What is loglevel-plugin-prefix?
The loglevel-plugin-prefix npm package is a plugin for the loglevel logging library that allows you to add customizable prefixes to your log messages. This can be useful for adding context to your logs, such as timestamps, log levels, or custom tags.
What are loglevel-plugin-prefix's main functionalities?
Basic Prefixing
This feature allows you to add a basic prefix to your log messages. By default, it adds the log level as a prefix.
const log = require('loglevel');
const prefix = require('loglevel-plugin-prefix');
prefix.apply(log);
log.info('This is an info message');
Custom Prefix Format
This feature allows you to customize the format of the prefix. You can include a timestamp, log level, and logger name in the prefix.
const log = require('loglevel');
const prefix = require('loglevel-plugin-prefix');
prefix.apply(log, {
template: '[%t] %l (%n):',
timestampFormatter: date => date.toISOString(),
levelFormatter: level => level.toUpperCase(),
nameFormatter: name => name || 'global'
});
log.info('This is an info message');
Custom Prefix Function
This feature allows you to define a custom function to format the prefix. This gives you complete control over how the prefix is generated.
const log = require('loglevel');
const prefix = require('loglevel-plugin-prefix');
prefix.apply(log, {
format(level, name, timestamp) {
return `${timestamp} [${level}] ${name}:`;
}
});
log.info('This is an info message');
Other packages similar to loglevel-plugin-prefix
winston
Winston is a versatile logging library for Node.js that supports multiple transports, custom formats, and log levels. It offers more advanced features compared to loglevel-plugin-prefix, such as log rotation and integration with various logging services.
bunyan
Bunyan is another logging library for Node.js that provides a JSON-based logging format. It includes features like log levels, serializers, and streams. Bunyan is more focused on structured logging compared to the prefixing capabilities of loglevel-plugin-prefix.
pino
Pino is a fast and low-overhead logging library for Node.js. It supports JSON logging, log levels, and custom serializers. Pino is designed for performance and is more lightweight compared to loglevel-plugin-prefix.
loglevel-plugin-prefix
Plugin for loglevel message prefixing.


Installation
npm i loglevel-plugin-prefix --save
API
This plugin is under active development and should be considered as an unstable. No guarantees regarding API stability are made. Backward compatibility is guaranteed only by path releases.
reg(loglevel)
This method must be called before any calling the apply method.
Parameters
loglevel
- the root logger, imported from loglevel package
apply(logger, options)
This method applies the plugin to the logger. Before using this method, the reg
method must be called, otherwise a warning will be logged. From the next release, the call apply before reg will throw an error.
Parameters
logger
- a loglevel logger
options
- an optional configuration object
var defaults = {
template: '[%t] %l:',
timestampFormatter: function (date) {
return date.toTimeString().replace(/.*(\d{2}:\d{2}:\d{2}).*/, '$1');
},
levelFormatter: function (level) {
return level.toUpperCase();
},
nameFormatter: function (name) {
return name || 'root';
},
format: undefined
};
Plugin formats the prefix using template option as a printf-like format. The template is a string containing zero or more placeholder tokens. Each placeholder token is replaced with the value from loglevel messages parameters. Supported placeholders are:
%t
- timestamp of message%l
- level of message%n
- name of logger
The timestampFormatter, levelFormatter and nameFormatter is a functions for formatting corresponding values.
Alternatively, you can use format option. This is a function that receives formatted values (level, logger, timestamp) and should returns a prefix string. If the format option is present, the template option are ignored.
Usage
Browser directly
<script src="https://unpkg.com/loglevel/dist/loglevel.min.js"></script>
<script src="https://unpkg.com/loglevel-plugin-prefix@^0.8/dist/loglevel-plugin-prefix.min.js"></script>
<script>
var logger = log.noConflict();
var prefixer = prefix.noConflict();
prefixer.reg(logger);
prefixer.apply(logger);
logger.warn('prefixed message');
</script>
Output
[16:53:46] WARN: prefixed message
Node
const chalk = require('chalk');
const log = require('loglevel');
const prefix = require('loglevel-plugin-prefix');
const colors = {
TRACE: chalk.magenta,
DEBUG: chalk.cyan,
INFO: chalk.blue,
WARN: chalk.yellow,
ERROR: chalk.red,
};
prefix.reg(log);
log.enableAll();
prefix.apply(log, {
format(level, name, timestamp) {
return `${chalk.gray(`[${timestamp}]`)} ${colors[level.toUpperCase()](level)} ${chalk.green(`(${name})`)}`;
},
});
const critical = log.getLogger('critical');
prefix.apply(critical, {
format(level, name, timestamp) {
return chalk.red(`[${timestamp}] ${level} (${name}):`);
},
});
log.trace('trace');
log.debug('debug');
critical.info('Something significant happened');
log.info('info');
log.warn('warn');
log.error('error');
Output
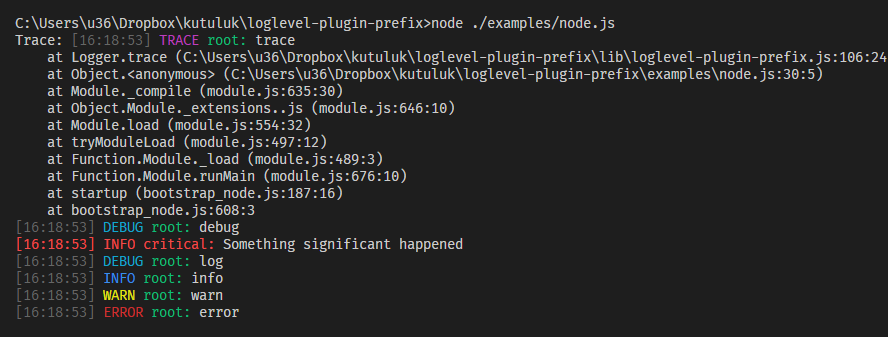
Custom options
import log from 'loglevel';
import prefix from 'loglevel-plugin-prefix';
log.enableAll();
prefix.apply(log, {
template: '[%t] %l (%n) static text:',
timestampFormatter(date) { return date.toISOString() },
levelFormatter(level) { return level.toUpperCase() },
nameFormatter(name) { return name || 'global' }
});
log.info('%s prefix', 'template');
const fn = (level, logger, timestamp) => {
return `[${timestamp}] ${label} (${name}) static text:`;
};
prefix.apply(log, { format: fn });
log.info('%s prefix', 'functional');
prefix.apply(log, { template: '[%t] %l (%n) static text:' });
log.info('again %s prefix', 'template');
Output
[2017-05-29T12:53:46.000Z] INFO (global) static text: template prefix
[2017-05-29T12:53:46.000Z] INFO (global) static text: functional prefix
[2017-05-29T12:53:46.000Z] INFO (global) static text: again template prefix
Option inheritance
const log = require('loglevel');
const prefix = require('../lib/loglevel-plugin-prefix');
prefix.reg(log);
log.enableAll();
log.info('root');
const chicken = log.getLogger('chicken');
chicken.info('chicken');
prefix.apply(chicken, { template: '%l (%n):' });
chicken.info('chicken');
prefix.apply(log);
log.info('root');
const egg = log.getLogger('egg');
egg.info('egg');
const fn = (level, logger) => `${level} (${logger}):`;
prefix.apply(egg, { format: fn });
egg.info('egg');
prefix.apply(egg, {
levelFormatter(level) {
return level.toLowerCase();
},
});
egg.info('egg');
chicken.info('chicken');
log.info('root');
Output
root
chicken
INFO (chicken): chicken
[13:20:24] INFO: root
[13:20:24] INFO: egg
INFO (egg): egg
info (egg): egg
INFO (chicken): chicken
[13:20:24] INFO: root