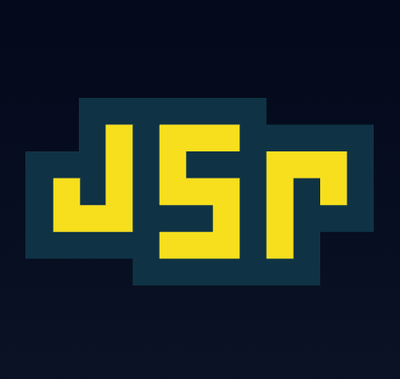
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Madge is a JavaScript library that helps you visualize and analyze the dependency graph of your project. It can be used to identify circular dependencies, generate dependency graphs, and more.
Generate Dependency Graph
This feature allows you to generate a dependency graph of your project. The code sample demonstrates how to use Madge to analyze the dependencies in a given project directory and output the result as an object.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
console.log(res.obj());
});
Identify Circular Dependencies
Madge can identify circular dependencies in your project. The code sample shows how to use Madge to find and log any circular dependencies in the specified project directory.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
console.log(res.circular());
});
Generate Graphviz DOT File
Madge can generate a Graphviz DOT file for visualizing the dependency graph. The code sample demonstrates how to generate and log the DOT representation of the dependency graph.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
return res.dot();
}).then((output) => {
console.log(output);
});
Create an Image of the Dependency Graph
Madge can create an image file of the dependency graph. The code sample shows how to generate an image of the dependency graph and save it as 'graph.png'.
const madge = require('madge');
madge('path/to/your/project').then((res) => {
return res.image('graph.png');
}).then((writtenImagePath) => {
console.log('Image written to ' + writtenImagePath);
});
Dependency-cruiser is a tool to analyze and visualize dependencies in JavaScript and TypeScript projects. It offers similar functionalities to Madge, such as detecting circular dependencies and generating dependency graphs. However, it provides more customization options and supports more file types.
Webpack-bundle-analyzer is a plugin and CLI utility that represents the size of webpack output files with an interactive zoomable treemap. While it focuses more on bundle size analysis rather than dependency graphs, it provides insights into the structure of your project and helps optimize bundle sizes.
Plato is a JavaScript source code visualization, static analysis, and complexity tool. It generates visual reports of your codebase, including dependency graphs. Compared to Madge, Plato offers a broader range of static analysis features but may not be as focused on dependency graph generation.
Madge is a developer tool for generating a visual graph of your module dependencies, finding circular dependencies, and give you other useful info. Joel Kemp's awesome dependency-tree is used for extracting the dependency tree.
Read the changelog for latest changes.
Graph generated from madge's own code and dependencies.
A graph with circular dependencies. Blue has dependencies, green has no dependencies, and red has circular dependencies.
$ npm -g install madge
Only required if you want to generate the visual graphs using Graphviz.
$ brew install graphviz || port install graphviz
$ apt-get install graphviz
path
is a single file or directory, or an array of files/directories to read. A predefined tree can also be passed in as an object.
config
is optional and should be the configuration to use.
Returns a
Promise
resolved with the Madge instance object.
Returns an
Object
with all dependencies.
const madge = require('madge');
madge('path/to/app.js').then((res) => {
console.log(res.obj());
});
Returns an
Array
with all modules that has circular dependencies.
const madge = require('madge');
madge('path/to/app.js').then((res) => {
console.log(res.circular());
});
Returns an
Array
with all modules that depends on a given module.
const madge = require('madge');
madge('path/to/app.js').then((res) => {
console.log(res.depends());
});
Returns a
Promise
resolved with a DOT representation of the module dependency graph.
const madge = require('madge');
madge('path/to/app.js')
.then((res) => res.dot())
.then((output) => {
console.log(output);
});
Write the graph as an image to the given image path. The image format to use is determined from the file extension. Returns a
Promise
resolved with a full path to the written image.
const madge = require('madge');
madge('path/to/app.js')
.then((res) => res.image('path/to/image.svg'))
.then((writtenImagePath) => {
console.log('Image written to ' + writtenImagePath);
});
});
Property | Type | Default | Description |
---|---|---|---|
baseDir | String | null | Base directory to use instead of the default |
includeNpm | Boolean | false | If node_modules should be included |
fileExtensions | Array | ['js'] | Valid file extensions used to find files in directories |
showFileExtension | Boolean | false | If file extension should be included in module name |
excludeRegExp | Array | false | An array of RegExp for excluding modules |
requireConfig | String | null | RequireJS config for resolving aliased modules |
webpackConfig | String | null | Webpack config for resolving aliased modules |
layout | String | dot | Layout to use in the graph |
fontName | String | Arial | Font name to use in the graph |
fontSize | String | 14px | Font size to use in the graph |
backgroundColor | String | #000000 | Background color for the graph |
nodeColor | String | #c6c5fe | Default node color to use in the graph |
noDependencyColor | String | #cfffac | Color to use for nodes with no dependencies |
cyclicNodeColor | String | #ff6c60 | Color to use for circular dependencies |
edgeColor | String | #757575 | Edge color to use in the graph |
graphVizOptions | Object | false | Custom GraphViz options |
graphVizPath | String | null | Custom GraphViz path |
Note that when running the CLI it's possible to use a runtime configuration file. The config should placed in
.madgerc
in your project or home folder. Look here for alternative locations for the file. Here's an example:
{
"showFileExtension": true,
"fontSize": "10px",
"graphVizOptions": {
"G": {
"rankdir": "LR"
}
}
}
List dependencies from a single file
$ madge path/src/app.js
List dependencies from multiple files
$ madge path/src/foo.js path/src/bar.js
List dependencies from all *.js files found in a directory
$ madge path/src
List dependencies from multiple directories
$ madge path/src/foo path/src/bar
List dependencies from all *.js and *.jsx files found in a directory
$ madge --extensions js,jsx path/src
Finding circular dependencies
$ madge --circular path/src/app.js
Show modules that depends on a given module
$ madge --depends 'wheels' path/src/app.js
Excluding modules
$ madge --exclude '^(foo|bar)$' path/src/app.js
Save graph as a SVG image (graphviz required)
$ madge --image graph.svg path/src/app.js
Save graph as a DOT file for further processing (graphviz required)
$ madge --dot path/src/app.js > graph.gv
Using pipe to transform tree (this example will uppercase all paths)
$ madge --json path/src/app.js | tr '[a-z]' '[A-Z]' | madge --stdin
To enable debugging output if you encounter problems, run madge with the
--debug
option then throw the result in a gist when creating issues on GitHub.
$ madge --debug path/src/app.js
$ npm test
Ensure you have Graphviz installed. And if you're running Windows graphviz is not setting PATH variable during install. You should add folder of gvpr.exe (typically %Graphviz_folder%/bin) to PATH variable.
Try running madge with a different layout, here's a list of the ones you can try:
dot "hierarchical" or layered drawings of directed graphs. This is the default tool to use if edges have directionality.
neato "spring model'' layouts. This is the default tool to use if the graph is not too large (about 100 nodes) and you don't know anything else about it. Neato attempts to minimize a global energy function, which is equivalent to statistical multi-dimensional scaling.
fdp "spring model'' layouts similar to those of neato, but does this by reducing forces rather than working with energy.
sfdp multiscale version of fdp for the layout of large graphs.
twopi radial layouts, after Graham Wills 97. Nodes are placed on concentric circles depending their distance from a given root node.
circo circular layout, after Six and Tollis 99, Kauffman and Wiese 02. This is suitable for certain diagrams of multiple cyclic structures, such as certain telecommunications networks.
MIT License
FAQs
Create graphs from module dependencies.
The npm package madge receives a total of 465,683 weekly downloads. As such, madge popularity was classified as popular.
We found that madge demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.