, #561, test]_.clone
should allow deep
cloning [#595, test]_.contains
should work with strings [#667, test]_.countBy
and _.groupBy
should only add values to own, not inherited, properties [#736, test]_.extend
should recursively extend objects [#379, #718, test]_.forEach
should be chainable [#142, test]_.forEach
should allow exiting iteration early [#211, test]_.isElement
should use strict equality for its duck type check [#734, test]_.isEmpty
should support jQuery/MooTools DOM query collections [#690, test]_.isEqual
should return true
for like-objects from different documents [#733, test]_.isEqual
should use custom isEqual
methods before checking strict equality [#748, test]_.isObject
should avoid V8 bug #2291 [#605, test]_.isNaN(new Number(NaN))
should return true
[#749, test]_.keys
should work with arguments
objects cross-browser [#396, test]_.range
should coerce arguments to numbers [#634, #683, test]_.reduceRight
should pass correct callback arguments when iterating objects [test]_.sortedIndex
should support arrays with high length
values [#735, test]_.throttle
should work when called in a loop [#502, test]_.toArray
uses custom toArray
methods of arrays and strings [#747, test]_.bind
_.bindAll
_.clone
_.compact
_.contains
, _.include
_.defaults
_.defer
_.difference
_.each
_.every
, _.all
_.extend
_.filter
, _.select
_.find
, _.detect
_.flatten
_.forEach
, _.each
_.functions
, _.methods
_.groupBy
_.indexOf
_.intersection
_.invert
_.invoke
_.isArguments
_.isDate
_.isEmpty
_.isFinite
_.isFunction
_.isObject
_.isNumber
_.isRegExp
_.isString
_.keys
_.lastIndexOf
_.map
, _.collect
_.max
_.memoize
_.min
_.mixin
_.omit
_.pairs
_.pick
_.pluck
_.reduce
, _.foldl
, _.inject
_.reject
_.result
_.shuffle
_.some
, _.any
_.sortBy
_.sortedIndex
_.template
_.throttle
_.times
_.toArray
_.union
_.uniq
, _.unique
_.values
_.without
_.wrap
_.zip
_(…)
method wrappers_.zipObject
to _.object
_.drop
with _.omit
_.drop
alias _.rest
_.result
to the backbone
buildexports
, iife
, -c
/--stdout
, -o
/--output
, and -s
/--silent
build optionsisPlainObject
works with objects from other documements_.isEqual
compares values with circular references correctly_.merge
work with four or more arguments_.sortBy
performs a stable sort for undefined
values_.template
works with "interpolate" delimiters containing ternary operators_chain
and _wrapped
double-underscored to avoid conflictsminify.js
support underscore.js
mobile
and underscore
builds_.isEqual
and _.size
The full changelog is available here.
Lo-Dash is part of the BestieJS “Best in Class” module collection. This means we promote solid browser/environment support, ES5 precedents, unit testing, and plenty of documentation.
FAQs
Lodash modular utilities.
The npm package lodash receives a total of 70,130,400 weekly downloads. As such, lodash popularity was classified as popular.
We found that lodash demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.