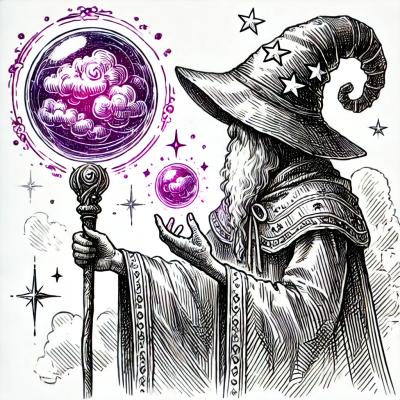
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Provides useful tools for writing command line scripts. It also ensures that a block of code is only invoked when called directly (as in calling node script
). It will not call the block of code if the script has been required in another module (as in require('script')
).
Bare bones example:
#!/usr/bin/env node
// won't be called if this module is required from another module!
require('main')(module).run(function(argv, scriptTools) {
scriptTools.exit('running this as a script!');
});
npm install --save main
require('main')(module)
Once required, you can chain the functions below.
Note that the module
part is required. This is to properly allow nesting of modules. e.g.
script1
contains require('main')(module)
script2
requires script1
, and implements it's own require('main')(module)
.We expect that when running script2
from the command line that it will only call the main function of script2
, and not call the main function of script1
.
For the time being, this is required until I or someone else figures out a way around it. Read the caching section of the Node Modules API for more information.
.usage(message)
An optional message to append to the top of flags that can describe how the script should be invoked, e.g.
Usage: ./script [flags] <posArg1> <posArg2>
Flags do not have to be specified in this string. Usage for flags are automatically generated based on the options provided (see below) and will appear after this usage message.
.flags(options)
options
follows the optimist format for options, but groups them together, e.g.:
require('main')(module).flags({
f: { alias: 'flag' },
t: { alias: 'secondFlag' },
d: { demand: true },
// ...
})
fn
is the callback that will be invoked when the script is ran directly from a terminal. It can take the following parameters:
fn(argv, scriptTools)
argv
is the parsed optimist argv objectscriptTools
is a helper that provides tools for working with the command line. See below for it's usage.When invoking .run()
, you will have access to the argv and the script tools in it's callback function. These tools allow you to perform short hand for repetitive tasks that come up when working with command line scripts.
In the documentation below, the $
symbol stands for the script tool helper function.
$.help
A variable holding the help message that was generated from the usage & any flags provided.
$.exit([exitCode], [printThis])
Exits the running program with the specified exit code and an optional output (printThis
can be an object, string, error, etc). It will write to stdout if the exitCode is 0 (default), or stderr if the exitCode != 0.
Some examples:
$.exit(); // exits with code 0
$.exit('done!'); // exits with code 0, prints hello to the console (stdout)
$.exit(0, 'done!'); // same as above
$.exit(0, 1); // exits with code 0, and prints "1" to the window (stdout)
$.exit(1); // exit's with code 1
$.exit(127, 'command not found!'); // exits with code 127 (stderr)
$.exit(1, new Error('foo')); // exit 1, prints the errors message (stderr)
$.readIn(callback)
Read from stdin in it's entirety, and return a string once finished. Do not use this if you are dealing with large amounts of data from stdin.
// read from stdin, write to stdout
$.readIn(function(input) { $.out(input); });
$.out(something)
and $.cout(something)
Write something to standard output. $.out
using process.stdout.write
and cout
uses console.log
.
$.err(something)
and $.cerr(something)
Write something to standard output. $.err
using process.stderr.write
and $.cerr
uses console.error
.
To view more examples visit the examples
directory.
Refer to the following script as basic.js
#!/usr/bin/env node
exports.sentence = function(name, word1, word2) {
return name + ', ' + word1 + ' ' + word2 + '.';
};
require('../index')(module)
.usage('Usage:\n node test.js [flags] <word1> <word2>')
.flags({
n: { alias: 'name', demand: true }
})
.run(function(argv, $) {
// exit if there aren't two words (positional arguments)
if (argv._.length !== 2) { $.exit(1, $.help); }
var word1 = argv._[0],
word2 = argv._[1];
var sentence = exports.sentence(argv.name, word1, word2);
$.exit(sentence); // prints the sentence, exits 0
});
Using the module from another script will not execute the code in main:
var basic = require('./basic');
console.log(basic.sentence('Nolan', 'sit', 'down'));
Running from the terminal ($? indicates exit status):
> node basic.js --name Nolan sit down
Nolan, sit down.
> $?
0 (success)
> node basic.js
# (prints out help & usage information. name / words are not defined)
> $?
1 (failure)
When installing, make sure to use the --save
option and/or specify the version in your package.json
dependencies. This package is undergoing some heavy changes at the moment and new versions may be radically different from previous releases. This type of business will stop once it reaches 0.1.0.
FAQs
main entry point
The npm package main receives a total of 2,651 weekly downloads. As such, main popularity was classified as popular.
We found that main demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.