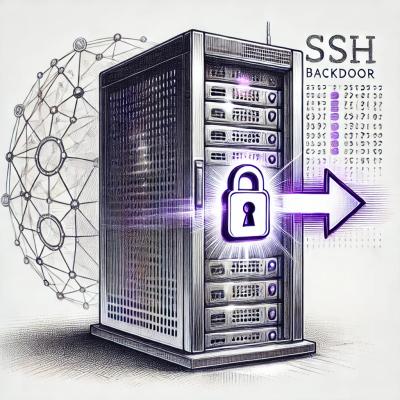
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
A simple but potentially magical GraphQL client for React backed by [react-query](https://github.com/tannerlinsley/react-query). The API is very similar to Apollo Client. The cache is managed by react-query and a very thin fetch wrapper is used to make th
A simple but potentially magical GraphQL client for React backed by react-query. The API is very similar to Apollo Client. The cache is managed by react-query and a very thin fetch wrapper is used to make the requests. A simple middleware API is used to allow for things like auth.
Usage example:
import {
MagiqlProvider,
createClient,
useQuery,
// no cost function, just returns the string,
// but allows IDE's to recognize the string as GraphQL tag.
gql,
} from "magiql";
const client = createClient("https://graphql-pokemon.now.sh");
const SearchPokemon = () => {
const { data, status, error } = useQuery(gql`
query pokemon($name: String) {
pokemon(name: $name) {
id
number
name
attacks {
special {
name
type
damage
}
}
image
}
}
`, {
variables: {
name: "pikachu",
},
});
return <pre>{JSON.stringify({ status, data, error }, null, 2)}</pre>;
};
const App = () => {
return (
<MagiqlProvider client={client}>
<SearchPokemon />
</MagiqlProvider>
);
}
We can use middleware to customize options passed down to the fetch function. This allows adding functionality for things like auth.
import { parseCookies } from "nookies";
import { createClient } from "magiql";
const getCookieToken = () => {
return parseCookies().token;
};
export const authMiddleware = (getToken: () => string) => (fetch) => {
return (url, operation, vars, options = {} as any) => {
const token = getToken();
options.headers = {
...options.headers,
authorization: token ? `Bearer ${token}` : "",
};
const a = fetch(url, operation, vars, options);
return a;
};
};
const client = createClient("https://graphql-pokemon.now.sh", {}, [authMiddleware(getCookieToken)]);
Inspired by babel-blade, an experimental API to infer the GraphQL query from usage within the components.
With babel config (example with Next.js):
{
"presets": ["next/babel"],
"plugins": ["magiql/babel"]
}
Code example:
import { useMagiqlQuery } from "magiql";
const MagicalPokemonSearch = () => {
const { query, loading, error } = useMagiqlQuery("searchPokemon");
if (loading) {
return <div>loading...</div>;
}
const pokemons = query.pokemons({
first: 10,
})
?.map((pokemon) => ({ image: pokemon?.image, id: pokemon?.id, name: pokemon?.name }));
return (
<pre>
{JSON.stringify({ loading, data: query, error, pokemons }, null, 2)}
</pre>
);
};
# Query generated by magiql at build-time:
`query searchPokemon {
pokemons_35d4: pokemons(first: 10) {
image
id
name
}
}`
Uses graphql-code-generator to generate hooks from graphql documents
across your project. Uses graphql-config spec to get the necessary config.
Converts magiql into a smart module that supplies necessary hooks with full type-safety during the developent. Just need to run magiql
command on the CLI to generate types. magiql --watch
runs it in watch mode.
# .graphqlconfig.yml
schema: https://graphql-pokemon.now.sh
documents: pages/**/*.{tsx,ts,graphql}
# pokemon.graphql
query searchPokemon($name: String) {
pokemon(name: $name) {
id
number
name
attacks {
special {
name
type
damage
}
}
image
}
}
`;
import { useSearchPokemonQuery } from "magiql";
const SearchPokemon = () => {
const { data, status, error } = useSearchPokemonQuery({
variables: {
name: "pikachu",
},
});
return <pre>{JSON.stringify({ status, data, error }, null, 2)}</pre>;
};
Using code generation, we can get autocompletion for types while working with useMagiqlQuery
.
FAQs
Most of the features that we are providing are thanks to [`react-query`](https://github.com/tannerlinsley/react-query). Using inspiration from `relay` and `urql`, we are able to provide a bunch of additional awesome GraphQL-specific features that you can
The npm package magiql receives a total of 22 weekly downloads. As such, magiql popularity was classified as not popular.
We found that magiql demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.