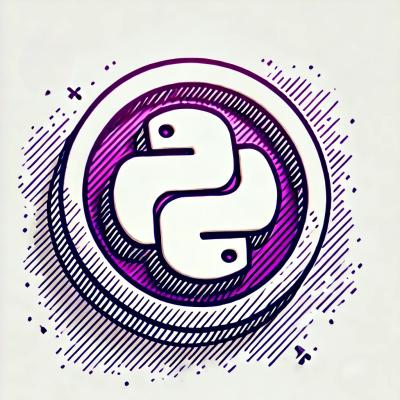
Security News
PyPI Introduces Digital Attestations to Strengthen Python Package Security
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Lowdb is a small local JSON database for Node, Electron, and the browser. It's a simple and lightweight way to store data locally using a JSON file. It is ideal for small projects, quick prototypes, and applications that do not require a full-fledged database.
Create and Read Data
This feature allows you to create and read data from a JSON file. The code initializes a lowdb instance, sets default values, adds a new post, and retrieves it.
const low = require('lowdb');
const FileSync = require('lowdb/adapters/FileSync');
const adapter = new FileSync('db.json');
const db = low(adapter);
db.defaults({ posts: [] }).write();
db.get('posts').push({ id: 1, title: 'lowdb is awesome' }).write();
const post = db.get('posts').find({ id: 1 }).value();
console.log(post);
Update Data
This feature allows you to update existing data in the JSON file. The code updates the title of the post with id 1 and retrieves the updated post.
db.get('posts').find({ id: 1 }).assign({ title: 'lowdb is super awesome' }).write();
const updatedPost = db.get('posts').find({ id: 1 }).value();
console.log(updatedPost);
Delete Data
This feature allows you to delete data from the JSON file. The code removes the post with id 1 and retrieves the remaining posts.
db.get('posts').remove({ id: 1 }).write();
const posts = db.get('posts').value();
console.log(posts);
NeDB is a lightweight JavaScript database that is similar to MongoDB but is meant for small projects. It supports indexing and querying and can be used both in Node.js and in the browser. Compared to lowdb, NeDB offers more advanced querying capabilities and indexing.
LokiJS is a fast, in-memory database for Node.js and browsers. It is designed for performance and can handle large datasets efficiently. LokiJS offers more advanced features like indexing, binary serialization, and live querying compared to lowdb.
json-server is a full fake REST API with zero coding in less than 30 seconds. It is ideal for quick prototyping and mocking REST APIs. Unlike lowdb, json-server is more focused on providing a RESTful interface to your JSON data.
Need a quick way to get a local database for a CLI, a small server or the browser?
const low = require('lowdb')
const storage = require('lowdb/file-sync')
const db = low('db.json', { storage })
db('posts').push({ title: 'lowdb is awesome'})
Database is automatically saved to db.json
{
"posts": [
{ "title": "lowdb is awesome" }
]
}
You can query and manipulate it using any lodash method.
db('posts').find({ title: 'lowdb is awesome' })
Please note that lowdb can only be run in one instance of Node, it doesn't support Cluster.
npm install lowdb --save
Lowdb is also very easy to learn since it has only 4 methods and properties.
lowdb powers json-server package, jsonplaceholder website and many other great projects.
Depending on the context, you can use different storages and formats.
Lowdb comes bundled with file-sync
, file-async
and browser
storages, but you can also write your own if needed.
For CLIs, it's easier to use lowdb/file-sync
synchronous file storage .
const low = require('lowdb')
const fileSync = require('lowdb/file-sync')
const db = low('db.json', fileSync)
db('users').push({ name: 'typicode' })
const user = db('users').find({ name: 'typicode' })
For servers, it's better to avoid blocking requests. Use lowdb/file-async
asynchronous file storage.
Important
const low = require('lowdb').
const storage = require('lowdb/file-async')
const db = low('db.json', { storage })
app.get('/posts/:id', (req, res) => {
// Returns a post
const post = db('posts').find({ id: req.params.id })
res.send(post)
})
app.post('/posts', (req, res) => {
// Returns a Promise that resolves to a post
db('posts')
.push(req.body)
.then(post => res.send(post))
})
In the browser, lowdb/browser
will add localStorage
support.
const low = require('lowdb')
const storage = require('lowdb/browser')
const db = low('db.json', { storage })
db('users').push({ name: 'typicode' })
const user = db('users').find({ name: 'typicode' })
For the best performance, use lowdb in-memory storage.
const low = require('lowdb')
const db = low()
db('users').push({ name: 'typicode' })
const user = db('users').find({ name: 'typicode' })
Please note that, as an alternative, you can also disable writeOnChange
if you want to control when data is written.
low([filename, [storage, [writeOnChange = true]]])
Creates a new database instance. Here are some examples:
low() // in-memory
low('db.json', { storage: /* */ }) // persisted
low('db.json', { storage: /* */ }, false) // auto write disabled
// To create read-only or write-only database
// pass only the read or write function.
// For example:
const fileSync = require('lowdb/fileSync')
// write-only
low('db.json', {
storage: { write: fileSync.write }
})
// read-only
low('db.json', {
storage: { read: fileSync.read }
})
Full method signature:
low(source, {
storage: {
read: (source, deserialize) => // obj
write: (dest, obj, serialize) => // undefined or a Promise
},
format: {
deserialize: (data) => // obj
serialize: (obj) => // data
}
}, writeOnChange)
db._
Database lodash instance. Use it to add your own utility functions or third-party mixins like underscore-contrib or underscore-db.
db._.mixin({
second: function(array) {
return array[1]
}
})
var song1 = db('songs').first()
var song2 = db('songs').second()
db.object
Use whenever you want to access or modify the underlying database object.
db.object // { songs: [ ... ] }
If you directly modify the content of the database object, you will need to manually call write
to persist changes.
// Delete an array
delete db.object.songs
db.write()
// Drop database
db.object = {}
db.write()
db.write([source])
Persists database using storage.write
method.
const db = low('db.json')
db.write() // writes to db.json
db.write('copy.json') // writes to copy.json
With lowdb, you get access to the entire lodash API, so there is many ways to query and manipulate data. Here are a few examples to get you started.
Please note that data is returned by reference, this means that modifications to returned objects may change the database. To avoid such behaviour, you need to use .cloneDeep()
.
Also, the execution of chained methods is lazy, that is, execution is deferred until .value()
is called.
Sort the top five songs.
db('songs')
.chain()
.where({published: true})
.sortBy('views')
.take(5)
.value()
Retrieve song titles.
db('songs').pluck('title')
Get the number of songs.
db('songs').size()
Make a deep clone of songs.
db('songs').cloneDeep()
Update a song.
db('songs')
.chain()
.find({ title: 'low!' })
.assign({ title: 'hi!'})
.value()
Remove songs.
db('songs').remove({ title: 'low!' })
Being able to retrieve data using an id can be quite useful, particularly in servers. To add id-based resources support to lowdb, you have 2 options.
underscore-db provides a set of helpers for creating and manipulating id-based resources.
var db = low('db.json')
db._.mixin(require('underscore-db'))
var songId = db('songs').insert({ title: 'low!' }).id
var song = db('songs').getById(songId)
uuid is more minimalist and returns a unique id that you can use when creating resources.
var uuid = require('uuid')
var songId = db('songs').push({ id: uuid(), title: 'low!' }).id
var song = db('songs').find({ id: songId })
By default, lowdb storages will use JSON
to parse
and stringify
database object.
But it's also possible to specify custom format.serializer
and format.deserializer
methods that will be passed by lowdb to storage.read
and storage.write
methods.
For example, if you want to store database in .bson
files (MongoDB file format):
const low = require('lowdb')
const storage = require('lowdb/file-sync')
const bson = require('bson')
const BSON = new bson.BSONPure.BSON()
low('db.bson', { storage, format: {
serialize: BSON.serialize
deserialize: BSON.deserialize
})
// Alternative ES2015 short syntax
const bson = require('bson')
const format = new bson.BSONPure.BSON()
low('db.bson', { storage, format })
Simply encrypt
and decrypt
data in format.serialize
and format.deserialize
methods.
For example, using cryptr:
const Cryptr = require("./cryptr"),
const cryptr = new Cryptr('my secret key')
const db = low('db.json', {
format: {
deserialize: (str) => {
const decrypted = cryptr.decrypt(str)
const obj = JSON.parse(decrypted)
return obj
},
serialize: (obj) => {
const str = JSON.stringify(obj)
const encrypted = cryptr.encrypt(str)
return encrypted
}
}
})
See changes for each version in the release notes.
lowdb is a convenient method for storing data without setting up a database server. It is fast enough and safe to be used as an embedded database.
However, if you seek high performance and scalability more than simplicity, you should probably stick to traditional databases like MongoDB.
MIT - Typicode
FAQs
Tiny local JSON database for Node, Electron and the browser
The npm package lowdb receives a total of 288,838 weekly downloads. As such, lowdb popularity was classified as popular.
We found that lowdb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports digital attestations, enhancing security and trust by allowing package maintainers to verify the authenticity of Python packages.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.