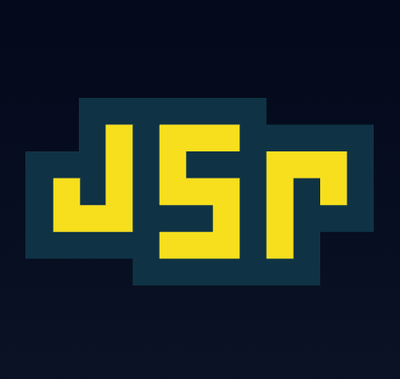
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Madge is a JavaScript library that helps you visualize and analyze the dependency graph of your project. It can be used to identify circular dependencies, generate dependency graphs, and more.
Generate Dependency Graph
This feature allows you to generate a dependency graph of your project. The code sample demonstrates how to use Madge to analyze the dependencies in a given project directory and output the result as an object.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
console.log(res.obj());
});
Identify Circular Dependencies
Madge can identify circular dependencies in your project. The code sample shows how to use Madge to find and log any circular dependencies in the specified project directory.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
console.log(res.circular());
});
Generate Graphviz DOT File
Madge can generate a Graphviz DOT file for visualizing the dependency graph. The code sample demonstrates how to generate and log the DOT representation of the dependency graph.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
return res.dot();
}).then((output) => {
console.log(output);
});
Create an Image of the Dependency Graph
Madge can create an image file of the dependency graph. The code sample shows how to generate an image of the dependency graph and save it as 'graph.png'.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
return res.image('graph.png');
}).then((writtenImagePath) => {
console.log('Image written to ' + writtenImagePath);
});
Dependency-cruiser is a tool to analyze and visualize dependencies in JavaScript and TypeScript projects. It offers similar functionalities to Madge, such as detecting circular dependencies and generating dependency graphs. However, it provides more customization options and supports more file types.
Webpack-bundle-analyzer is a plugin and CLI utility that represents the size of webpack output files with an interactive zoomable treemap. While it focuses more on bundle size analysis rather than dependency graphs, it provides insights into the structure of your project and helps optimize bundle sizes.
Plato is a JavaScript source code visualization, static analysis, and complexity tool. It generates visual reports of your codebase, including dependency graphs. Compared to Madge, Plato offers a broader range of static analysis features but may not be as focused on dependency graph generation.
Create graphs from your CommonJS or AMD module dependencies. Could also be useful for finding circular dependencies in your code. Tested on Node.js and RequireJS projects. Dependencies are calculated using static code analysis. CommonJS dependencies are found using James Halliday's detective and for AMD I'm using some parts copied from James Burke's RequireJS (both are using UglifyJS). Modules written in CoffeeScript with extension .coffee are supported and will automatically be compiled on-the-fly.
Here's a very simple example of a generated image.
Here's an example generated from the Express project.
And some terminal usage.
To install as a library:
$ npm install madge
To install the command-line tool:
$ sudo npm -g install madge
Only required if you want to generate the visual graphs using Graphviz.
$ sudo port install graphviz
$ sudo apt-get install graphviz
var madge = require('madge');
var dependencyObject = madge('./');
console.log(dependencyObject.tree);
{Object|Array|String} src (required)
{Object} opts (optional)
Options object passed used in the constructor.
Dependency tree object. Can be overwritten with an object in the format:
{
'module1': ['dep1a', 'dep1b'],
'module2': ['dep2a']
}
Alias to the tree property.
Returns all the modules with circular dependencies.
Returns a list of modules that depends on a given module.
Get a DOT representation of the module dependency graph.
Get an image representation of the module dependency graph.
Usage: madge [options] <file|dir ...>
Options:
-h, --help output usage information
-V, --version output the version number
-f, --format <name> format to parse (amd/cjs)
-o, --output <type> output format (plain/json)
-s, --summary show summary of all dependencies
-c, --circular show circular dependencies
-d, --depends <id> show modules that depends on the given id
-x, --exclude <regex> a regular expression for excluding modules
-t, --dot output graph in the DOT language
-i, --image <filename> write graph to file as a PNG image
-l, --layout <name> layout engine to use for image graph (dot/neato/fdp/sfdp/twopi/circo)
-b, --break-on-error break on parse errors & missing modules
-n, --no-colors skip colors in output and images
-r, --read skip scanning folders and read JSON from stdin
-C, --config <filename> provide a config file
-O, --optimized if given file is optimized with r.js
$ madge /path/src
$ madge --format amd /path/src
$ madge --circular /path/src
$ madge --depends 'wheels' /path/src
$ madge --exclude '^foo$|^bar$|^tests' /path/src
$ madge --image graph.png /path/src
$ madge --dot /path/src > graph.gv
$ cat << EOF | madge --read --image example.png
{
"a": ["b", "c", "d"],
"b": ["c"],
"c": [],
"d": ["a"]
}
EOF
{
"format": "amd",
"image": "dependencyMap.png",
"fontFace": "Arial",
"fontSize": "14px",
"imageColors": {
"noDependencies" : "#0000ff",
"dependencies" : "#00ff00",
"circular" : "#bada55",
"edge" : "#666666",
"bgcolor": "#ffffff"
}
}
Try running madge with a different layout, here's a list of the ones you can try:
dot "hierarchical" or layered drawings of directed graphs. This is the default tool to use if edges have directionality.
neato "spring model'' layouts. This is the default tool to use if the graph is not too large (about 100 nodes) and you don't know anything else about it. Neato attempts to minimize a global energy function, which is equivalent to statistical multi-dimensional scaling.
fdp "spring model'' layouts similar to those of neato, but does this by reducing forces rather than working with energy.
sfdp multiscale version of fdp for the layout of large graphs.
twopi radial layouts, after Graham Wills 97. Nodes are placed on concentric circles depending their distance from a given root node.
circo circular layout, after Six and Tollis 99, Kauffman and Wiese 02. This is suitable for certain diagrams of multiple cyclic structures, such as certain telecommunications networks.
$ npm test
Added support for reading AMD dependencies from a r.js optimized file by using option -O.
Added missing fontsize option when generating images.
AMD plugins are now ignored as dependencies. Fixes issue.
Fixed Windows issue when reading from standard input with --read.
Switched library for walking directory tree which should solve issues on Windows.
Added proper exit code when running "madge --circular" so it can be used in build scripts.
Relative AMD module identifiers (if the first term is "." or "..") are now resolved.
Tweaked circular dependency path output.
Complete path in circular dependencies is now printed (and marked as red in image graphs).
Added support for CoffeeScript. Files with extension .coffee will automatically be compiled on-the-fly.
Fixed dependency issues with Node.js v0.8.
Added support for Node.js v0.8 and dropped support for lower versions.
Added ability to read config file and customize colors.
Initial release.
(The MIT License)
Copyright (c) 2012 Patrik Henningsson <patrik.henningsson@gmail.com>
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Create graphs from module dependencies.
The npm package madge receives a total of 465,683 weekly downloads. As such, madge popularity was classified as popular.
We found that madge demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.