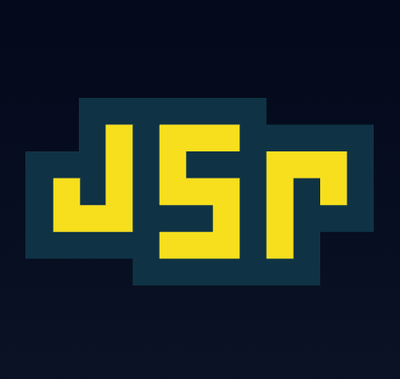
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
mappersmith
Advanced tools
Mappersmith is a lightweight HTTP client library for JavaScript that simplifies the process of making HTTP requests. It provides a declarative way to define API endpoints and offers features like middleware support, request/response transformation, and retries.
Declarative API Definition
Mappersmith allows you to define your API endpoints in a declarative manner. This example shows how to define a client with endpoints for fetching all users and fetching a user by ID.
const { forge } = require('mappersmith');
const api = forge({
clientId: 'my-api',
host: 'https://api.example.com',
resources: {
User: {
all: { path: '/users' },
byId: { path: '/users/{id}' }
}
}
});
api.User.all().then(response => console.log(response.data));
Middleware Support
Mappersmith supports middleware, allowing you to intercept and modify requests and responses. This example demonstrates how to log requests and responses using middleware.
const { forge, configs } = require('mappersmith');
configs.middleware = [
(request) => {
console.log('Request:', request);
return request;
},
(response) => {
console.log('Response:', response);
return response;
}
];
const api = forge({
clientId: 'my-api',
host: 'https://api.example.com',
resources: {
User: {
all: { path: '/users' }
}
}
});
api.User.all().then(response => console.log(response.data));
Request/Response Transformation
Mappersmith allows you to transform requests and responses. This example shows how to transform the response to extract user names from the list of users.
const { forge } = require('mappersmith');
const api = forge({
clientId: 'my-api',
host: 'https://api.example.com',
resources: {
User: {
all: { path: '/users', transform: (response) => response.data.map(user => user.name) }
}
}
});
api.User.all().then(userNames => console.log(userNames));
Retries
Mappersmith provides built-in support for retrying failed requests. This example configures the client to retry failed requests up to 3 times with exponential backoff.
const { forge, configs } = require('mappersmith');
configs.retry = { retries: 3, factor: 2, minTimeout: 1000 };
const api = forge({
clientId: 'my-api',
host: 'https://api.example.com',
resources: {
User: {
all: { path: '/users' }
}
}
});
api.User.all().then(response => console.log(response.data));
Axios is a popular promise-based HTTP client for JavaScript. It offers a simple API for making HTTP requests and supports features like interceptors, request/response transformation, and automatic JSON parsing. Compared to Mappersmith, Axios is more widely used and has a larger community, but it does not provide a declarative way to define API endpoints.
Fetch is a built-in web API for making HTTP requests in modern browsers. It provides a low-level API for making requests and handling responses. While Fetch is more lightweight and has no external dependencies, it lacks the higher-level abstractions and features like middleware and retries that Mappersmith offers.
Superagent is a small, progressive HTTP request library for Node.js and browsers. It provides a flexible API for making HTTP requests and supports features like plugins, request/response transformation, and retries. Compared to Mappersmith, Superagent is more flexible but requires more manual setup for defining API endpoints.
Mappersmith is a lightweight, dependency-free, rest client mapper for javascript. It helps you map your API to use at the client, giving you all the flexibility you want to customize requests or write your own gateways.
npm install mappersmith
Download the tag/latest version from the build folder.
Install the dependencies
npm install
Build
npm run build
To create a client for your API, you will need to provide a simple manifest, which must have host
and resources
keys. Each resource has a name and a list of methods with its definitions, like:
var manifest = {
host: 'http://my.api.com',
resources: {
Book: {
all: {path: '/v1/books.json'},
byId: {path: '/v1/books/{id}.json'}
},
Photo: {
byCategory: {path: '/v1/photos/{category}/all.json'}
}
}
}
You can specify an HTTP method for every API call, but if you don't, GET
will be used. For instance, let's say you can save a photo:
...
Photo: {
save: {method: 'POST', path: '/v1/photos/{category}/save'}
}
...
With the manifest in your hands, you are able to forge your client:
var Client = Mappersmith.forge(manifest)
And then, use it as defined:
// without callbacks
Client.Book.byId({id: 3})
// with all callbacks
Client.Book.byId({id: 3}, function(data) {
// success callback
}).fail(function() {
// fail callback
}).complete(function() {
// complete callback, it will always be called
})
If your method doesn't require any parameter, you can just call it without them:
Client.Book.all() // http://my.api.com/v1/books.json
Every parameter that doesn't match a pattern ({parameter-name}
) in path
will be sent as part of the query string:
Client.Book.all({language: 'en'}) // http://my.api.com/v1/books.json?language=en
It is possible to configure default parameters for your resources, just use the key params
in the definition. It will replace params in the URL or include query strings, for example, imagine that our manifest has the method byYear in the resource Photo:
...
Photo: {
byYear: {
path: '/v1/photos/{year}.json',
params: {year: new Date().getFullYear(), category: 'cats'}
}
}
...
If we call it without any params and new Date().getFullYear()
is 2015, it will generate the following URL:
Client.Photo.byYear();
// http://my.api.com/v1/photos/2015.json?category=cats
And, of course, we can override the defaults:
Client.Photo.byYear({category: 'dogs'});
// http://my.api.com/v1/photos/2015.json?category=dogs
To send values in the request body (usually for POST or PUT methods) you will use the special parameter body
:
Client.Photo.save({category: 'family', body: {year: 2015, tags: ['party', 'family']}})
It will create a urlencoded version of the object (year=2015&tags[]=party&tags[]=family
). If the body
used
is not an object it will use the original value. If body
is not possible as a special parameter
for your API you can configure it with another value, just pass the new name as the third argument
of method forge:
var Client = Mappersmith.forge(manifest, Mappersmith.VanillaGateway, 'data')
...
Client.Photo.save({category: 'family', data: {year: 2015, tags: ['party', 'family']}})
You can specify functions to process returned data before they are passed to success callback:
...
Book: {
all: {
path: '/v1/books.json',
processor: function(data) {
return data.result;
}
}
}
...
If you find tiring having to map your API methods with hashes, you can use our incredible compact syntax:
...
Book: {
all: 'get:/v1/books.json', // The same as {method: 'GET', path: '/v1/books.json'}
byId: '/v1/books/{id}.json' // The default is GET, as always
},
Photo: {
// The same as {method: 'POST', path: '/v1/photos/{category}/save.json'}
save: 'post:/v1/photos/{category}/save'
}
...
A downside is that you can't use processor functions with compact syntax.
Mappersmith allows you to customize the transport layer. You can use the default Mappersmith.VanillaGateway
, the included Mappersmith.JQueryGateway
or write your own version.
var MyGateway = Mappersmith.createGateway({
get: function() {
// you will have:
// - this.url
// - this.params
// - this.body
// - this.opts
},
post: function() {
}
// and other HTTP methods
})
Just provide an object created with Mappersmith.createGateway
as the second argument of the method forge
:
var Client = Mappersmith.forge(manifest, Mappersmith.JQueryGateway)
You can pass options for the gateway implementation that you are using. For example, if we are using the Mappersmith.JQueryGateway
and want one of our methods to use jsonp
, we can call it like:
Client.Book.byId({id: 2}, function(data) {}, {jsonp: true})
The third argument is passed to the gateway as this.opts
and, of course, the accepted options vary by each implementation. The default gateway, Mappersmith.VanillaGateway
, accepts a configure
callback:
Client.Book.byId({id: 2}, function(data) {}, {
configure: function(request) {
// do whatever you want
}
})
Imagine that you are using Mappersmith.JQueryGateway
and all of your methods must be called with jsonp
or use a special header, it will be incredibly boring add those configurations every time. Global configurations allow you to configure gateway options and a processor that will be used for every method. Keep in mind that the processor configured in the resource will be prioritized instead to global, for example:
var manifest = {
host: 'http://my.api.com',
rules: [
{ // This is our global configuration
values: {
gateway: {jsonp: true},
processor: function(data) { return data.result }
}
}
],
resources: {
Book: {
all: {path: '/v1/books.json'},
byId: {path: '/v1/books/{id}.json'}
},
Photo: {
byCategory: {path: '/v1/photos/{category}/all.json'}
}
}
}
It is possible to add some configurations based on matches in the URLs, let's include a header for every book URL:
...
rules: [
{ // This is our global configuration
values: {
gateway: {jsonp: true},
processor: function(data) { return data.result }
}
},
{ // This will only be applied when the URL matches the regexp
match: /\/v1\/books/,
values: {headers: {'X-MY-HEADER': 'value'}}
}
]
...
Just keep in mind that the configurations and processors will be prioritized by their order, and the global configurations does not have a match
key.
The gateways listed here are available through the Mappersmith
namespace.
The default gateway - it uses plain XMLHttpRequest
. Accepts a configure
callback that allows you to change the request object before it is used.
_method
in the body and X-HTTP-Method-Override
header, both with requested method as value. (default false
)It uses $.ajax
and accepts an object that will be merged with defaults
. It doesn't include jquery, so you will need to include that in your page.
_method
in the body and X-HTTP-Method-Override
header, both with request method as value. (default false
)npm run build-test
)npm run build
npm run release
See LICENCE for more details.
FAQs
It is a lightweight rest client for node.js and the browser
The npm package mappersmith receives a total of 145,904 weekly downloads. As such, mappersmith popularity was classified as popular.
We found that mappersmith demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.